How to Print Binary of Number in C
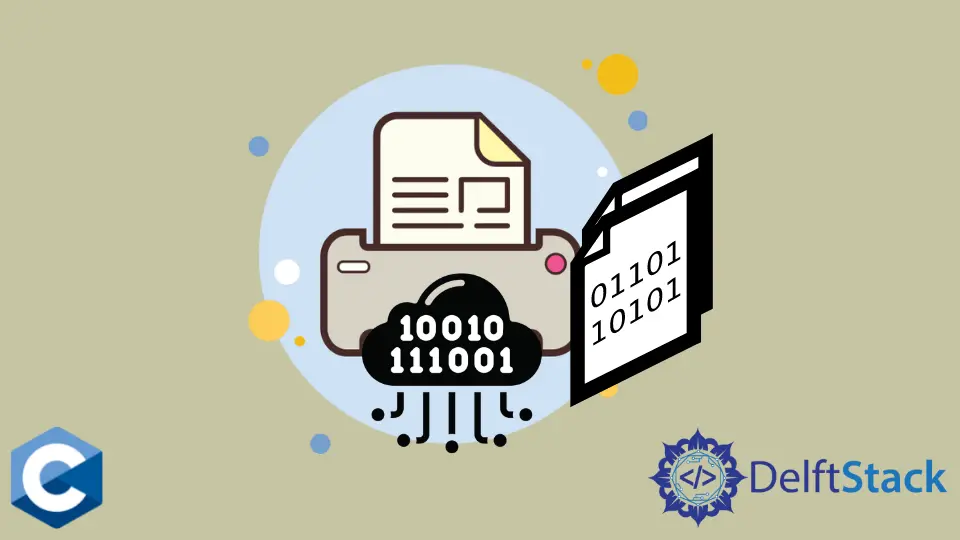
This trivial guide is about implementing decimal to binary number system converter using the C language. Before jumping into the implementation directly, we will first recap the binary number system and then discuss multiple C implementations to convert a decimal representation into a binary equivalent.
Binary Number System
Any system which operates on two discrete or categorical states is known as a binary system. Similarly, a binary number system represents numbers using only two symbols: 1
(one) and 0
(zero).
Therefore, it is also known as the base-2 system.
Currently, most transistor-based logic circuit implementations use discrete binary states. Therefore, all modern digital computers use binary systems to represent, store, and process data.
For example, converting 6
into a binary number system.
Here, 6
is the number from the decimal number system with base 10
, and its corresponding binary is 110
, which is in the binary number system having base 2
. Let’s look at the process of this conversion.
Process of Conversion
Step 1: Dividing 6 by 2 to get the answer. You get the dividend for the next step, using the integer quotient achieved in this stage.
Continue in this manner until the quotient reaches zero.
Dividend | Remainder |
---|---|
6/2 = 3 | 0 |
3/2 = 1 | 1 |
1/2 = 0 | 1 |
Step 2: The binary can be formed by collecting all the remainders in reverse chronological order (from bottom to top).
With 1
being the most significant bit (MSB) and 0
being the least significant bit (LSB). Hence, the binary of 6
is 110
.
C Implementations for Conversion
There can be multiple ways in the C language to convert a number into a binary number system. It can be an iterative solution or a recursive one.
It is up to your choice of programming. This article will discuss a recursive solution because it is very straightforward.
Solution 1:
If number > 1
:
- place
number
on the stack - call function with
number/2
recursively - Take a
number
from the stack, divide it by two, and output the remainder.
#include <stdio.h>
void convertToBinary(unsigned a) {
/* step 1 */
if (a > 1) convertToBinary(a / 2);
/* step 2 */
printf("%d", a % 2);
}
int main() {
// Write C code here
printf("Binary of the number is: ");
convertToBinary(6);
printf("\n");
return 0;
}
This code snippet releases the following output.
Binary of the number is: 110
Solution 2:
- Check if
number > 0
- Apply the right shift operator by 1 bit and then call the function recursively.
- Output the bits of the
number
#include <stdio.h>
void convertToBinary(unsigned n) {
if (n > 1) convertToBinary(n >> 1);
printf("%d", n & 1);
}
int main() {
// Write C code here
printf("Binary of the number is: ");
convertToBinary(8);
printf("\n");
return 0;
}
The output of the given code snippet is:
Binary of the number is: 1000
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn