PyQt5 教程 - 按钮
Jinku Hu
2024年2月15日
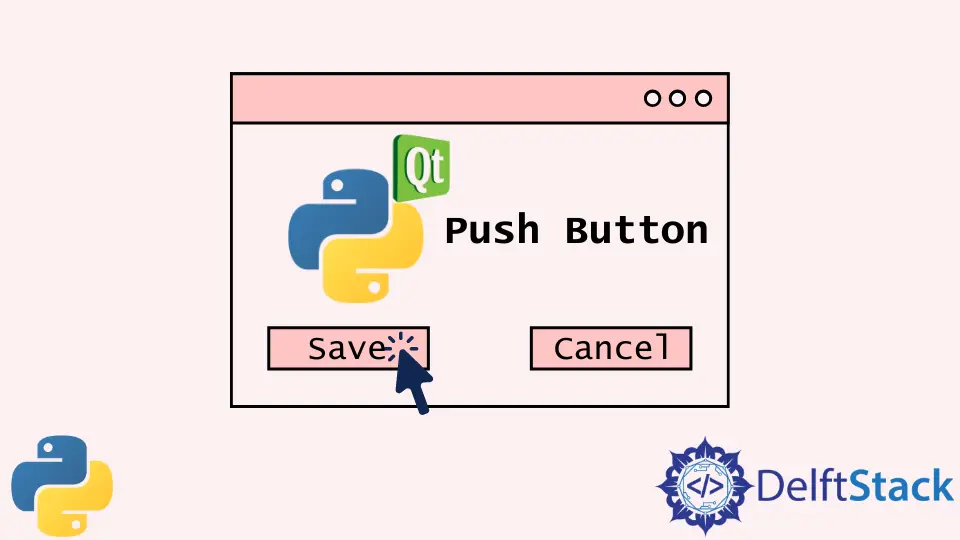
按钮控件 QPushButton
是 PyQt5 中的命令按钮。用户单击它命令 PC 执行某些特定操作,如确认、取消或保存等。
PyQt5 按钮 - QPushButton
import sys
from PyQt5 import QtWidgets
def basicWindow():
app = QtWidgets.QApplication(sys.argv)
windowExample = QtWidgets.QWidget()
buttonA = QtWidgets.QPushButton(windowExample)
labelA = QtWidgets.QLabel(windowExample)
buttonA.setText("Click!")
labelA.setText("Show Label")
windowExample.setWindowTitle("Push Button Example")
buttonA.move(100, 50)
labelA.move(110, 100)
windowExample.setGeometry(100, 100, 300, 200)
windowExample.show()
sys.exit(app.exec_())
basicWindow()
这里,
buttonA = QtWidgets.QPushButton(windowExample)
buttonA
是来自 QtWidgets
的 QPushButton
,它会被添加到窗口 windowExample
中,与前面章节中介绍的按钮相同。
buttonA.setText("Click!")
它将 buttonA
的文本设置为 Click!
。
实际上,按钮现在不会做任何事情。
PyQt5 QLabel
按钮控件集样式
QLabel
可以使用 setStyleSheet
方法设置 PyQt5 控件的样式,例如背景色、字体系列和字体大小。它的工作方式类似于 CSS
中的样式表。
buttonA.setStyleSheet(
"background-color: red;font-size:18px;font-family:Times New Roman;"
)
它设置 buttonA
了以下样式,
样式 | 值 |
---|---|
background-color |
red |
font-size |
18px |
font-family |
Times New Roman |
在 PyQt5 中设置样式很方便,因为它类似于 CSS。
import sys
from PyQt5 import QtWidgets
def basicWindow():
app = QtWidgets.QApplication(sys.argv)
windowExample = QtWidgets.QWidget()
buttonA = QtWidgets.QPushButton(windowExample)
labelA = QtWidgets.QLabel(windowExample)
buttonA.setStyleSheet(
"background-color: red;font-size:18px;font-family:Times New Roman;"
)
buttonA.setText("Click!")
labelA.setText("Show Label")
windowExample.setWindowTitle("Push Button Example")
buttonA.move(100, 50)
labelA.move(110, 100)
windowExample.setGeometry(100, 100, 300, 200)
windowExample.show()
sys.exit(app.exec_())
basicWindow()
PyQt5 QLabel
按钮点击事件
按钮单击事件通过 QLabel.clicked.connect(func)
方法连接到具体的函数。
import sys
from PyQt5 import QtWidgets
class Test(QtWidgets.QMainWindow):
def __init__(self):
QtWidgets.QMainWindow.__init__(self)
self.buttonA = QtWidgets.QPushButton("Click!", self)
self.buttonA.clicked.connect(self.clickCallback)
self.buttonA.move(100, 50)
self.labelA = QtWidgets.QLabel(self)
self.labelA.move(110, 100)
self.setGeometry(100, 100, 300, 200)
def clickCallback(self):
self.labelA.setText("Button is clicked")
if __name__ == "__main__":
app = QtWidgets.QApplication(sys.argv)
test = Test()
test.show()
sys.exit(app.exec_())
当 QPushButton
buttonA
被点击时,它触发 clickCallback
函数来设置标签文本是 Button is clicked
。