Python math.pow() Method
-
Syntax of Python
math.pow()
Method -
Example 1: Use the
math.pow()
Method in Python -
Example 2: Shorter Syntax of the
math.pow()
Method -
Example 3: Calculate the Square and Square Root of a Number Using the
math.pow()
Method -
Example 4: Errors When Using the
math.pow()
Method
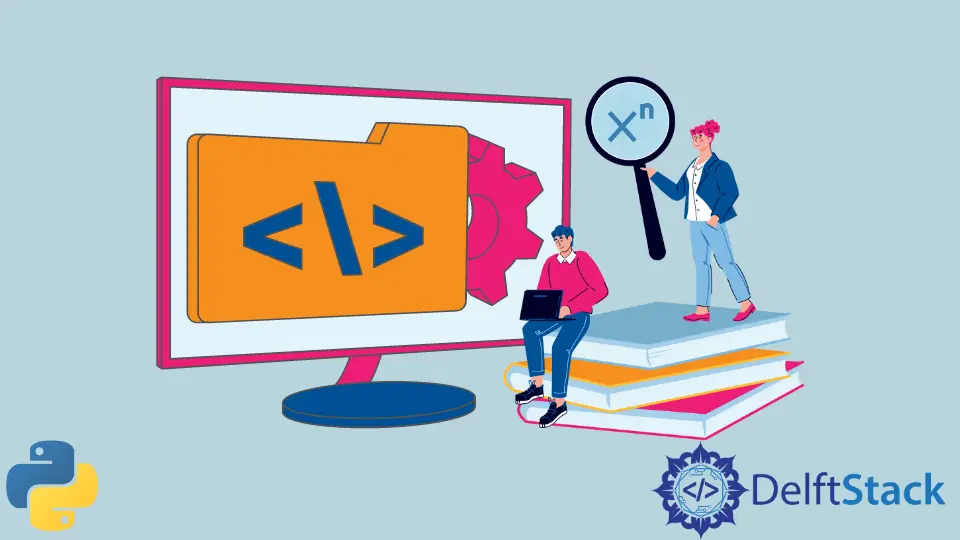
Python math.pow()
method is an efficient way of calculating the value of the number x
raised to the power of another number, y
.
Syntax of Python math.pow()
Method
math.pow(x, y)
Parameters
Parameters | Description |
---|---|
x |
Any positive or negative number representing the base. |
y |
Any positive or negative number representing the power. |
Return
This method returns a float/integer value depending on the input parameters. It returns x^y.
Example 1: Use the math.pow()
Method in Python
import math
x = 2
y = 4
value = math.pow(x, y)
print(f"{x} raised to the power {y} is {value}.")
x = 6
y = -3
value = math.pow(x, y)
print(f"{x} raised to the power {y} is {value}.")
x = math.inf
y = 4
value = math.pow(x, y)
print(f"{x} raised to the power {y} is {value}.")
x = 1
y = math.inf
value = math.pow(x, y)
print(f"{x} raised to the power {y} is {value}.")
Output:
2 raised to the power 4 is 16.0.
6 raised to the power -3 is 0.004629629629629629.
inf raised to the power 4 is inf.
1 raised to the power inf is 1.0.
Note that the arguments can be in integers or floats. The values may be either positive or negative.
Example 2: Shorter Syntax of the math.pow()
Method
import math
print("2 raised to the power 5 is ", math.pow(2, 5))
Output:
2 raised to the power 5 is 32.0
These methods are used in mathematical computations related to geometry and have a certain application in astronomical computations.
Example 3: Calculate the Square and Square Root of a Number Using the math.pow()
Method
import math
x = 4
y = 2
z = 1 / 2
value = math.pow(x, y)
print(f"The square of {x} is {value}.")
value = math.pow(x, z)
print(f"The square root of {x} is {value}.")
Output:
The square of 4 is 16.0.
The square root of 4 is 2.0.
Adjusting the value of the second parameter of the math.pow()
method can help us to calculate the roots and powers of any number x
.
Example 4: Errors When Using the math.pow()
Method
import math
x = -2 # x is negative
y = 1.5 # y is not an integer
value = math.pow(x, y)
print(f"The square of {x} is {value}.")
x = 4
y = 1 + 4j # complex number
value = math.pow(x, y)
print(f"The square of {x} is {value}.")
x = -2
y = "hello" # string
value = math.pow(x, y)
print(f"The square of {x} is {value}.")
Output:
Traceback (most recent call last):
File "main.py", line 7, in <module>
value=math.pow(x, y)
ValueError: math domain error
Traceback (most recent call last):
File "main.py", line 15, in <module>
value=math.pow(x, y)
TypeError: can't convert complex to float
Traceback (most recent call last):
File "main.py", line 24, in <module>
value=math.pow(x, y)
TypeError: must be real number, not str
Note that if both the parameters of math.pow()
are finite numbers and x
is negative but y
is not an integer, then a ValueError
exception is thrown.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn