How to Check the C++ Version
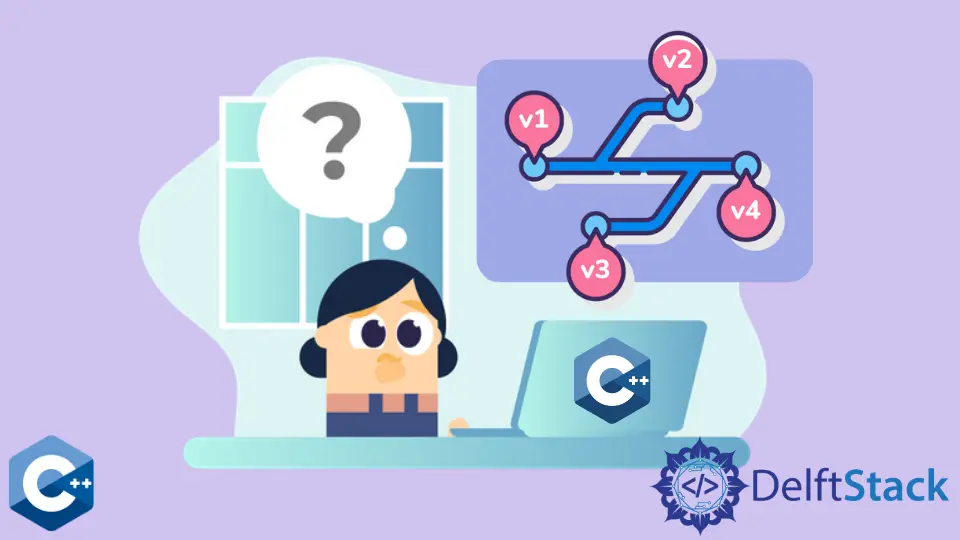
This article provides an overview of the importance of understanding the C++ version and explores various methods for checking it and the values of the __cplusplus
macro.
Versions of C++
Knowing the version of your C++ compiler is important for several reasons. Firstly, each version of C++ introduces new language features, enhancements, and improvements.
By knowing the version you’re working with, you can leverage these features to write more modern, efficient, and maintainable code. Secondly, different versions of C++ may have varying levels of compatibility with third-party libraries and frameworks.
Understanding your compiler version helps ensure compatibility with the tools and libraries you intend to use. Additionally, knowing the version allows you to communicate effectively with other developers, especially when collaborating on projects or seeking support in online communities.
Overall, being aware of your C++ version enables you to make informed decisions about language features, compatibility, and tooling, ultimately enhancing your development process.
As of my last update in January 2022, here are the major versions of the C++ language, along with their standardization years:
Version | Standard | Description | Value of __cplusplus |
---|---|---|---|
C++98 | ISO/IEC 14882:1998 |
The first standardized version of the C++ language, published in 1998. It introduced many core features of the language. | 199711L |
C++03 | ISO/IEC 14882:2003 |
Published in 2003, C++03 is a minor update to C++98, mainly correcting defects and ambiguities in the previous standard. | 199711L (Same as C++98) |
C++11 | ISO/IEC 14882:2011 |
Also known as C++0x during development, C++11 was a major update to the language, introducing significant new features such as lambda expressions, range-based for loops, and more. |
201103L |
C++14 | ISO/IEC 14882:2014 |
Published in 2014, C++14 is a minor update over C++11, mainly fixing issues and providing additional library features. It maintains full compatibility with C++11. | 201402L |
C++17 | ISO/IEC 14882:2017 |
C++17 introduced several new language features and library additions, including structured bindings, constexpr if , inline variables, and more. |
201703L |
C++20 | ISO/IEC 14882:2020 |
C++20 is a major update to the language, bringing significant new features such as concepts, ranges, coroutines, modules, and more. | 202002L |
C++23 (Expected) | ISO/IEC 14882:2023 |
C++23 is the upcoming version of the C++ standard. While the final standard has not been published as of the last update, it is expected to introduce further improvements and new features to the language. | Not Applicable |
This combined table provides information about both the C++ standard versions along with their associated __cplusplus
macro values, allowing developers to understand the relationship between the C++ language standards and the compiler’s predefined macros. These versions represent significant milestones in the evolution of the C++ language, with each iteration bringing new features, improvements, and enhancements to the language and its standard library.
Developers should be aware of these versions and their associated features to write modern, efficient, and maintainable C++ code.
Methods to Check the C++ Version
In C++, different compiler versions may support different language features and extensions. Therefore, it’s important for developers to know the version of the compiler being used, especially when writing code that relies on specific language features or compatibility.
Predefined Compiler Macros
One method to determine the version of the C++ compiler being used is by using predefined compiler macros. These macros, provided by the compiler, can be queried within your code to obtain information about the compiler version.
Predefined Compiler Macros: _cplusplus
Macro
Let’s explore how to check the C++ version using predefined compiler macros with a complete working code example:
#include <iostream>
int main() {
#ifdef __cplusplus
// Using __cplusplus macro to check C++ version
if (__cplusplus == 201703L)
std::cout << "C++17" << std::endl;
else if (__cplusplus == 201402L)
std::cout << "C++14" << std::endl;
else if (__cplusplus == 201103L)
std::cout << "C++11" << std::endl;
else if (__cplusplus == 199711L)
std::cout << "C++98" << std::endl;
else
std::cout << "Unknown C++ version" << std::endl;
#else
std::cout << "Not a C++ compiler" << std::endl;
#endif
return 0;
}
In this code snippet, we start by including the necessary header file <iostream>
for standard input-output operations.
Next, we define the main()
function, which serves as the entry point of our program.
Within the main()
function, we use an #ifdef
directive to check if the __cplusplus
macro is defined. The __cplusplus
macro is a standard predefined macro provided by C++ compilers, which holds the value corresponding to the version of the C++ standard being used.
Within the conditional block, a series of if
statements are employed to compare the value of the __cplusplus
macro with predefined constants representing different C++ standards. The code checks for various values, each corresponding to a specific version of the C++ standard.
For instance, if the value of __cplusplus
matches 201703L
, it indicates that the code is being compiled with a compiler supporting C++17. Similarly, values such as 201402L
, 201103L
, and 199711L
correspond to C++14, C++11, and C++98, respectively.
This approach allows developers to conditionally execute code based on the version of the C++ standard being used during compilation, enabling them to tailor their programs to the features and capabilities supported by the compiler.
If the value of __cplusplus
matches any of these constants, we print out the corresponding C++ version using std::cout
.
If the __cplusplus
macro is not defined, we print Not a C++ compiler
, indicating that the code is being compiled by a non-C++ compiler.
Finally, we return 0
to indicate successful program execution.
When you compile and run this code with a C++ compiler, it will output the version of C++ being used. For example:
This output confirms that the compiler being used supports C++17. By checking the C++ version at compile time, developers can ensure that their code remains compatible across different compilers and versions, and can make informed decisions about language features to use.
Predefined Compiler Macros: __GNUC__
and _MSC_VER
Let’s explore how to check the C++ version using predefined compiler macros with a complete working code example:
#include <iostream>
int main() {
#ifdef __GNUC__
std::cout << "GCC version: " << __GNUC__ << "." << __GNUC_MINOR__ << "."
<< __GNUC_PATCHLEVEL__ << std::endl;
#elif defined(_MSC_VER)
std::cout << "MSVC version: " << _MSC_VER << std::endl;
#else
std::cout << "Unknown compiler" << std::endl;
#endif
return 0;
}
In this code snippet, we start by including the necessary header file <iostream>
for standard input-output operations.
The main()
function is the entry point of our program. Inside it, we use preprocessor directives (#ifdef
, #elif
, #else
, #endif
) to conditionally compile different code blocks based on whether certain predefined macros are defined.
If the __GNUC__
macro is defined, it means the code is being compiled with GCC or a compiler compatible with GCC. In this case, we print out the version information obtained from the predefined macros __GNUC__
, __GNUC_MINOR__
, and __GNUC_PATCHLEVEL__
, which represent the major version, minor version, and patch level of GCC respectively.
If the _MSC_VER
macro is defined, it means the code is being compiled with Microsoft Visual C++. In this case, we print out the value of the _MSC_VER
macro, which represents the version of the Microsoft Visual C++ compiler being used.
If neither __GNUC__
nor _MSC_VER
is defined, it means the compiler being used is unknown or not recognized by the code. In this case, we print out the Unknown compiler
.
When you compile and run this code with different compilers, it will output the version information corresponding to the compiler being used. For example, with GCC, the output might be:
GCC version: major.minor.patchlevel
And with MSVC:
MSVC version: version_number
This output demonstrates the effectiveness of using predefined compiler macros to check the C++ version, providing valuable information about the compiler being used, which can be useful for compatibility testing and understanding the language features available.
Conclusion
Understanding the version of the C++ compiler being used is crucial for writing portable, compatible, and efficient code. Knowing the C++ version enables developers to leverage the latest language features, ensure compatibility with third-party libraries, and communicate effectively with other developers.
By utilizing these methods, developers can accurately determine the compiler version and adapt their code accordingly, ultimately enhancing the development process and the quality of C++ software.