How to Align Columns in printf Function in C
-
Use the
%{integer}d
Notation to Align the Output in C -
Use the
%*d
Notation to Align the Output in C -
Use the
%*d
and%{int}d
Notations to Align Columns in Table -
Use the
%0*d
Notation to Align the Output in C - Conclusion
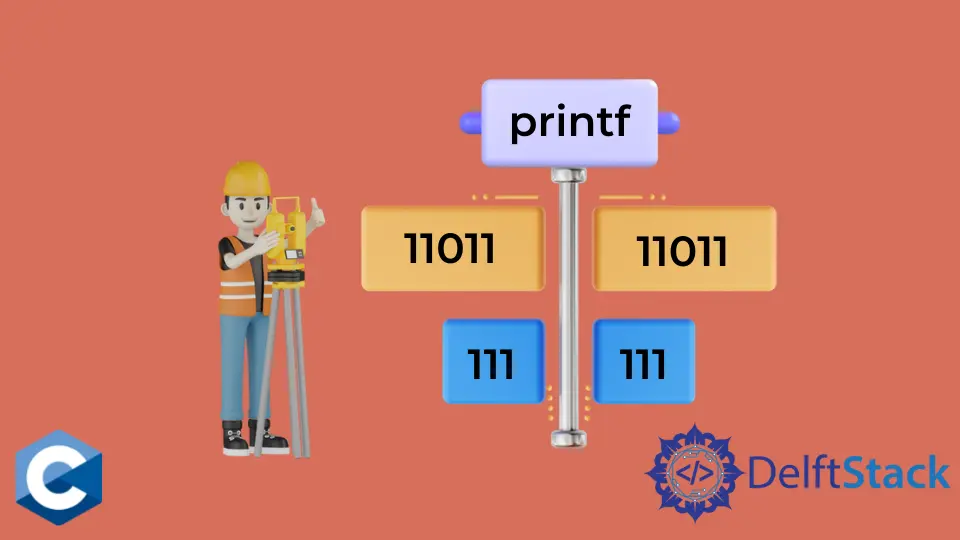
The printf
function in C is a powerful tool for formatting and displaying output. One of the key challenges in programming is presenting data in a visually organized and readable manner.
Achieving proper alignment of columns is important for enhancing the clarity and aesthetics of output. In this article, we will explore various methods to align columns effectively using the printf
function in C.
Use the %{integer}d
Notation to Align the Output in C
Before delving into column alignment techniques, let’s briefly review the essentials of the printf
function. This function is part of the standard I/O library and serves as a versatile tool for formatting output to the stdout
stream.
Notably, this stream employs buffering, introducing potential delays in output display, particularly when a newline character is absent within the string. To exert precise control over string formatting, printf
relies on format specifiers initiated by the %
character.
Among these specifiers, we find a particularly useful notation: %{integer}d
. By strategically placing an integer between the %
symbol and the format specifier character (d
for integers), we can dictate the number of spaces to be allocated on the left side of the output item.
When applied in the printf
function, it ensures that each entry is padded with the specified number of spaces, resulting in well-aligned columns. This simple yet powerful technique allows us to align numerical entries in a manner that enhances readability.
To illustrate this concept, consider the following example code snippet:
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char const *argv[]) {
int mm1 = 11011;
int mm2 = 111;
int mm3 = 11;
printf("%10d %d\n", mm1, mm1);
printf("%10d %d\n", mm2, mm2);
printf("%10d %d\n", mm3, mm3);
exit(EXIT_SUCCESS);
}
In this example, the %10d
notation is used to align the output columns. The integer 10
dictates the number of spaces allocated on the left side of each integer, ensuring a neatly aligned presentation.
The output of the provided code will look like this:
11011 11011
111 111
11 11
Use the %*d
Notation to Align the Output in C
In addition to the %{integer}d
notation, C provides another tool for aligning columns in the printf
function: the %*d
notation. This notation provides greater flexibility in formatting.
The %*d
notation enables us to specify the width of an integer dynamically by using an asterisk *
as a placeholder. The actual width value is then passed as an additional argument to printf
.
This feature is particularly useful when the column width needs to be calculated at runtime or when it varies for different elements within the same column.
Now, let’s explore the practical application of %*d
with a comprehensive code example.
#include <stdio.h>
int main() {
int column_width = 8;
printf("%*d %-*d\n", column_width, 11011, column_width, 11011);
printf("%*d %-*d\n", column_width, 111, column_width, 111);
printf("%*d %-*d\n", column_width, 11, column_width, 11);
return 0;
}
In this example, first, we set the column_width
variable to 8, determining the width for each column in our table.
Then, in each printf
statement, we use the %*d
format specifier to dynamically set the width of the first integer in the row based on the value stored in the column_width
variable. The second integer in each row is formatted with %-*d
, ensuring left alignment within a column of the specified width.
For example, in the first printf
statement, "%*d %-*d\n"
tells printf
to print two integers followed by a newline. The first %*d
dynamically adjusts the width of the first integer (11011
) using the column_width
, and the second %-*d
does the same for the second integer.
The subsequent printf
statements follow the same pattern, printing two more rows of integers with varying values.
Output:
11011 11011
111 111
11 11
Use the %*d
and %{int}d
Notations to Align Columns in Table
To achieve even more sophisticated column alignment in C, we can combine both %*d
and %{int}d
notations in the printf
function.
When combining %*d
and %{int}d
notations, we dynamically set the width of the columns using %*d
and simultaneously specify the precision for integer values with %{int}d
. This combination is useful when we want to align columns dynamically while ensuring a specific number of digits are displayed for integers.
Now, let’s dive into a complete code example to illustrate the application of these notations:
#include <stdio.h>
int main() {
int column_width = 8;
printf("%-*s | %-*s | %-*s\n", column_width, "Number", column_width,
"Description", column_width, "Value");
printf("%-*c | %-*c | %-*c\n", column_width, '-', column_width, '-',
column_width, '-');
printf("%-*d | %-*s | %*.*d\n", column_width, 123, column_width, "First",
column_width, 2, 4567);
printf("%-*d | %-*s | %*.*d\n", column_width, 456, column_width, "Second",
column_width, 4, 789);
printf("%-*d | %-*s | %*.*d\n", column_width, 7890, column_width, "Third",
column_width, 1, 12345);
return 0;
}
In this code, the %-*s
notation is used for dynamic width adjustment for strings, and the %-*d
notation is used for integers. The combination of %*.*d
allows us to dynamically set the width and precision for integers.
Here, the negative sign in %-*d
ensures left alignment for integers.
The program prints a table with three columns: Number
, Description
, and Value
. The width of each column is controlled dynamically using the specified column_width
, and the precision for integer values in the Value
column is also dynamically set.
Output:
Number | Description | Value
-------- | ----------- | -------
123 | First | 4567
456 | Second | 789
7890 | Third | 12345
Use the %0*d
Notation to Align the Output in C
In C programming, the %0*d
notation provides another way for aligning columns with fixed-width and zero padding. It helps ensure a consistent and visually appealing presentation of numeric data in tables.
The format specifier %d
represents an integer, and the 0
flag instructs the printf
function to pad the integer with zeros to meet the specified width. The *
is a placeholder that indicates the width will be passed as an additional argument to printf
.
Now, let’s see a code example demonstrating the application of %0*d
:
#include <stdio.h>
int main() {
int column_width = 5;
printf("%-*s | %-*s\n", column_width, "ID", column_width, "Count");
printf("%0*d | %0*d\n", column_width, 123, column_width, 7);
printf("%0*d | %0*d\n", column_width, 456, column_width, 23);
return 0;
}
In this C code snippet, we begin by defining a variable, column_width
, which sets the width for each column in our table, ensuring consistency and a visually organized presentation.
The first printf
statement prints the table header with the strings ID
and Count
, applying the %-*s
notation for string columns to dynamically set their width based on the column_width
value. The negative sign ensures left alignment.
Moving on to the data rows: the subsequent printf
statements use the %0*d
notation for integers, where the %d
represents the integer and the 0
flag instructs the printf
function to pad the integer with zeros.
The *
serves as a placeholder for the width, and the width itself is passed as an argument, in this case, column_width
. The result is fixed-width integers with zero padding.
The program then proceeds to print two data rows with integers, where ID
values 123
and 456
are displayed with a fixed width of 5 and zero padding. Similarly, the Count
values 7
and 23
follow the same formatting.
Output:
ID | Count
00123 | 00007
00456 | 00023
Conclusion
Aligning columns in the printf
function is a fundamental skill for any C programmer. Whether you are working with integers, floats, or a combination of data types, mastering these alignment techniques will significantly improve the readability and presentation of your program’s output.
Experiment with these methods, and choose the approach that best suits your specific formatting requirements.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook