JavaScript Array.concat() Method
-
Syntax of JavaScript
array.concat()
Method -
Example Codes: Use the
array.concat()
Method to Merge Two Arrays -
Example Codes: Use the
array.concat()
Method to Merge Three Arrays -
Example Codes: Use the
array.concat()
Method to Merge Values and Arrays Together
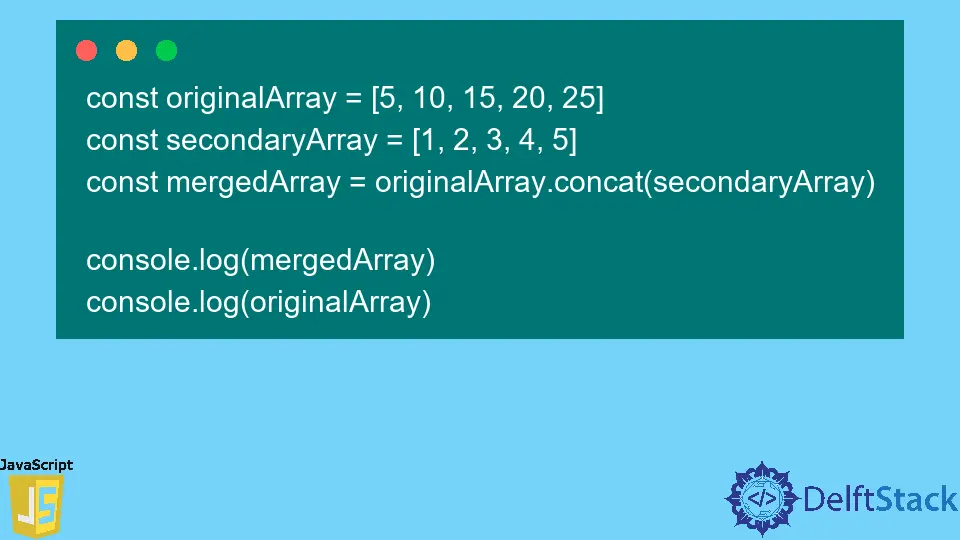
The array in JavaScript is considered a single variable used to store multiple values. To join two or more arrays in JavaScript, the array.concat()
method is used.
This method does not make any changes to the original array. Instead, it creates a shallow copy of the original array and merges the value of the new array along with the value of the original array.
Syntax of JavaScript array.concat()
Method
let newArray = array.concat(value1, value2, ....., valueN)
Parameter
ValueN |
This can be arrays, values, or both. The value will be concatenated with the values of the original array. |
If no value is passed as the parameter, this method will return a shallow copy of the original array. |
Return
After merging the values or arrays with the original array, a new array is returned.
Example Codes: Use the array.concat()
Method to Merge Two Arrays
const originalArray = [5, 10, 15, 20, 25]
const secondaryArray = [1, 2, 3, 4, 5]
const mergedArray = originalArray.concat(secondaryArray)
console.log(mergedArray)
console.log(originalArray)
Output:
[ 5, 10, 15, 20, 25, 1, 2, 3, 4, 5 ]
[ 5, 10, 15, 20, 25 ]
We have taken two arrays and merged the secondaryArray
with the originalArray
using the array.concat()
method. This method does not change the original array and returns the result in a new array.
We have stored the result in the mergedArray
and printed the original array to check whether any changes occurred or not.
Example Codes: Use the array.concat()
Method to Merge Three Arrays
const fruitArray = ['Banana', 'Apple', 'Mango', 'Orange']
const quantityArray = [7, 2, 13, 4]
const priceArray = [14, 5, 20, 10 ]
const mergedArray = fruitArray.concat(quantityArray, priceArray)
console.log(mergedArray)
Output:
[ 'Banana', 'Apple', 'Mango', 'Orange', 7, 2, 13, 4, 14, 5, 20, 10 ]
The quantityArray
and priceArray
have been merged with the fruitArray
. We passed the name of the arrays inside the array.concat()
method and stored the result in the mergedArray
.
Example Codes: Use the array.concat()
Method to Merge Values and Arrays Together
const originalArray = ['I', 'II', 'III', 'IV']
const arr2 = [1, 2, 3, 4]
const mergedArray = originalArray.concat('A', 'B', 'C', 'D', arr2)
console.log(mergedArray)
Output:
[ 'I', 'II', 'III', 'IV', 'A', 'B', 'C', 'D', 1, 2, 3, 4 ]
We have defined two arrays above. One is the originalArray
, and the other is arr2
.
After that, we passed some values and the arr2
as the argument of the array.concat()
method. This method merged them with the originalArray
.
Niaz is a professional full-stack developer as well as a thinker, problem-solver, and writer. He loves to share his experience with his writings.
LinkedIn