MIN and MAX Function in C
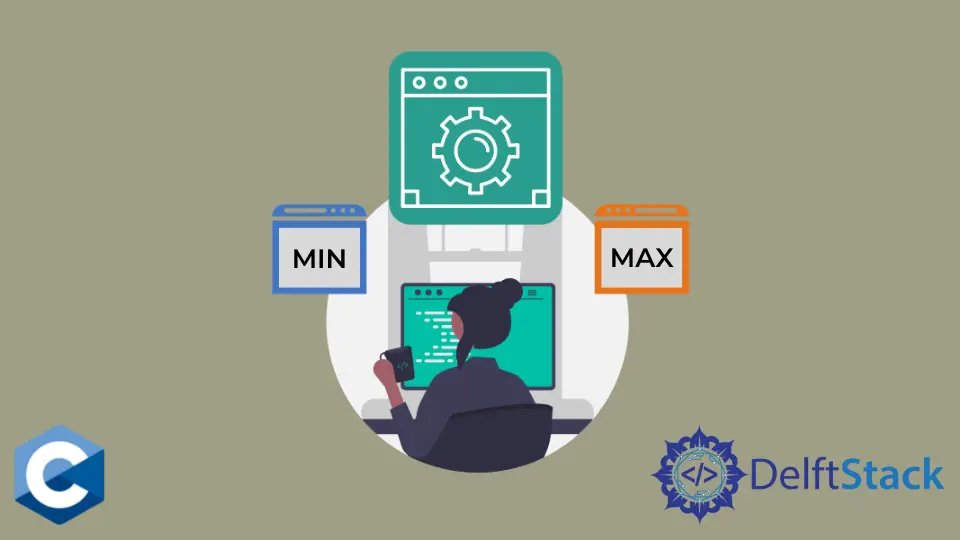
This tutorial will discuss using macros or loops to implement the MIN
and MAX
functions in C.
the MIN
and MAX
Function in C
The MIN
and MAX
functions are used to find the minimum and maximum number from two values and are not predefined in C language. If we want to use the MIN
and MAX
functions, we must define them in C.
We can use macros to define the MIN
and MAX
functions in the C language. A macro is a code segment used to define variable values and functions.
When we call a macro, it gets replaced by its value or function. For example, we can define the value of a variable like PI
, and when we use the name PI
in our code, the name will be replaced by its value.
We can also define a macro as a function that will be called if we write its name in the code. We use the #define
keyword to define macros in the C language.
For example, let’s define the MIN
and MAX
functions using C language macros. See the code below.
#include <stdio.h>
#define MIN(i, j) (((i) < (j)) ? (i) : (j))
#define MAX(i, j) (((i) > (j)) ? (i) : (j))
int main() {
printf("Min = %d\n", MIN(0, 10));
printf("Max = %d", MAX(0, 10));
return 0;
}
Output:
Min = 0
Max = 10
In the above code, the ?
symbol is the ternary operator and is used in place of the if-else
statement. If the condition before the ternary operator is true, the output will be equal to the left side of the colon :
, and if the condition is false, the output will equal the right side of the colon :
.
In the above code, we used brackets because the input can also be an expression like a+b
, and the output will change if we don’t use brackets. We can also use the if-else
statement instead of the ternary operator.
The above code will create problems in case of a double evaluation side effect in which we pass an expression inside a function. We can use the __typeof__
command to define the MIN
and MAX
functions to avoid the above problem, giving us more safety and fewer bugs.
The __typeof__
command refers to the type of an expression. We can use the typeof
command with expressions and a type.
For example, let’s define the above MIN
and MAX
functions using the __typeof__
command. See the code below.
#include <stdio.h>
#define MAX(x, y) \
({ \
typeof(x) _x = (x); \
typeof(y) _y = (y); \
_x > _y ? _x : _y; \
})
#define MIN(x, y) \
({ \
typeof(x) _x = (x); \
typeof(y) _y = (y); \
_x < _y ? _x : _y; \
})
int main() {
printf("Min = %d\n", MIN(0, 10));
printf("Max = %d", MAX(0, 10));
return 0;
}
Output:
Min = 0
Max = 10
In the above code, we used the __typeof__
command to refer to the type of the inputs and then we used the ternary operator to define the if-else
statement. We can see that the output is the same as in the previous example.
The above code can avoid the double evaluation problem because the above macro will evaluate the arguments only once. The above methods only work in the case of two integer inputs.
If we want to find the minimum and maximum number present in an array of numbers, we cannot use the above methods because, in the above methods, we are comparing only two numbers. In the case of an array, we have to compare all the elements together to find the minimum or maximum value present in the array.
We can store the first element of the array in a variable, and then we can use a loop to compare this element with other elements of the array.
In the case of the maximum number, if the next element is greater than the next element present in the array, it means the element which is saved is not the maximum element present in the array, and we will replace the saved element with the next element, and we will repeat this procedure until the saved element is compared with all the elements present in the array.
In case of a minimum number, we will replace the saved element with the next element only if the next element is less than the saved element, and we will repeat this procedure until the saved element is compared with all the elements present in the array.
#include <stdio.h>
int MAX(int My_array[], int len);
int MIN(int My_array[], int len);
int main() {
int My_array[] = {6, 3, 15, 9, 10, 50, 99, 11};
int len = sizeof(My_array) / sizeof(My_array[0]);
int numMax = MAX(My_array, len);
int numMin = MIN(My_array, len);
printf("Max number = %d\n", numMax);
printf("Min number = %d", numMin);
return 0;
}
int MAX(int My_array[], int len) {
int num = My_array[0];
for (int i = 1; i < len; i++) {
if (My_array[i] > num) {
num = My_array[i];
}
}
return num;
}
int MIN(int My_array[], int len) {
int num = My_array[0];
for (int i = 1; i < len; i++) {
if (My_array[i] < num) {
num = My_array[i];
}
}
return num;
}
Output:
Max number = 99
Min number = 3
In the above code, we created two functions, MAX()
and MIN()
, which have two input arguments. The first argument is the given array, and the second is the array’s length.
We used the sizeof()
function to find the size of the entire array and divided it by the size of the first element of the array to get the length of the given array.
Inside the MAX()
and MIN()
function, we stored the first element of the array in a variable, and then we compared it with all the other elements of the array using a loop that will stop when the integer i
becomes equal to the length of the array which means the loop has reached the end of the array.
Inside the loop, we used an if
statement to compare the next element of the array with the value that we saved, and we replaced its value if the next element is greater than the saved element in the case of MAX()
function, and in MIN()
function, we will replace the value if the next element is smaller than the saved element. We used the printf()
function to print the value returned by the MIN()
and MAX()
function.
The above code will only work for an array of an integer data type, but we can also change the above code to find the minimum and maximum numbers in the case of other data types like float
. In the above code, we have to change the int
data type to float
in case of an array of float
data types.
For example, the data type of the array will become float
, and the data type of the variable used to store the first element of the array will also become float
, and the data type of the length will remain the same.