How to Handling Errors in C
- Error Handling in C
-
Use Global Variable
errno
-
Use
perror()
andstrerror()
Functions -
Use
EXIT_STATUS
- Conclusion
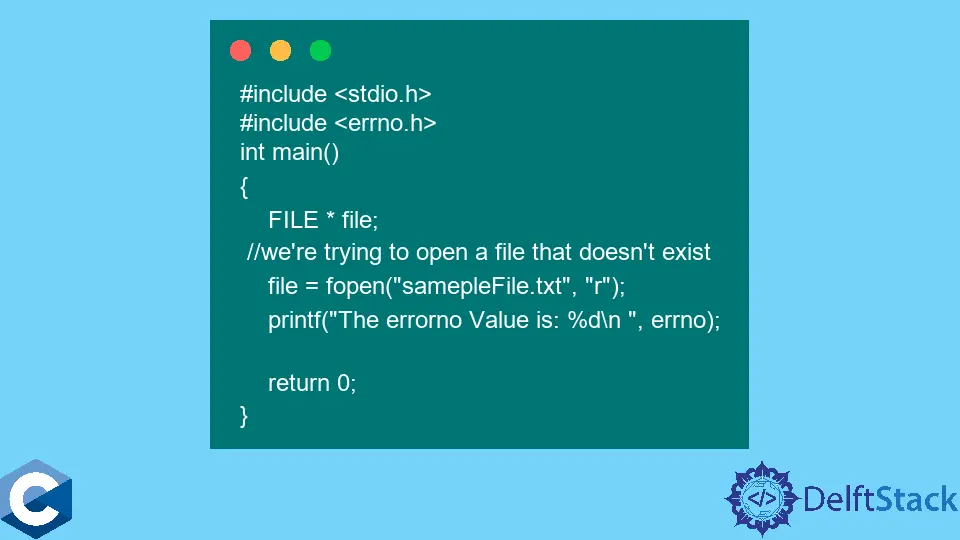
This brief article is about the discussion on different ways that can be used to handle Errors in C language programs.
Error Handling in C
Although error handling (or exception handling) is not directly supported by C, there are still ways to handle errors in the language. A programmer must test function return values and attempt to prevent errors from occurring in the first place.
A quick test on these return values can be done quickly. For example, an if
statement because many C function calls return a -1
or NULL
in case of an error.
Different methods can be used to handle different types of errors in C language. Some of them are discussed in this article.
- Use the global variable
errno
. - Using
perror()
andstrerror
() functions by C language. - Use the Exit status of the function.
Use Global Variable errno
A global variable errno
is defined in the library file errorno.h
. In the C programming language, a variable called errno
is automatically given a code (value) that can be used to specify the error that occurred.
Error types are indicated by different codes (values) for errno
. Here’s a list of a few different errno
values and their explanations.
Error Code | Description |
---|---|
1 | This operation is not allowed. |
2 | File or directory is not found. |
3 | Function not found. |
4 | Any system call interrupted. |
5 | Input or output error. |
6 | No such device found. |
7 | Arguments list passed is longer than specified. |
8 | Exec function error. |
9 | Bad file number error. |
10 | Chile process not found. |
11 | Try it again. |
12 | Memory shortage error. |
13 | Access permission is denied. |
Let’s consider an example code segment in which we will try to open a file that doesn’t exist. Then we will see the value of the errno
variable.
Code Example:
#include <errno.h>
#include <stdio.h>
int main() {
FILE* file;
// we're trying to open a file that doesn't exist
file = fopen("samepleFile.txt", "r");
printf("The errorno Value is: %d\n ", errno);
return 0;
}
In the code example above, we created an object for File
and then called the fopen()
function so that it could open a file for reading. But we have given a file name not found in the directory.
So it will create an error, and an errno
value will be set. We will then print its value.
Output:
From the output, the error number returned is 2
. It can be matched from the table above that 2
means "no such file or directory was found"
.
Use perror()
and strerror()
Functions
The error types encountered are indicated by the errno
value obtained above.
If the error description is necessary, two functions can be used to display a text message linked to the error number. These are perror()
and strerror()
.
-
The
perror()
function displays the user-defined text message for the error, followed by the actual message set for thaterrno
.Syntax:
void perror(const char *str)
The
str
is the string for the custom message that you need to display before the actual error message. -
The
strerror
returns the textual message set for theerrno
passed to it.Syntax:
char *strerror(int errnum)
The
errnum
is the error no that is passed to it.
We will modify the previous example for opening a non-existing file and display the error messages through perror()
and strerror()
.
Code Example:
#include <errno.h>
#include <stdio.h>
int main() {
FILE* file;
// we're trying to open a file that doesn't exist
file = fopen("samepleFile.txt", "r");
if (file == NULL) {
printf("Value of error number: %d\n", errno);
perror("Error message by perror");
printf("Error Message from strerror : %s\n", strerror(errno));
`
} else {
fclose(file);
}
return 0;
}
In the above code segment, we have printed the errno
value, then the error message using perror,
and then through strerror
.
Output:
Use EXIT_STATUS
To indicate a successful or unsuccessful termination, the C standard specifies two constant macros: EXIT SUCCESS
and EXIT FAILURE
, which may be passed to exit()
. These macros are those listed in stdlib.h
.
We will further modify the previous example and make the failure exit call if the file is not found and a successful exit in the case file is found.
Code Example:
#include <errno.h>
#include <stdio.h>
#include <stdlib.h>
int main() {
FILE* file;
// we're trying to open a file that doesn't exist
file = fopen("samepleFile.txt", "r");
if (file == NULL) {
int errorNumber = errno;
printf("Value of error number: %d\n", errno);
perror("Error message by perror");
printf("Error Message from strerror : %s\n", strerror(errno));
exit(EXIT_FAILURE);
} else {
fclose(file);
exit(EXIT_SUCCESS);
}
printf("Program exiting");
return 0;
}
In the code example above, we have included the exit function in both cases of the conditional statement. We have added a printf
function just before the program exits.
This message will not be printed as the program would be exited before.
Output:
Conclusion
C does not have explicit support for exception handling. However, we can use certain error-handling primitives (i.e., library functions) to achieve sufficient exception handling in C.