如何使用 Python 逐行写入文件
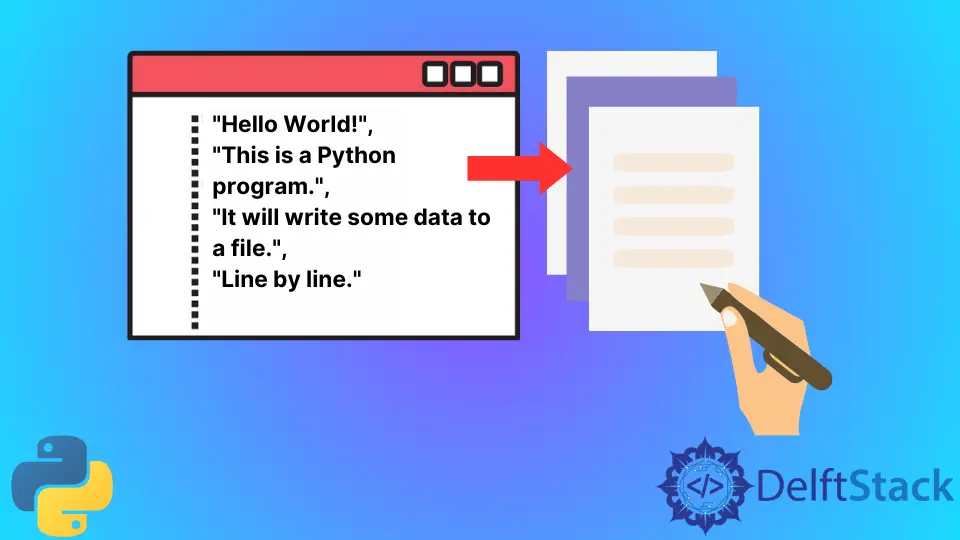
使用 Python,你可以完成的许多任务之一是逐行写入文件。本文将引导你了解实现此任务的主要方法,并配有清晰、注释良好的 Python 代码示例和详细解释。
在我们深入了解如何做之前,让我们先了解什么和为什么。在 Python 中逐行写入文件的任务听起来复杂,但实际上,由于 Python 的内置函数,这个任务非常简单。当你想要存储 Python 程序的输出以供后续使用或分析时,或当你处理需要逐行处理以节省内存的大数据集时,这个任务是至关重要的。
方法 1:使用 write()
在 Python 中逐行写入文件的第一个也是最简单的方法是使用 write()
函数。此函数允许你向文件添加单行。
# First, open the file in write mode
file = open('example.txt', 'w')
# Then, write a line to the file
file.write('This is an example line.\n')
# Always remember to close the file when you're done
file.close()
在此示例中,字符串末尾的 \n
创建了一个新行,因此下次你使用 write()
时,新文本将在新行上写入。完成写入后,记得使用 file.close()
关闭文件。这确保你所做的更改已保存,并释放资源。
方法 2:使用 writelines()
在 Python 中逐行写入文件的另一个方法是使用 writelines()
函数。当你有一个要写入文件的行列表时,此函数特别有用。
# Open the file in write mode
file = open('example.txt', 'w')
# Create a list of lines to write to the file
lines = ['First line.\n', 'Second line.\n', 'Third line.\n']
# Write the lines to the file
file.writelines(lines)
# Close the file
file.close()
在此示例中,列表 lines
中的每个字符串表示文件中的一行。writelines()
函数逐行将每个字符串写入文件。
方法 3:使用 with open
Python 中的 with open
语句是简化逐行写入文件过程的绝佳方法。完成后,它会自动关闭文件,因此你不需要记得调用 file.close()
。
# Open the file in write mode
with open('example.txt', 'w') as file:
# Write a line to the file
file.write('This is an example line.\n')
在此示例中,with open
语句打开文件,后续缩进的代码行将写入文件。一旦这些代码行被执行,文件将被自动关闭。
结论
在 Python 中逐行写入文件是一个简单的任务,Python 的内置函数使其变得简单。无论你选择使用 write()
、writelines()
还是 with open
语句,你都有必要的工具来存储程序的输出或逐行处理大型数据集。
常见问题
-
open()
函数中的 ‘w’ 代表什么?
‘w’ 代表 ‘write’(写入)。它以写入模式打开文件,这允许你向文件添加文本。 -
如果我忘了关闭文件会发生什么?
如果文件未关闭,则更改可能不会保存,并且资源未释放。这可能导致内存问题,尤其是在处理大文件时。 -
我可以使用
write()
函数一次写入多行吗?
是的,你可以通过在字符串中包含\n
来一次写入多行以创建新行。然而,对于行列表,writelines()
函数更合适。