使用 Excel VBA 将数据写入文本文件
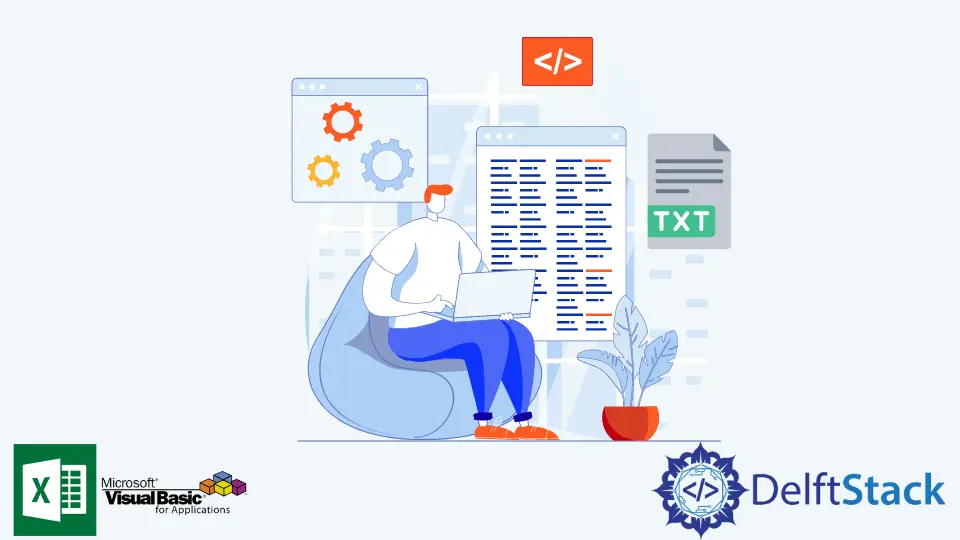
Excel VBA 在处理数据处理任务和清理时是一种流行的解决方案。此外,它可以自动执行需要花费大量时间才能完成的重复性任务。
VBA 的关键功能之一是它能够生成和编辑文本文件,而无需手动打开、命名和保存更改。
本文将演示如何在 VBA 的帮助下生成文本 (.txt)
文件。
在本文中,我们尝试使用 Excel VBA 生成文本文件。在深入研究代码之前,我们需要启用 Excel VBA 与处理文件和文件夹的 FileSystemObject
进行交互。
启用 FileSystemObject
的步骤:
-
打开 Excel 文件。
-
从
开发人员
选项卡,打开Visual Basic
编辑器。 -
从
工具
工具栏中,单击参考
。 -
勾选
Microsoft Scripting Runtime
复选框。
现在一切就绪。
下面的代码块将演示使用 VBA 创建文本文件。
SaveTextToFile
子程序需要两个参数,fileName
和 fileContent
。fileName
变量将是要生成的文本文件的所需文件名。fileContent
变量将是文本文件的实际内容。应该编辑 filePath
,使其适合输出文件的正确位置。
建议将 FileSystemObject
声明为 fso
以启用 Intellisense
的自动完成功能,这可以避免印刷错误,还有助于发现 FileSystemObject
库中的其他方法。
在代码执行结束之前包含 fso = Nothing
和 Set fileStream = Nothing
很重要,因为如果这两个未关闭,运行时将发生错误。
Option Explicit
Public Sub SaveTextToFile(fileName As String, fileContent As String)
Dim filePath, fileAttributes As String
filePath = "C:\Users\temp\Desktop\Destination"
fileAttributes = filePath & "\" & fileName & ".txt"
Dim fso As FileSystemObject
Set fso = New FileSystemObject
Dim fileStream As TextStream
Set fileStream = fso.CreateTextFile(fileAttributes)
fileStream.WriteLine fileContent
fileStream.Close
If fso.FileExists(fileAttributes) Then
Debug.Print "File Created Successfully."
End If
Set fileStream = Nothing
Set fso = Nothing
End Sub
Sub testSub()
Call SaveTextToFile("sample1", "Here is the content.")
End Sub
上面的代码块实际上并不与 Excel 交互,只生成一个文件。
下面的代码块将演示根据 Excel 文件中的数据创建文本文件。有关示例值,请参阅 Sheet1
。
工作表 1
:
| A | B | C |
1| File Name | Content | GenerateTextFile? |
2| sample1 | This is the content of sample1| Yes |
2| sample2 | This is the content of sample2| No |
2| sample3 | This is the content of sample3| Yes |
2| sample4 | This is the content of sample4| No |
2| sample5 | This is the content of sample5| Yes |
2| sample6 | This is the content of sample6| No |
在下面的示例中,我们将重用 SaveTextToFile
子例程来创建文本文件。testSub
子程序将根据 Sheet1
的数据循环调用 SaveTextToFile
。
Option Explicit
Public Sub SaveTextToFile(fileName As String, fileContent As String)
Dim filePath, fileAttributes As String
filePath = "C:\Users\temp\Desktop\Destination"
fileAttributes = filePath & "\" & fileName & ".txt"
Dim fso As FileSystemObject
Set fso = New FileSystemObject
Dim fileStream As TextStream
Set fileStream = fso.CreateTextFile(fileAttributes)
fileStream.WriteLine fileContent
fileStream.Close
If fso.FileExists(fileAttributes) Then
Debug.Print fileName & " Created Successfully."
End If
Set fileStream = Nothing
Set fso = Nothing
End Sub
Sub testSub()
Dim wb As Workbook
Dim s1 As Worksheet
Set wb = ThisWorkbook
Set s1 = wb.Sheets("Sheet1")
Dim i As Integer
i = 2
Do Until s1.Cells(i, 1) = ""
If s1.Cells(i, 3) = "Yes" Then
Call SaveTextToFile(s1.Cells(i, 1), s1.Cells(i, 2))
End If
i = i + 1
Loop
End Sub
testSub
输出:
sample1 Created Successfully.
sample3 Created Successfully.
sample5 Created Successfully.
Sample1
、Sample3
和 Sample5
是创建的文本文件,因为它们在 GenerateTextFile
列中有 Yes
。Sample2
和 Sample4
未创建,因为它们在 GenerateTextFile
列中为 No
。