JavaScript boolean.valueOf() Method
-
Syntax of JavaScript
boolean.valueOf()
Method -
Example 1: Use
boolean.valueOf()
to Get the Primitive Value of an Object -
Example 2: Use
boolean.valueOf()
to Use Primitive Value of an Object With a String -
Example 3: Create Boolean Object With Integer Value and Use the
Boolean.valueOf()
Method -
Example 4: Create a Boolean Object With Undefined Value and Use With the
Boolean.valueOf()
Method -
Example 5: Create a Boolean Object With Non-Integer or Non-String Value and Use With the
Boolean.valueOf()
Method
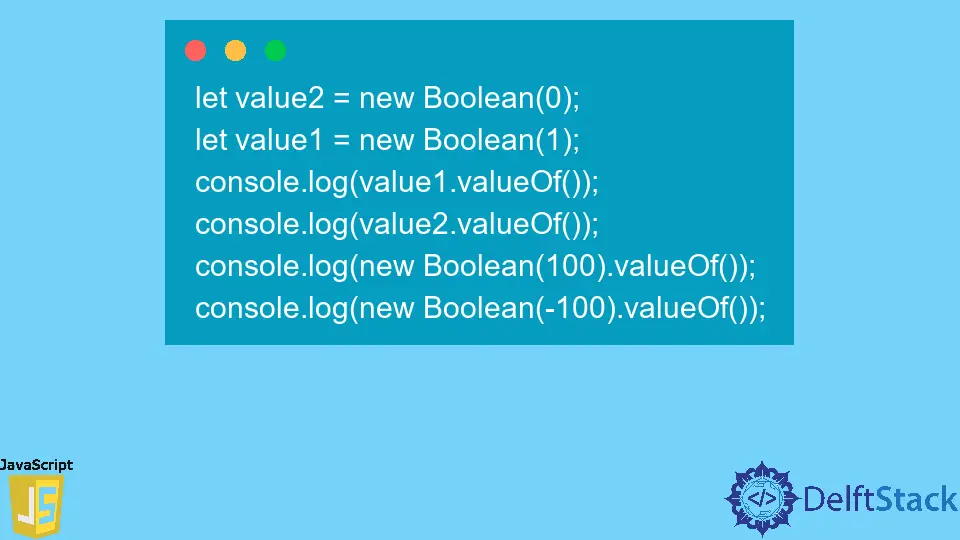
In JavaScript, the boolean.valueOf()
method allows us to get the primitive value of a Boolean object. This method is mostly used internally and not inside the code.
Syntax of JavaScript boolean.valueOf()
Method
boolean.valueOf();
Parameters
This method doesn’t contain any parameters.
Return
This method returns the primitive value of a Boolean object (true
or false
).
Example 1: Use boolean.valueOf()
to Get the Primitive Value of an Object
In JavaScript, the boolean.valueOf()
method provides the primitive value of an object so that it can be used internally. In this example, we have created an object, and by using the boolean.valueOf()
method, we will get its primitive value.
let boolean = new Boolean(false);
let string = boolean.valueOf();
console.log(string);
Output:
false
Example 2: Use boolean.valueOf()
to Use Primitive Value of an Object With a String
In JavaScript, the boolean.valueOf()
method allows us to use the primitive value of an object with another string. In this example, we have created a string and an object.
We got the string and primitive value as the output using the boolean.valueOf()
method.
let ques ="Are you on DelftStack? :";
let ans = new Boolean(true);
let text = ques + ans.valueOf();
console.log(text);
Output:
Are you on DelftStack? : true
Example 3: Create Boolean Object With Integer Value and Use the Boolean.valueOf()
Method
In this example below, we have taken the various integers and created the Boolean objects using that.
When we use the valueOf()
method on that object, it returns false
for 0 and true
for all other numeric values.
let value2 = new Boolean(0);
let value1 = new Boolean(1);
console.log(value1.valueOf());
console.log(value2.valueOf());
console.log(new Boolean(100).valueOf());
console.log(new Boolean(-100).valueOf());
Output:
true
false
true
true
Example 4: Create a Boolean Object With Undefined Value and Use With the Boolean.valueOf()
Method
The primitive Boolean value of the all falsy value is false
. In JavaScript, undefined
, null
, NaN
, etc are the falsy values and Boolean.valueOf()
method always returns the false
Boolean values for these types of values.
let value2 = new Boolean(undefined);
let value1 = new Boolean(null);
console.log(value1.valueOf());
console.log(value2.valueOf());
console.log(new Boolean(NaN).valueOf());
Output:
false
false
false
Example 5: Create a Boolean Object With Non-Integer or Non-String Value and Use With the Boolean.valueOf()
Method
For all non-string or non-integer truly values, Boolean.valueOf()
method always returns true
Boolean value.
In this example below, we have created the array of 2 elements and an empty object using the Boolean()
constructor and invoked the valueOf()
method to get the primitive true
or false
values. Users can observe the output for the different inputs.
let value2 = new Boolean(["Delft","stack"]);
let value1 = new Boolean({});
console.log(value1.valueOf());
console.log(value2.valueOf());
Output:
true
true
The boolean.valueOf()
method is supported in all browsers.