Event Emitter in Angular
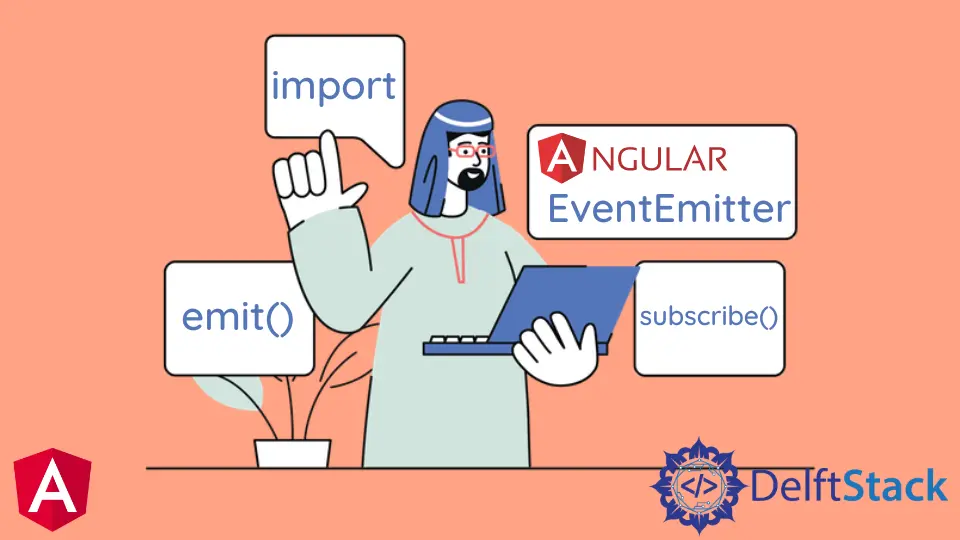
We will introduce EventEmitter
in angular and the proper use of EventEmitter
in angular. And we will also give an example of EventEmitter
in angular.
What Is EventEmitter in Angular
EventEmitter
is a module that helps share data between components using emit()
and subscribe()
methods. EventEmitter
is in the Observables layer, which observes changes and values and emits the data to the components subscribed to that EventEmitter
instance.
emit()
emit()
is an EventEmitter
method that emits an event containing a given value.
# angular
emit(value?: A): void
emit()
has only one parameter, value
.
subscribe()
subscribe()
is an EventEmitter
method that registers handlers for events emitted by this instance.
# angular
subscribe(next?: (value: A) => void, error?: (error: any) => void, complete?: () => void) : Subscription
subscribe()
have three optional parameters which can be used to pass values, errors, or completion notification in EventEmitter
.
- The
next
parameter is a custom handler for emitted events. - The
error
parameter is a custom handler for error notifications from this emitter. - The
complete
parameter is a custom handler for a completion notification from this emitter.
Use of EventEmitter in Angular
Now, we will use EventEmitter
as an example to understand it completely. In this example, we will change the component’s background color and font color and display its values in other components.
So, first of all, we will set up our app.module.ts
file and import modules and components.
# angular
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { AppComponent } from './app.component';
import { HelloComponent } from './hello.component';
import { BgChangeComponent } from './bg-change.component';
@NgModule({
imports: [BrowserModule, FormsModule],
declarations: [AppComponent, HelloComponent, BgChangeComponent],
bootstrap: [AppComponent],
})
export class AppModule {}
Now, We will set our html file for our app component and add the following code in the app.component.html
file
# angular
<emitted (touch)="respond()" name="{{ name }}" [color]="color"></emitted>
<p>{{ response }}</p>
<p>{{ color }}</p>
<bg-change (colorChange)="changeColor($event)"></bg-change>
Here we have used changeColor($event)
which is an EventEmitter
, and we are displaying values from EventEmitter
in response
and color
.
Now we will set the app.component.ts
file and set values for name
, response
, and color
variables displayed when the first time page is loaded.
We will define a function respond()
, which will change the value of the response
.
We will also define the changeColor()
function, which will set the value of color. So, our app.component.ts
file will look like this:
# angular
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
name = 'Angular 11';
response = 'Background not changed yet';
color = 'brown';
respond() {
this.response = 'Success';
}
changeColor(color) {
this.color = color;
}
}
Now we will edit our hello.component.ts
file and add the following code to it.
# angular
import {
Component,
Input,
ElementRef,
Renderer2,
Output,
EventEmitter,
OnInit,
OnChanges,
} from '@angular/core';
@Component({
selector: 'emitted',
template: `<h1
(click)="emit()"
>{{text}} {{name}}</h1>`,
})
export class HelloComponent implements OnInit, OnChanges {
@Input() name: string;
@Input() color = 'brown';
text = 'It is';
@Output()
touch = new EventEmitter<string>();
constructor(private renderer: Renderer2, private el: ElementRef) {}
ngOnInit() {
this.renderer.setStyle(this.el.nativeElement, 'color', this.color);
}
ngOnChanges() {
this.renderer.setStyle(this.el.nativeElement, 'color', this.color);
}
emit() {
this.touch.emit('touched');
}
}
In the above component, @component
will get the emitted
tag from the app.component.html
file and set a color on initializing. When the button is clicked, it will change the color. We are also passing text
which is displayed on load.
Now we will add a new bg-change.component.ts
file. and add the following code in it.
# angular
import {
Component,
ViewChild,
ElementRef,
Renderer2,
EventEmitter,
Output,
} from '@angular/core';
@Component({
selector: 'bg-change',
template: `<button
(click)="Bgchange()"
>{{content}}</button>`,
styles: [`button { padding: 10px; }`],
})
export class BgChangeComponent {
body = this.el.nativeElement.ownerDocument.body;
activateColor = 'white';
@Output()
colorChange = new EventEmitter<string>();
content = 'Change Page Background';
constructor(private renderer: Renderer2, private el: ElementRef) {}
Bgchange() {
this.colorChange.emit(this.activateColor);
this.activateColor === 'white'
? (this.activateColor = 'red')
: (this.activateColor = 'white');
this.renderer.setStyle(this.body, 'background', this.activateColor);
}
}
So our output will look like below.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn