How to Use SetInterval in AngularJS
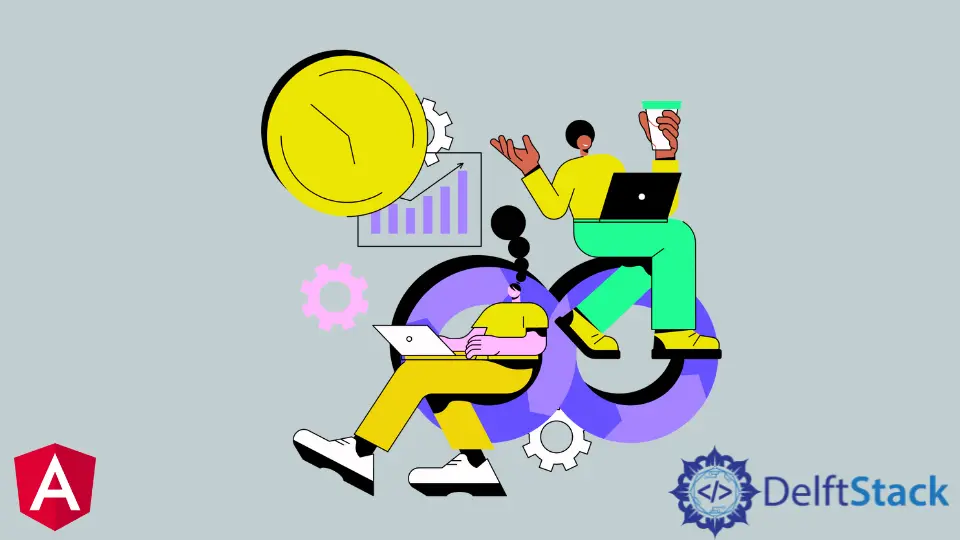
This article will introduce setInterval()
in AngularJS with examples.
the setInterval()
in AngularJS
While working on a big productive application in Angular, we need to set intervals for certain tasks. These tasks take care of some important data after some interval automatically.
For example, if we want to take a backup of the whole database, we can set the interval time to 24 hours so that it will be backed up after every 24 hours.
If we want to display the latest activity, posts, or notifications on our application, we can use the setInterval()
method in the AngularJS application. This method takes in two parameters.
The first parameter is the function that will be executed after each interval. The second parameter is the time in milliseconds.
The setInterval()
method is a function that specifies the interval in milliseconds. The setInterval()
method will keep on executing when the application is running.
There are only two methods to stop the setInterval()
method. We can close the application or use another method, clearInterval()
, to end the setInterval()
method.
The syntax of the setInterval()
method is shown below.
newInterval = setInterval(function(), milliseconds);
If we want to stop the execution, we must use the clearinterval()
method. The syntax of this method is shown below.
clearInterval(newInterval);
Let’s go through an example in which we will get the current time and use the setInterval()
method to update it every second. The code for this example is shown below.
<!DOCTYPE html>
<html>
<body>
<p id="timer"></p>
<script>
setInterval(newTimer, 1000);
function newTimer() {
const newDate = new Date();
document.getElementById("timer").innerHTML = newDate.toLocaleTimeString();
}
</script>
</body>
</html>
Output:
The above example shows that the time is updated after every second. Now, if we want to add an option to stop the time whenever we want, we can use the clearInterval()
method.
Let’s go through the same example and add a button to stop the time or the setInterval()
method with the help of the clearInterval()
method, as shown below.
<!DOCTYPE html>
<html>
<body>
<p id="timer"></p>
<button onclick="newStopFunc()">Stop Time By Clicking This Button</button>
<script>
const newInterval = setInterval(newTimer, 1000);
function newTimer() {
const newDate = new Date();
document.getElementById("timer").innerHTML = newDate.toLocaleTimeString();
}
function newStopFunc() {
clearInterval(newInterval);
}
</script>
</body>
</html>
Output:
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn