Python os.path.dirname() Method
-
Syntax of the
os.path.dirname()
Method -
Example 1: Use the
os.path.dirname()
Method in Python - Example 2: Get the Parent and Current Directory in Python
-
Example 3: Use the Absolute Path Inside the
os.path.dirname
Method as an Argument in Python -
Example 4:
os.path.dirname
One Level Up in Python -
Example 5: Use
sys.argv[0]
in theos.path.dirname()
Method in Python
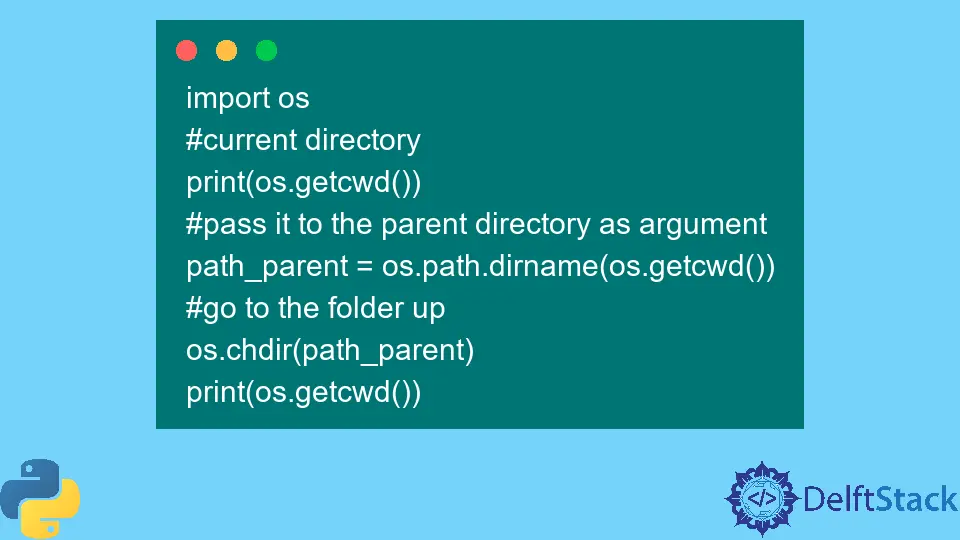
For communicating with the operating system, the OS module provides different methods to interact with it. One method is os.path.dirname
, which returns the provided path’s directory.
In the method os.path.dirname
, path
is sent as an argument. The syntax of this method is shown below.
Syntax of the os.path.dirname()
Method
os.path.dirname(path)
Parameters
path |
path refers to the directory of the file |
Return
This method will return a string type value consisting of the directory from the provided path.
Example 1: Use the os.path.dirname()
Method in Python
We must first import the os
module to use the os.path.dirname
method. After importing, we can use the os.path.dirname
method.
We can send the argument in multiple ways, for example, writing the file directory along with filename and extension, sending the file directory alone (no file name), or even sending the file name only.
# importing the os.path module
import os.path
# sending argument as the file directory with filename and extension
path = "/home/Junaid/Myfolder/myfile.doc"
dirname = os.path.dirname(path)
print(dirname)
# sending argument as the file directory only
path = "/home/Junaid/Myfolder"
dirname = os.path.dirname(path)
print(dirname)
# sending argument as filename only
path = "myfile.doc"
dirname = os.path.dirname(path)
print(dirname)
Output:
/home/Junaid/Myfolder
/home/Junaid/
Nothing will be printed in the third line of output as the dirname
argument doesn’t contain any directory. From the outputs above, we can also say that the os.path.dirname
method only returns the head part of the path directory.
The important thing is to see that the dirname
method can be viewed as a wrapper of the os.path.split
method, which splits the directory path into two things, i.e. head
and tail
. The tail
contains the last part of the directory path, while the head
contains the rest of the part.
Example 2: Get the Parent and Current Directory in Python
To get the parent and child directory from the specified path, we can use the os.getcwd
and os.path.dirname()
methods, respectively.
import os
# get the current directory
current_path = os.getcwd()
print("My current directory is : ", current_path)
# parent directory
parent_directory = os.path.dirname(current_path)
print("My parent directory is : ", parent_directory)
Output:
My current directory is : D:\myfolder\Pythonfiles
My parent directory is : D:\myfolder
Example 3: Use the Absolute Path Inside the os.path.dirname
Method as an Argument in Python
The os.path.abspath
method returns an absolute version of the path name. So, looking at the below example, we can either pass the string or os.path.abspath
method as an argument to the dirname
method.
import os
path = "D:\Pythonfiles\myfile.doc"
# path_name1 is already an absolute path
path_name1 = os.path.dirname(path)
# path_name2 uses abspath() method to return absolte path
path_name2 = os.path.dirname(os.path.abspath(path))
print(path_name1)
print(path_name2)
Output:
D:\Pythonfiles
D:\Pythonfiles
The above example shows that the output is the same for both methods, even though we have used the os.path.abspath
method with path_name2
. The reason is that the method os.path.abspath
returns the absolute path, and the string path provided as an argument in path_name1
is already the absolute path of the module.
Hence, we can say that os.path.dirname(os.path.abspath(path))
is same as os.path.dirname(path)
.
Example 4: os.path.dirname
One Level Up in Python
Until now, we can see that we can get the current directory of the provided path; however if we want to go one level up in the provided directory.
We can use the following statements to do so.
import os
# current directory
print(os.getcwd())
# pass it to the parent directory as argument
path_parent = os.path.dirname(os.getcwd())
# go to the folder up
os.chdir(path_parent)
print(os.getcwd())
Output:
C:\Users\lenovo
C:\Users
In this example, we call the os.getcwd()
method if we want to get the current working directory. Then we call the os.path.dirname
method to go a folder up to the specified path.
Finally, we call the os.chdir()
method) to move to the new directory.
Example 5: Use sys.argv[0]
in the os.path.dirname()
Method in Python
The method sys.argv[0]
contains the script’s name we are working on. This method may or may not contain the path information and will show the script name exactly as it appears on the command line.
Let’s assume we are working on a Python script named mywork.py
(here, .py
is the extension for the Python script file). Let’s look at the following example to further understand this method.
import sys
import os
print("The command sys.argv[0] outputs =", sys.argv[0])
pathname = os.path.dirname(sys.argv[0])
print("path to the file =", pathname)
print("full path to the file =", os.path.abspath(pathname))
Output:
The command sys.argv[0] outputs = C:\Users\lenovo\mywork.py
path to the file = C:\Users\lenovo
full path to the file = C:\Users\lenovo
Here the output shows the full path along with the script’s name, for example, mywork.py
by using the method sys.argv[0]
. Passing the sys.argv[0]
to the method os.path.dirname
as an argument will output the file directory as the full path.
Hi, I'm Junaid. I am a freelance software developer and a content writer. For the last 3 years, I have been working and coding with Python. Additionally, I have a huge interest in developing native and hybrid mobile applications.
LinkedIn