Python 数据类型 - 字符串
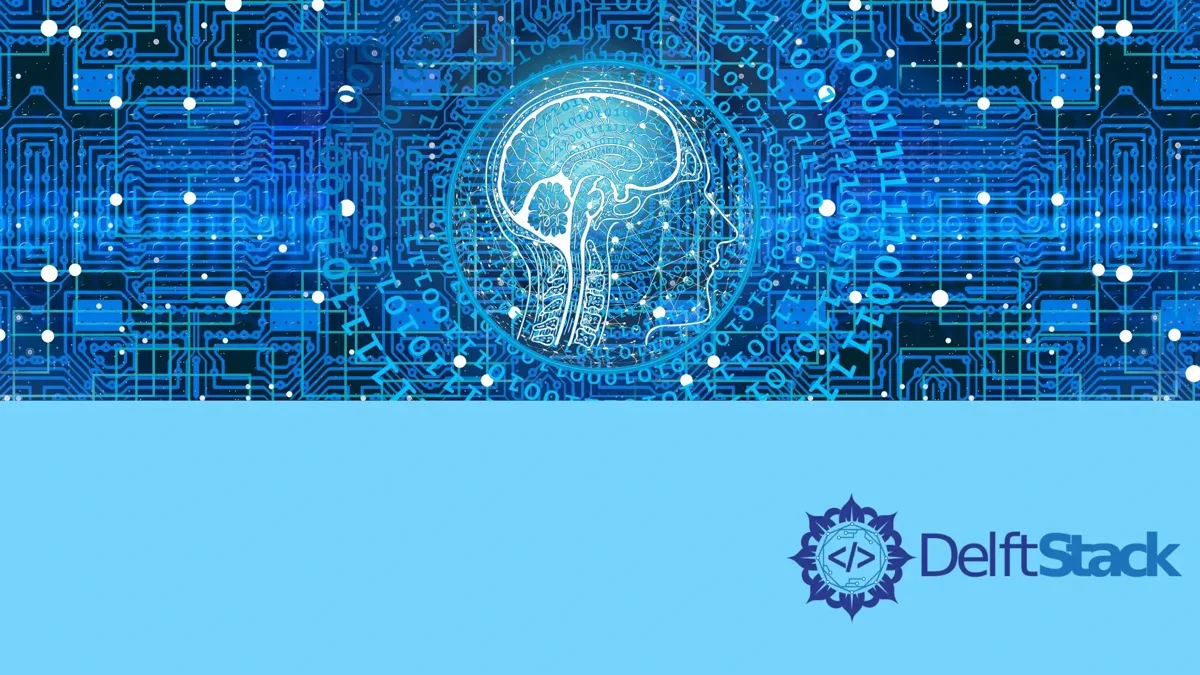
我们将在本节中介绍 Python 的字符串数据类型。
字符串是一系列字符,由计算机中的二进制数据表示。在 Python 中,字符串是 unicode
字符的集合。
创建字符串
可以使用单引号或双引号创建字符串,也可以使用三引号创建多行字符串。
x = "String of characters"
x = """Multiline
string"""
访问字符串元素
切片操作符 []
用来访问字符串中的字符,索引是跟 Python 其他的数据类型一样是从 0 开始的。
>>> x = 'Python Programming'
>>> print('x[0] =', x[0])
'P'
>>> print('x[4:15] =', x[4:15])
'on Programm'
>>> x[4] = 'l'
TypeError: 'str' object does not support item assignment
TypeError
报错。负索引
你可以通过负索引来访问字符串中的字符,比如 -1
就是指向最后一个字符,类似的,-2
指向倒数第二个字符。
>>> s = "Python"
>>> print(s[-1])
'n'
>>> print(s[-2])
'o'
>>> print(s[-3])
'h'
删除字符串中的字符
由于字符串是不可变的,因此无法删除字符串中的字符。但是你可以为同一变量指定一个新字符串。例如,
>>> s = 'Python'
>>> s = 'Program'
>>> s
'Program'
整个字符串可以用 del
关键字来删除,
>>> del s
Python 字符串操作
连接两个或多个字符串
你可以通过来+
号连接其两个或多个字符串。
>>> s1 = 'Python '
>>> s2 = 'Programming'
>>> print(s1 + s2)
'Python Programming'
如果你把两个字符串写在一起,它们会跟用+
一样,互相连接起来。
>>> print('Python ' 'Programming')
'Python Programming'
括号也可以用来连接在多行内的不同的字符串,这个在需要分叉长字符串(比如文件路径)的时候非常实用。
>>> s = ('Python '
'Programming')
>>> s
'Pyhton Programming'
遍历字符串
可以通过 for
循环来遍历整个字符串,
s = "Python"
for i in s:
print(i)
P
y
t
h
o
n
字符串成员检查
通过 in
关键字可以来检查某个字符或者字符串是否存在于该字符串中。
>>> s = 'Python'
>>> print('a' in s)
False
>>> print('Py' in s)
True
适用于字符串的内置函数
应用于其他序列数据类型的函数也可适用于字符串。常用的函数比如 len()
来查找字符串中的字符数,enumerate()
则返回一个包含索引的对象和字符串中元素的值作为一对数据的数据对象。
Python 字符串格式化
转义
你可以通过 string-escape 来转义某些特殊的字符,这些特殊字符在 Python 中有特别的含义。比如,如果你想输出
James asked, "Do you know Andrew's place?"
你在这里既不能用单引号,也不能用双引号,因为字符串中含有了这两种引号。如果你试着把这段文字放在单引号或双引号内,你会得到 SyntaxError
错误。
>>> print("James asked, "Do you know Andrew's place?"")
SyntaxError: invalid syntax
>>> print('James asked, "Do you know Andrew's place?"')
SyntaxError: invalid syntax
解决方法就是要么你用三个单引号'''
,要么你就用转义方法。在 Python 中,转义是以反斜杠\
来开始的,\
用来给解释器提示这里有特殊的处理。
# using triple quotation marks
print('''James asked, "Do you know Andrew's place?"''')
# escaping single quotes
print('James asked, "Do you know Andrew\'s place?"')
# escaping double quotes
print('James asked, "Do you know Andrew\'s place?"')
下表当中列出了 Python 当中的转义字符。
Escape sequence | Description |
---|---|
\\ |
反斜杠 |
\' |
单引号 |
\" |
双引号 |
\a |
ASCII 声音警示 |
\b |
后退键 |
\n |
新建行 |
\r |
回车 |
\t |
水平制表符 |
\v |
竖直制表符 |
\ooo |
八进制符 ooo |
\xHH |
十六进制符 HH |
>>> print("C:\\User\\Python36-32\\Lib")
C:\User\Python36-32\Lib
>>> print("First Line\nSecond Line")
First Line
Second Line
用原始字符串 r
来省略转义
你可以在字符串前加 r
或者 R
来把字符串变成原始字符串,这样就省略了转义字符。
比如下面的例子,
#without r
>>> print("C:\\User\\Python36-32\\Lib")
C:\User\Python36-32\Lib
#with r
>>> print(r"C:\\User\\Python36-32\\Lib")
C:\\User\\Python36-32\\Lib
字符串 format()
方法
format()
方法是用来对字符串进行格式化的一把利器,在格式化的字符串中,占位符 {}
将会被 format()
方法中的字符串来替代。
你可以通过位置或者关键字参数来制定替代的顺序。
#default placeholder
>>> s1 = "{}, {} and {}".format('Bread', 'Butter', 'Chocolate')
>>> print(s1)
'Bread, Butter and Chocolate'
#positional arguments
>>> s2 = "{1}, {2} and {0}".format('Bread', 'Butter', 'Chocolate')
>>> print(s2)
'Butter, Chocolate and Bread'
#keyword arguments
>>> s3 = "{a}, {x} and {c}".format(x = 'Bread', c = 'Butter', a = 'Chocolate')
>>> print(s3)
'Chocolate, Bread and Butter'