在 React 中渲染 HTML 字符串
Rana Hasnain Khan
2024年2月15日
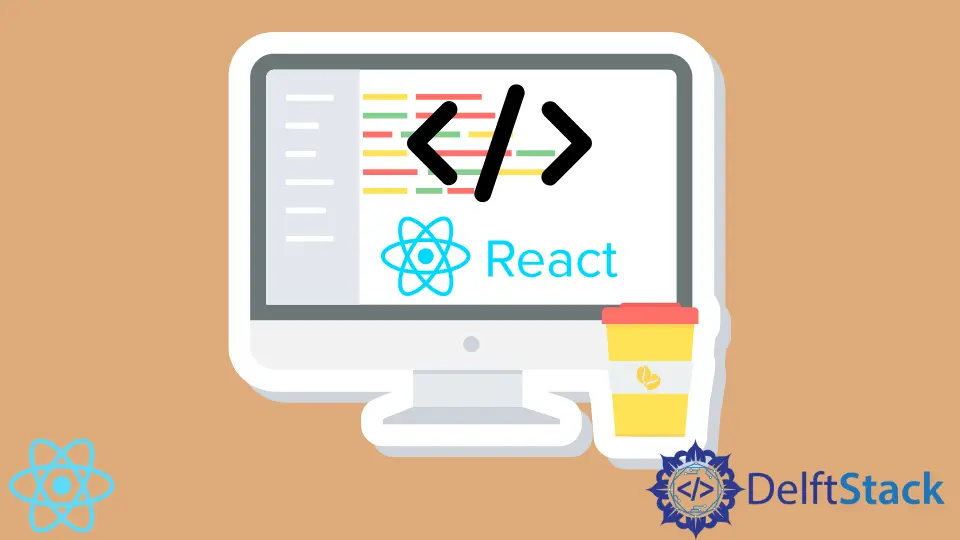
我们将介绍如何在 React 中渲染 HTML 字符串和渲染转义的 HTML 字符串。
在 React 中渲染 HTML 字符串
在大型网站上工作时,我们需要重用 HTML 组件。或者,当在 HTML 中处理 JSON 响应时,我们需要在 React 中呈现 HTML 字符串。
让我们举个例子,渲染一个普通的 HTML 字符串。首先,我们将创建一个 ID 为 root
的 div
。
# react
<div id="root"></div>
然后,让我们将 HTML 字符串呈现给 root
。
# react
class App extends React.Component {
constructor() {
super();
this.state = {
TextRender: '<h1>This is a heading</h1><p> This is a paragraph.</p>'
}
}
render() {
return (
<div dangerouslySetInnerHTML={{ __html: this.state.TextRender }} />
);
}
}
ReactDOM.render(<App />, document.getElementById('root'));
输出:
在上面的例子中,我们渲染了一个 HTML 字符串。但是如果我们尝试渲染一个转义的 HTML 字符串,它会报错。
让我们尝试渲染一个转义的 HTML 字符串。
# react
class App extends React.Component {
constructor() {
super();
this.state = {
TextRender: '<h1>This is a heading</h1><p> This is a paragraph.</p>'
}
}
render() {
return (
<div dangerouslySetInnerHTML={{ __html: this.state.TextRender }} />
);
}
}
ReactDOM.render(<App />, document.getElementById('root'));
输出:
正如你在上面的示例中注意到的那样,由于转义的 HTML 字符串,HTML 标记无法正确呈现。
现在,我们将创建一个 DecodeHtml
函数来将转义的 HTML 字符串转换为普通的 HTML 字符串。
# react
DecodeHtml(input){
var a = document.createElement('div');
a.innerHTML = input;
return a.childNodes.length === 0 ? "" : a.childNodes[0].nodeValue;
}
所以,代码应该是这样的。
# react
class App extends React.Component {
constructor() {
super();
this.state = {
TextRender: '<h1>This is a heading</h1><p> This is a paragraph.</p>'
}
}
htmlDecode(input){
var a = document.createElement('div');
a.innerHTML = input;
return a.childNodes.length === 0 ? "" : a.childNodes[0].nodeValue;
}
render() {
return (
<div dangerouslySetInnerHTML={{ __html: this.htmlDecode(this.state.TextRender) }} />
);
}
}
ReactDOM.render(<App />, document.getElementById('root'));
输出应如下所示。
输出:
通过这种方式,我们可以轻松渲染转义的 HTML 字符串。
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn