如何在 Python 中运行 Bash 脚本
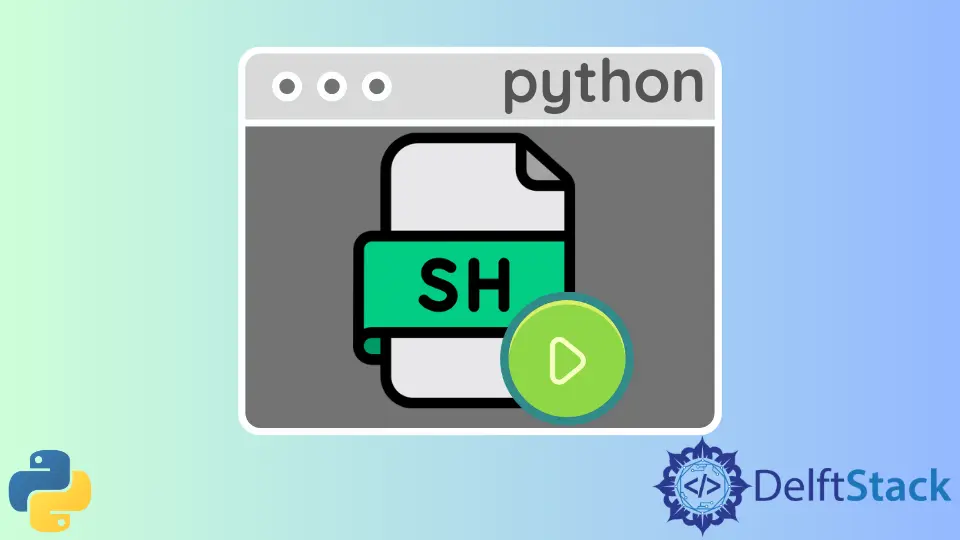
从 Python 执行 Bash 脚本可以显著增强你的自动化能力并简化你的工作流程。无论你是希望将系统命令集成到应用程序中的开发者,还是希望利用 shell 功能的数据科学家,理解如何使用 Python 执行 Bash 脚本是至关重要的。在本文中,我们将探讨通过 Python 执行 Bash 脚本的各种方法,包括使用 subprocess 模块、os.system 等。到本指南结束时,你将对如何在 Python 项目中无缝运行 Bash 命令和脚本有一个扎实的理解,从而能够充分利用两种语言的强大功能。
方法 1:使用 subprocess 模块
subprocess
模块是 Python 中的一个强大工具,允许你生成新的进程,连接到它们的输入/输出/错误管道,并获取它们的返回码。这个方法被推荐用于执行 Bash 脚本,因为它提供比其他方法更多的控制和灵活性。
下面是一个如何使用 subprocess
模块执行 Bash 脚本的简单示例:
import subprocess
# Define the command to execute
command = ["bash", "your_script.sh"]
# Execute the command
process = subprocess.run(command, capture_output=True, text=True)
# Print the output and error (if any)
print("Output:")
print(process.stdout)
print("Error:")
print(process.stderr)
输出:
example of output
subprocess.run
函数执行指定的命令并等待其完成。capture_output=True
参数允许你捕获输出和错误消息,这些信息可以通过返回的进程对象的 stdout
和 stderr
属性访问。这个方法的优点在于它允许你通过检查 stderr
输出来优雅地处理错误。此外,使用列表来定义命令有助于避免 shell 注入漏洞。
方法 2:使用 os.system
os.system
函数是从 Python 执行 Bash 脚本的另一种简单方法。虽然它比 subprocess
更简单易用,但灵活性较低,并且不提供对输出或错误消息的直接访问。
以下是如何使用 os.system
运行 Bash 脚本:
import os
# Define the command to execute
command = "bash your_script.sh"
# Execute the command
exit_code = os.system(command)
# Print the exit code
print("Exit Code:", exit_code)
输出:
example of output
os.system
函数在子 shell 中执行命令。它返回命令的退出码,你可以用它来确定脚本是否成功运行。然而,这种方法的一个限制是你无法直接捕获执行脚本的输出,这可能对更复杂的任务来说是个缺点。如果你需要处理输出或错误,建议使用 subprocess
。
方法 3:使用 subprocess.Popen
对于更高级的用例,subprocess.Popen
类提供了对 Bash 脚本执行更大的控制。这个方法允许你在进程运行时进行交互,适合于长时间运行的脚本或那些需要实时输入的脚本。
下面是使用 subprocess.Popen
执行 Bash 脚本的示例:
import subprocess
# Define the command to execute
command = ["bash", "your_script.sh"]
# Open the process
process = subprocess.Popen(command, stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
# Read output and error
stdout, stderr = process.communicate()
# Print the output and error
print("Output:")
print(stdout)
print("Error:")
print(stderr)
输出:
example of output
在这种方法中,subprocess.Popen
启动进程,并允许你通过管道与之进行交互。communicate()
方法在进程完成后读取输出和错误消息。这种方法对于长时间运行的脚本特别有用,因为它允许你实时处理输出。此外,如果需要,你可以向脚本提供输入,使得这个方法非常灵活。
结论
总之,使用 Python 执行 Bash 脚本可以显著增强你的编程能力,允许更高的自动化和效率。讨论的三种方法——使用 subprocess
模块、os.system
和 subprocess.Popen
——各自具有独特的优点和使用场景。根据你的需求,可以选择最适合你的项目的方法。通过掌握这些技术,你可以轻松地将 Bash 脚本集成到 Python 应用程序中,开启自动化和系统管理的新可能性。
常见问题
-
从 Python 执行 Bash 脚本的最佳方法是什么?
最佳方法取决于你的具体需求。在大多数情况下,建议使用subprocess
模块,因为它的灵活性和错误处理能力更强。 -
我可以在 Python 中捕获 Bash 脚本的输出吗?
可以,你可以通过设置capture_output=True
或使用subprocess.PIPE
与Popen
来捕获输出。 -
从 Python 执行 Bash 脚本是否安全?
是的,但要小心 shell 注入漏洞。当使用subprocess
时,将命令作为列表传递而不是单个字符串会更安全。 -
我可以从 Python 向 Bash 脚本传递参数吗?
可以,你可以在使用subprocess
或os.system
时将参数包括在命令列表中。 -
如果我的 Bash 脚本需要用户输入怎么办?
你可以使用subprocess.Popen
与进程进行交互,并根据需要提供输入。