How to Upload Image in Angular
- Upload an Image in Angular
- Export Components and Declare Variables in Angular
- Basic Upload For Multiple Images in Angular
- Basic Upload For Single Image in Angular
- Upload and Progress For Multiple Images in Angular
- Upload and Progress For Single Image in Angular
- Calling the Upload Function in Angular
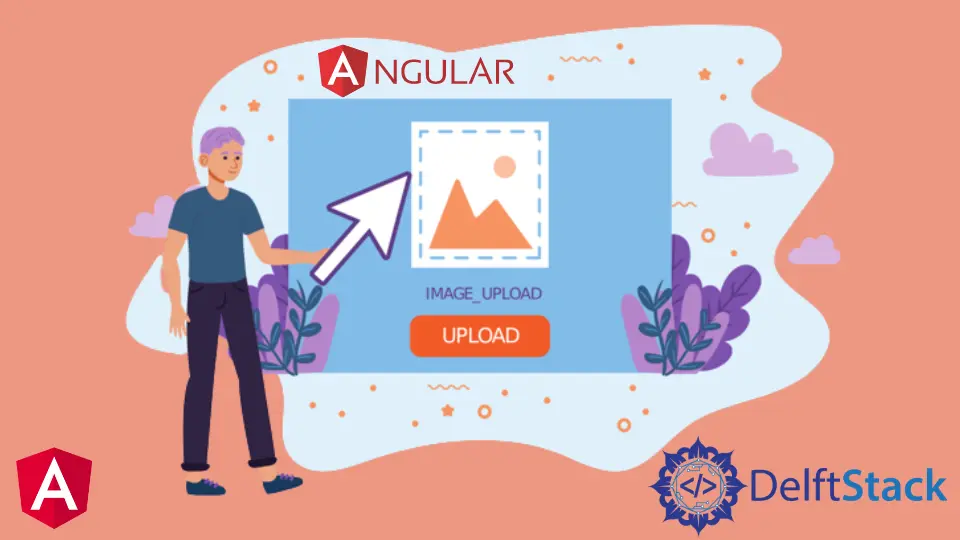
This article introduces the following:
- Upload an image in four different styles using Angular.
- Display the progress bar when the image is uploading.
- Display an image upload completion message when the upload is complete.
Upload an Image in Angular
Images are a basic part of any web application, and every website has images. In this tutorial, we will learn how to upload an image in Angular in different ways.
Let’s create a new application using the command below.
# angular
ng new my-app
After creating our new application in Angular, we will go to our application directory using this command.
# angular
cd my-app
Now, let’s run our app to check if all dependencies are installed correctly.
# angular
ng serve --open
We will import libraries and set components for the file uploader.
# angular
import { Component, VERSION } from '@angular/core';
import {HttpClientModule, HttpClient, HttpRequest, HttpResponse, HttpEventType} from '@angular/common/http';
@Component({
selector: 'my-app',
template: `
<input type="file" accept=".jpg,.png" class="button" (change)="upload($event.target.files)" />
<p>Upload Percent: {{percentDone}}% </p> <br />
<ng-container *ngIf="uploadSuccess" class="success">
<p class="sucess">Upload Successful</p>
</ng-container>
`,
styleUrls: ['./app.component.css'],
})
Export Components and Declare Variables in Angular
We will export our AppComponent
and declare variables percentDone
as the number
and UploadSuccess
as a boolean
. We will also declare a constructor, which calls HttpClient
.
# angular
export class AppComponent {
percentDone: number;
uploadSuccess: boolean;
constructor(private http: HttpClient) {}
Basic Upload For Multiple Images in Angular
There are 4 different styles of image upload in Angular; the basic upload of multiple images is one of them. Multiple images can be uploaded in this style without displaying a progress bar for images.
basicUploadImage(files: File[]) {
var formData = new FormData();
Array.from(files).forEach((f) => formData.append('file', f));
this.http.post('https://file.io', formData).subscribe((event) => {
console.log('done');
});
}
We are sending upload requests to https://file.io
, which can be changed with the backend server URL. The backend server will receive the uploaded images data, which will send a URL to the uploaded file.
Basic Upload For Single Image in Angular
The second style for uploading images in Angular is basic upload for an image file. In this style, the user can upload only one image at a time.
This style will also not display progress while images are uploading.
basicUploadSingleImage(file: File) {
this.http.post('https://file.io', file).subscribe((event) => {
console.log('done');
});
}
Upload and Progress For Multiple Images in Angular
The third style for uploading images in Angular is upload with progress for multiple images. The user can upload multiple images with a progress bar displaying the percentage uploaded in this style.
uploadImageAndProgress(files: File[]) {
console.log(files);
var formData = new FormData();
Array.from(files).forEach((f) => formData.append('file', f));
this.http.post('https://file.io', formData, {
reportProgress: true,
observe: 'events',
})
.subscribe((event) => {
if (event.type === HttpEventType.UploadProgress) {
this.percentDone = Math.round((100 * event.loaded) / event.total);
} else if (event instanceof HttpResponse) {
this.uploadSuccess = true;
}
});
}
Upload and Progress For Single Image in Angular
The fourth style for uploading images in Angular is a single image with a progress bar. The user can upload a single image with a progress bar displaying the percentage uploaded in this style.
uploadAndProgressSingleImage(file: File) {
this.http
.post('https://file.io', file, {
reportProgress: true,
observe: 'events',
})
.subscribe((event) => {
if (event.type === HttpEventType.UploadProgress) {
this.percentDone = Math.round((100 * event.loaded) / event.total);
} else if (event instanceof HttpResponse) {
this.uploadSuccess = true;
}
});
}
Calling the Upload Function in Angular
We have added four upload functions in Angular; we can call our upload function with the desired style.
uploadImage(files: File[]) {
this.uploadImageAndProgress(files);
}
Output:
Summary
We discussed four different styles of image upload in Angular and how we can call them in our upload
function. The full code is given below.
import { Component, VERSION } from '@angular/core';
import {
HttpClientModule,
HttpClient,
HttpRequest,
HttpResponse,
HttpEventType,
} from '@angular/common/http';
@Component({
selector: 'my-app',
template: `
<input type="file" accept=".jpg,.png" class="button" (change)="uploadImage($event.target.files)" />
<p>Upload Percent: {{percentDone}}% </p> <br />
<ng-container *ngIf="uploadSuccess" class="success">
<p class="sucess">Upload Successful</p>
</ng-container>
`,
styleUrls: ['./app.component.css'],
})
export class AppComponent {
percentDone: number;
uploadSuccess: boolean;
constructor(private http: HttpClient) {}
uploadImage(files: File[]) {
this.uploadImageAndProgress(files);
}
basicUploadImage(files: File[]) {
var formData = new FormData();
Array.from(files).forEach((f) => formData.append('file', f));
this.http.post('https://file.io', formData).subscribe((event) => {
console.log('done');
});
}
basicUploadSingleImage(file: File) {
this.http.post('https://file.io', file).subscribe((event) => {
console.log('done');
});
}
uploadImageAndProgress(files: File[]) {
console.log(files);
var formData = new FormData();
Array.from(files).forEach((f) => formData.append('file', f));
this.http.post('https://file.io', formData, {
reportProgress: true,
observe: 'events',
})
.subscribe((event) => {
if (event.type === HttpEventType.UploadProgress) {
this.percentDone = Math.round((100 * event.loaded) / event.total);
} else if (event instanceof HttpResponse) {
this.uploadSuccess = true;
}
});
}
uploadAndProgressSingleImage(file: File) {
this.http
.post('https://file.io', file, {
reportProgress: true,
observe: 'events',
})
.subscribe((event) => {
if (event.type === HttpEventType.UploadProgress) {
this.percentDone = Math.round((100 * event.loaded) / event.total);
} else if (event instanceof HttpResponse) {
this.uploadSuccess = true;
}
});
}
}
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn