Python 教程 - 异常处理
Jinku Hu
2023年1月30日
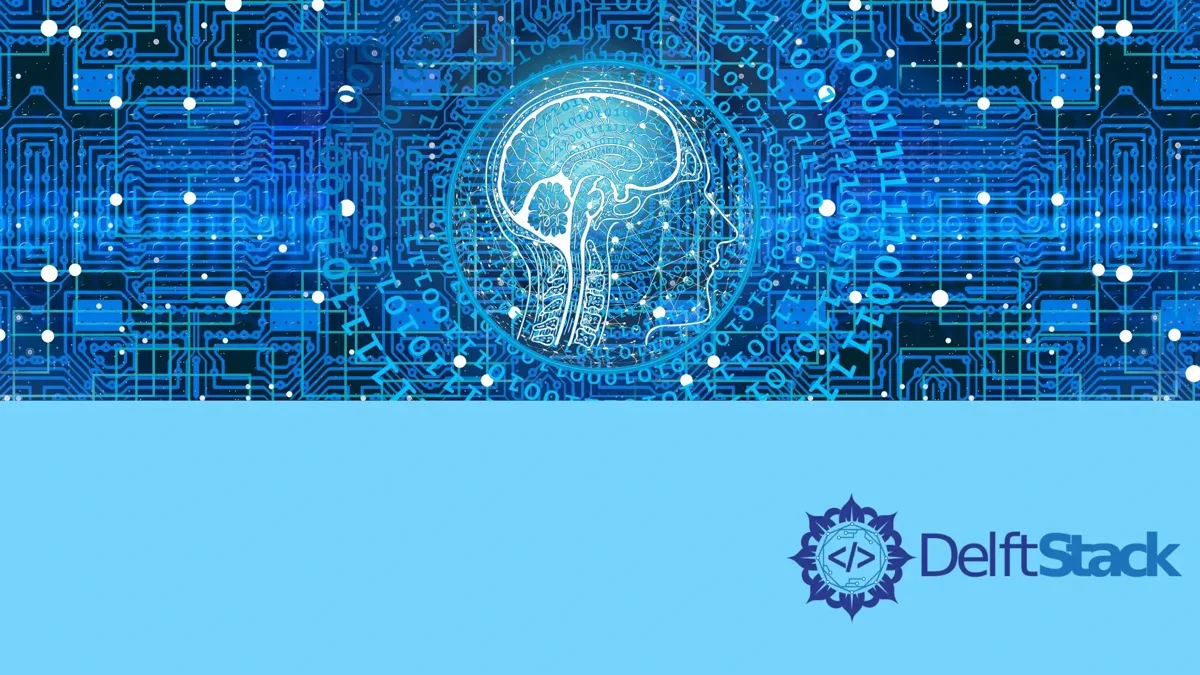
你将在本节中学习如何处理 Python 异常。此外,你将学习引发和捕获异常。
当程序中发生异常时,程序执行将终止。但是大多数时候,你不希望程序异常终止,因此你可以使用 Python 异常处理来避免程序崩溃。
try...except
在下面的示例中,要求用户输入 10 个整数。如果用户输入其他类型而不是整数,则将引发异常并执行 except
中的语句:
>>> n = 10
>>> i = 1
>>> sum = 0
>>> while i <= n:
try:
num = int(input('Enter a number: '))
sum = sum + num
i = i+1
except:
print('Please enter an integer!!')
break
Enter a number: 23
Enter a number: 12
Enter a number: Asd
Please enter an integer!!
>>> print('Sum of numbers =', sum)
Sum of numbers = 35
你还可以捕获指定的异常,例如,如果发生被零除的情况,则可以捕获对应的异常为:
try:
# statements
except ZeroDivisionError:
# exception handling
except:
# all other exceptions are handled here
raise
异常
你可以使用 raise
关键字强制发生特定的异常:
>>> raise ZeroDivisionError
Traceback (most recent call last):
File "<pyshell#17>", line 1, in <module>
raise ZeroDivisionError
ZeroDivisionError
>>> raise ValueError
Traceback (most recent call last):
File "<pyshell#18>", line 1, in <module>
raise ValueError
ValueError
如果需要向自举异常添加一些自定义信息,则可以将其添加到异常错误类型后的括号中。
>>> raise NameError("This is a customized exception")
Traceback (most recent call last):
File "<pyshell#18>", line 1, in <module>
raise NameError("This is a customized exception")
NameError: This is a customized exception
try...finally
finally
在异常处理 try
中是一个可选项。如果有一个 finally
块,无论结果如何,程序都会执行它。
在以下示例中我们对此进行演示:
>>> try:
raise ValueError
finally:
print('You are in finally block')
You are in finally block
Traceback (most recent call last):
File "<pyshell#23>", line 2, in <module>
raise ValueError
ValueError
try...finally
结构用于清理操作,例如在处理文件时,最好关闭文件。因此,文件将在 finally
块中关闭。
Python 内置异常
在本节中,你将学习 Python 编程中的标准异常。
异常是解释期间引发的运行时错误。例如,将任何数字除以零将产生一个称为 ZeroDivisionError
的运行时错误。
在 Python 中,下面描述了一些内置的异常:
异常 | 什么时候触发? |
---|---|
AssertionError |
当 assert 语句失败 |
AttributeError |
当 attribute 引用失败时 |
EOFError |
当 input() 函数未读取任何数据并到达文件末尾时 |
FloatingPointError |
当浮点计算失败时 |
GeneratorExit |
当协程或生成器关闭时,GeneratorExit 发生异常 |
ImportError |
当 import 找不到模块时 |
ModuleNotFoundError |
当 import 找不到模块时。此异常是 ImportError 的子类 |
IndexError |
当序列的索引不在范围内时 |
KeyError |
当在字典中找不到字典键时 |
KeyboardInterrupt |
当按下中断键时 |
MemoryError |
当指定操作的内存量较少时 |
NameError |
当找不到变量时 |
NotImplementedError |
需要但不提供抽象方法的实现时 |
OSError |
遇到系统错误时 |
OverFlowError |
当值太大而无法表示时 |
RecursionError |
当递归限制超过最大数量时 |
IndentationError |
为任何函数,类等的定义正确指定缩进时 |
SystemError |
当发现与系统相关的错误时 |
SystemExit |
当 sys.exit() 用于退出解释器时 |
TypeError |
当操作对于指定的数据类型无效时 |
ValueError |
当内置函数具有有效的自变量但提供的值无效时 |
RunTimeError |
当生成的错误不在其他类别中时 |
IOError |
当 I/O 操作失败时 |
UnBoundLocalError |
当访问局部变量但没有值时 |
SyntaxError |
当有语法错误时 |
TabError |
当有不必要的制表符缩进时 |
UnicodeError |
当遇到 Unicode 相关错误时 |
UnicodeEncodeError |
由于编码遇到 Unicode 相关错误时 |
UnicodeDecodeError |
由于解码遇到 Unicode 相关错误时 |
UnicodeTranslateError |
由于翻译而遇到 Unicode 相关错误时 |
ZeroDivisionError |
当数字除以零时 |
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe