Angular 2 Global Constants
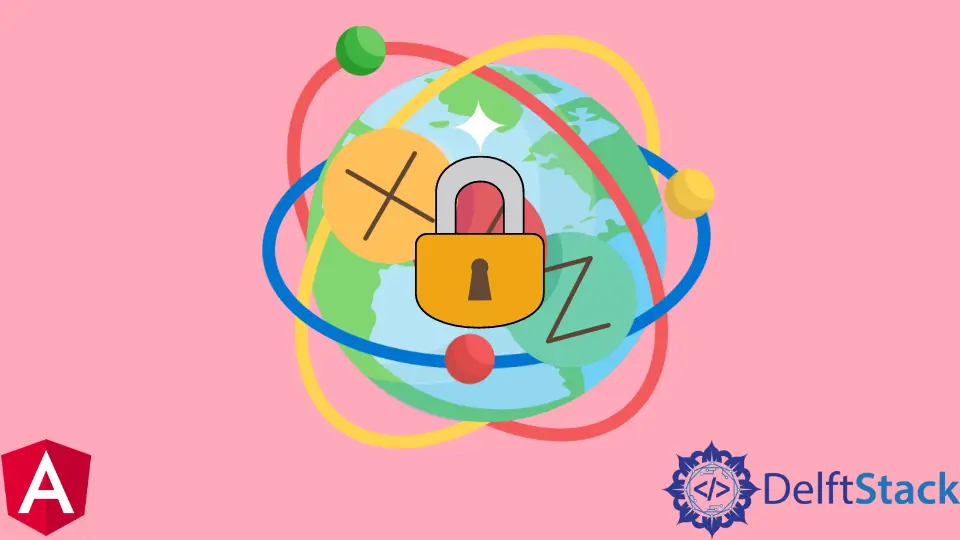
The Angular 2 framework has a lot of constants that are used by the different components in the framework. These constants can be accessed using the angular2 module, which is available at the root of your project directory.
To save our time, we take help from global constants. Angular 2 Global constants store a value in a global namespace.
They provide a way to keep track of data needed by all parts of the application. This is useful when we need to access the same value from different parts of the application.
Declare Angular 2 Global Constants
Angular 2 provides a mechanism to declare global constants. Global constants are declared using the const
keyword and can be accessed anywhere in the application.
It involves following the below steps.
- Create a constant file by adding the
.ts
extension to it. - Write out the constant value in the file and export it as a global variable by using the export default
const
keyword. - Import this file in your app module by using the import keyword followed by the path of your constant file.
Code Example:
const MY_CONSTANT = 'This is my constant';
// Use MY_CONSTANT in the rest of your app like this:
console.log(MY_CONSTANT);
Let’s discuss a complete example of Angular 2 global constants to better understand how it works.
Typescript Code:
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
readonly CONSTANTS = CONSTANTS;
global: { name: string; };
constructor() {
this.global = { name: 'demo' }
}
}
export const CONSTANTS = {
'globalName': 'demo'
}
Html Code:
<h1>Example of Angular 2 Global Constants</h1>
<span *ngIf="global.name === CONSTANTS.globalName ">
Hello World
</span>
Benefits of Global Constants
Angular 2 uses global constants to make it easier for developers to work with numbers. In Angular 2, global constants are used as an alternative to hard-coding values in code.
The benefits of using global constants in Angular 2 are the following.
- Developers can work with numbers more easily.
- The values can be changed without changing the code.
- Developers can make changes quickly.
- The developer doesn’t have to worry about typos or bugs because the compiler will catch them.
Click here to run the above code example.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook