Python os.unlink() Method
-
Syntax of
os.unlink()
Method -
Example 1: Use the
os.unlink()
Method to Remove or Delete a File Path -
Example 2: Use Conditional Statements With the
os.unlink()
Method -
Example 3: Removing Unused Image Files Using the
os.unlink()
Method -
Example 4: Handling Errors in the
os.unlink()
Method - Conclusion
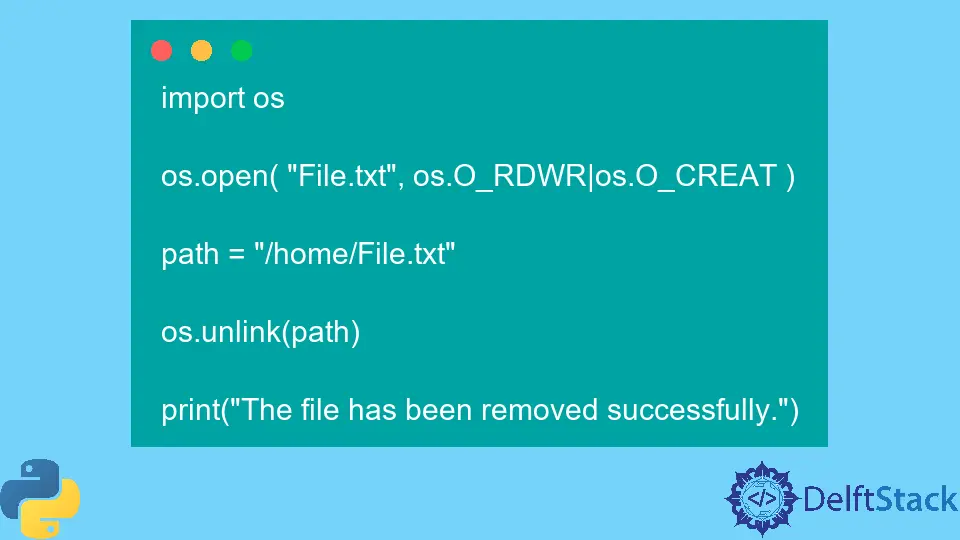
When working with files in Python, it’s essential to have mechanisms to manage them efficiently. One common task is deleting files programmatically, which can be achieved using various methods provided by Python’s built-in modules.
Among these methods, os.unlink()
stands out as a straightforward way to delete files.
The os.unlink()
function allows developers to delete a file specified by its path. This operation is irreversible, as it permanently removes the file from the file system.
Understanding how to use os.unlink()
is crucial for tasks involving file management, such as cleaning up temporary files, removing outdated data, or implementing file-related functionality in applications.
Syntax of os.unlink()
Method
os.unlink(path)
os.unlink(path, *, dir_fd=None)
Parameters
path |
It is an address object of a file system path or a symlink . The object can either be an str or bytes. |
* |
The asterisk shows that all following parameters are keyword-only. |
dir_fd |
It is an optional parameter representing a file descriptor referring to a path. Its default value is set as None . |
Return
In the execution process, this method does not return any value.
Example 1: Use the os.unlink()
Method to Remove or Delete a File Path
The code snippet showcases the usage of Python’s os.unlink()
function to delete a file named File.txt
.
This operation is performed after creating the file using os.open()
with specific file flags (os.O_RDWR
and os.O_CREAT
).
The purpose of this code is to demonstrate how to delete a file programmatically using os.unlink()
, highlighting its simplicity and effectiveness in file management tasks.
File.txt
:
Sample text file.
Example code:
import os
# Create a file named "File.txt" with read and write permissions and create the file if it does not exist
os.open("File.txt", os.O_RDWR | os.O_CREAT)
# Define the absolute path to the file to be deleted
# Full example of path: /home/File.txt
path = "File.txt"
# Delete the file specified by the path
os.unlink(path)
# Print a message confirming the successful removal of the file
print("The file has been removed successfully.")
We begin by importing the os
module, which provides operating system-dependent functionality, including file operations.
Next, we use os.open()
to create a file named File.txt
with the specified flags os.O_RDWR
(open for reading and writing) and os.O_CREAT
(create the file if it does not exist).
We define the path
variable, which stores the absolute path to the File.txt
that we want to delete.
Using os.unlink()
, we delete the file specified by the path
. This function call effectively removes the file from the file system.
Finally, we print a message indicating that the file has been successfully removed.
Output:
The file has been removed successfully.
This output confirms that the file File.txt
has been successfully deleted from the file system.
An OSError
might occur while using the os.unlink()
method due to invalid or inaccessible processes and paths.
Example 2: Use Conditional Statements With the os.unlink()
Method
This code snippet below demonstrates how to delete a file using the os.unlink()
function.
The script first checks if the file File.txt
exists using os.path.isfile()
. If the file exists, it is deleted using os.unlink()
, and a success message is printed.
Otherwise, if the file does not exist, a message indicating its absence is printed.
File.txt
:
Sample text file.
Example code:
import os
# Check if the file "File.txt" exists
if os.path.isfile("File.txt"):
# If the file exists, delete it
os.unlink("File.txt")
# Print a message confirming successful deletion
print("The file has been deleted successfully.")
else:
# If the file does not exist, print a message indicating its absence
print("The file does not exist.")
We begin by importing the os
module, which provides various operating system-dependent functionalities, including file operations.
The script checks if the file File.txt
exists using os.path.isfile()
. This function returns True
if the specified path points to a file.
If the file exists (os.path.isfile("File.txt")
evaluates to True
), the script proceeds to delete it using os.unlink("File.txt")
.
After successful deletion, a message confirming the deletion is printed: "The file has been deleted successfully."
.
If the file does not exist (os.path.isfile("File.txt")
evaluates to False
), the script prints a message indicating that the file does not exist: "The file does not exist."
.
Output:
The file does not exist.
The file does not exist in the directory, so the code executed the else
condition.
Alternatively, you can use the os.remove
method in the above code.
Example 3: Removing Unused Image Files Using the os.unlink()
Method
Let’s dive into a practical example.
In this example, we’ll demonstrate the usage of os.unlink()
to remove unused image files from a directory.
This scenario is common in web development projects, where temporary or outdated image files may accumulate over time, occupying disk space unnecessarily.
Example code:
import os
def remove_unused_images(directory):
for filename in os.listdir(directory):
if filename.endswith(".jpg") or filename.endswith(".png"):
file_path = os.path.join(directory, filename)
os.unlink(file_path)
print(f"Deleted: {file_path}")
# Directory containing image files
image_directory = "/path/to/images"
# Remove unused images
remove_unused_images(image_directory)
The remove_unused_images(directory)
function is designed to eliminate unused image files within a designated directory.
Within this function, a loop is employed to traverse through each file within the specified directory using os.listdir(directory)
.
For every file encountered, a check is performed to determine if its filename ends with .jpg
or .png
through filename.endswith(".jpg")
and filename.endswith(".png")
.
Files identified as image files undergo further processing: their absolute paths are constructed using os.path.join(directory, filename)
, and subsequently, they are deleted using os.unlink(file_path)
.
In order to provide feedback on the deletion process, a message indicating which file has been deleted is printed.
Finally, the function is invoked with the directory path parameter to initiate the removal of unused image files.
This systematic approach to file management streamlines the process of decluttering directories, enhancing storage efficiency and overall application performance.
Example output:
Deleted: /path/to/images/image1.jpg
Deleted: /path/to/images/image2.png
Deleted: /path/to/images/image3.jpg
As we can see, image files with .jpg
and .png
were deleted.
By incorporating this method into your projects, you can effectively clean up unnecessary files, optimize storage space, and improve the efficiency of your applications.
Example 4: Handling Errors in the os.unlink()
Method
This Python code snippet demonstrates file deletion using os.unlink()
. It attempts to delete the file specified by the path /home/User/File.txt
.
The script handles various potential errors that may occur during the deletion process, such as the file not existing, insufficient permissions, or attempting to delete a directory instead of a file.
File.txt
:
Sample text file.
Example code:
import os
# Define the absolute path to the file to be deleted
path = "/home/User/File.txt"
try:
# Attempt to delete the file specified by the path
os.unlink(path)
# Print a message confirming successful deletion
print("The file path has been removed successfully.")
except IsADirectoryError:
# Handle the case where the given path points to a directory instead of a file
print("The given path is a directory, and it can't be removed with this method.")
except FileNotFoundError:
# Handle the case where the file specified by the path does not exist
print("No such file or directory has been found.")
except PermissionError:
# Handle the case where access to the file is denied due to insufficient permissions
print("Access denied")
except:
# Handle any other unexpected errors that may occur during the deletion process
print("The file can not be deleted.")
We begin by importing the os
module, which provides various operating system-dependent functionalities, including file operations.
The script defines the path
variable, representing the absolute path to the file to be deleted (/home/User/File.txt
in this case).
Inside a try
block, the script attempts to delete the file specified by the path
using os.unlink(path)
.
If the deletion is successful, a message confirming the successful removal of the file is printed: "The file path has been removed successfully."
.
The script includes multiple except
blocks to handle specific exceptions that may occur during the deletion process:
IsADirectoryError
: If the given path points to a directory instead of a file, this exception is raised. The script prints a message indicating that the given path is a directory and cannot be removed using this method.FileNotFoundError
: If the file specified by the path does not exist, this exception is raised. The script prints a message indicating that no such file or directory has been found.PermissionError
: If the script encounters a permission error while attempting to delete the file, this exception is raised. The script prints a message indicating that access is denied.- Generic
except
block: This block catches any other unexpected errors that may occur during the deletion process and prints a generic error message indicating that the file cannot be deleted.
Output:
No such file or directory has been found.
Note that the os.unlink()
method cannot remove a directory. If the parameter entered is a directory, then the IsADirectoryError
error is thrown.
Alternatively, the os.rmdir()
method can be used to remove a valid path or directory.
Conclusion
In this exploration of os.unlink()
in Python, we’ve covered its application in deleting files, handling errors, and improving file management efficiency.
The provided examples demonstrate its versatility in various scenarios, from removing specific file types like images to handling different error conditions gracefully.
By utilizing os.unlink()
, developers can ensure effective file deletion while maintaining robust error handling mechanisms.
Moreover, the detailed explanations and code walkthroughs offer insights into its usage and the importance of proper error handling in file management tasks.
Whether it’s cleaning up unnecessary files or handling unexpected scenarios, os.unlink()
proves to be a valuable tool in Python’s file manipulation arsenal.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn