NULL Undeclared Error in C++
-
the
NULL
Keyword in C+ - Include Relevant Predefined Header
-
Define
NULL
as a Constant -
Use
0
Instead ofNULL
-
Use
nullptr
Instead ofNULL
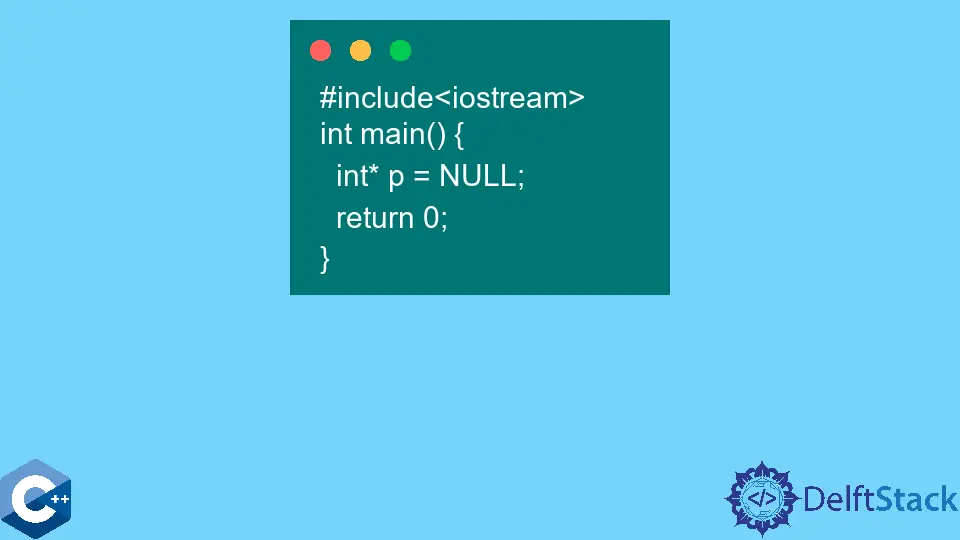
This article will discuss the NULL keyword and the error of NULL undeclared
in C++.
the NULL
Keyword in C+
The NULL
is constant in C++, which is used to initialize a pointer variable with a value of 0. We can use NULL
or 0
interchangeably.
It’s a good practice to assign a NULL
when you declare a pointer and don’t have an exact address to save in that pointer. So, it is called a null pointer until and unless it is pointed to some other value.
Syntax:
DataType *PointerName = NULL;
There are cases when you get the error when using the NULL
keyword like this:
int main() {
int* p = NULL;
return 0;
}
Output:
Now, let’s discuss how to solve this error.
Include Relevant Predefined Header
The NULL
keyword is declared a constant in different header files like iostream
, stdio
, or cstddef
. You can include any of these to resolve this error.
#include <iostream>
int main() {
int* p = NULL;
return 0;
}
The above code executes without an error because NULL
is also defined in iostream
.
Define NULL
as a Constant
You can define a constant named NULL
in your code.
#define NULL 0
int main() {
int* p = NULL;
return 0;
}
Use 0
Instead of NULL
Use 0
instead of NULL
. Both have the same meaning.
int main() {
int* p = NULL;
return 0;
}
Use nullptr
Instead of NULL
In the modern versions of C++ like C++ 11, nullptr
can be used as an alternative to the NULL
keyword.
int main() {
int* p = nullptr;
return 0;
}