在 Python 中计算百分位数
-
在 Python 中使用
scipy
包计算百分位数 -
在 Python 中使用
NumPy
包计算百分位数 -
在 Python 中使用
math
包计算百分位数 -
在 Python 中使用
statistics
包计算百分位数 - 在 Python 中使用 NumPy 的线性插值方法计算百分位数
- 在 Python 中使用 NumPy 的下插值方法计算百分位数
- 在 Python 中使用 NumPy 的高级插值方法计算百分位数
- 在 Python 中使用 NumPy 的中点插值方法计算百分位数
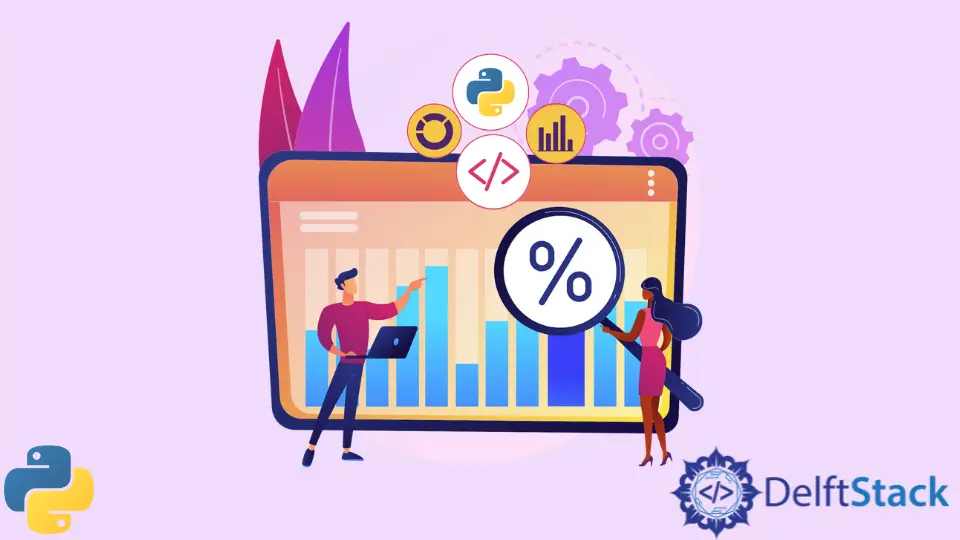
百分位数表示低于某一数值的分数的百分比。例如,一个人的智商为 120,就处于第 91 个百分位数,这意味着他的智商大于 91%的其他人。
本文将讨论一些在 Python 中计算百分位数的方法。
在 Python 中使用 scipy
包计算百分位数
这个包将计算输入序列在给定百分位的得分。scoreatpercentile()
函数的语法如下。
scipy.stats.scoreatpercentile(
a, per, limit=(), interpolation_method="fraction", axis=None
)
在 scoreatpercentile()
函数中,参数 a
代表一维数组,per
指定 0 到 100 的百分位数。另外两个参数是可选的。NumPy
库用来获取我们计算百分位数的数字。
完整的示例代码如下。
from scipy import stats
import numpy as np
array = np.arange(100)
percentile = stats.scoreatpercentile(array, 50)
print("The percentile is:", percentile)
输出:
The percentile is: 49.5
在 Python 中使用 NumPy
包计算百分位数
这个包有一个 percentile()
函数,可以计算给定数组的百分位数。percentile()
函数的语法如下。
numpy.percentile(
a,
q,
axis=None,
out=None,
overwrite_input=False,
interpolation="linear",
keepdims=False,
)
参数 q
代表百分位数的计算数。a
代表一个数组,其他参数是可选的。
完整的示例代码如下。
import numpy as np
arry = np.array([4, 6, 8, 10, 12])
percentile = np.percentile(arry, 50)
print("The percentile is:", percentile)
输出:
The percentile is: 8.0
在 Python 中使用 math
包计算百分位数
math
包及其基本函数-ceil
可用于计算不同的百分比。
完整的示例代码如下。
import math
arry = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
def calculate_percentile(arry, percentile):
size = len(arry)
return sorted(arry)[int(math.ceil((size * percentile) / 100)) - 1]
percentile_25 = calculate_percentile(arry, 25)
percentile_50 = calculate_percentile(arry, 50)
percentile_75 = calculate_percentile(arry, 75)
print("The 25th percentile is:", percentile_25)
print("The 50th percentile is:", percentile_50)
print("The 75th percentile is:", percentile_75)
math.ceil(x)
将数值四舍五入并返回大于或等于 x
的最小整数,而 sorted
函数则对数组进行排序。
输出:
The 25th percentile is: 3
The 50th percentile is: 5
The 75th percentile is: 8
在 Python 中使用 statistics
包计算百分位数
statistics
包中的 quantiles()
函数用于将数据分解为等概率,并返回 n-1
的分布列表。该函数的语法如下。
statistics.quantiles(data, *, n=4, method='exclusive')
完整的示例代码如下。
from statistics import quantiles
data = [1, 2, 3, 4, 5]
percentle = quantiles(data, n=4)
print("The Percentile is:", percentle)
输出:
The Percentile is: [1.5, 3.0, 4.5]
在 Python 中使用 NumPy 的线性插值方法计算百分位数
我们可以使用插值模式计算不同的百分比。插值模式有 linear
、lower
、higher
、midpoint
和 nearest
。当百分位数在两个数据点 i
和 j
之间时,会使用这些插值。当百分位值为 i
时,为低位插值模式,j
代表高位插值模式,i + (j - i) * fraction
代表线性模式,其中 fraction
表示 i
和 j
包围的索引。
下面给出了线性插值模式的完整示例代码。
import numpy as np
arry = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
print("percentiles using interpolation = ", "linear")
percentile_10 = np.percentile(arry, 10, interpolation="linear")
percentile_50 = np.percentile(arry, 50, interpolation="linear")
percentile_75 = np.percentile(arry, 75, interpolation="linear")
print(
"percentile_10 = ",
percentile_10,
", median = ",
percentile_50,
" and percentile_75 = ",
percentile_75,
)
我们使用 numpy.percentile()
函数,附加参数 interpolation
。你可以看到,我们得到的是这种插值的浮动值。
输出:
percentiles using interpolation = linear
percentile_10 = 1.9 , median = 5.5 and percentile_75 = 7.75
在 Python 中使用 NumPy 的下插值方法计算百分位数
下面给出了较低插值模式的完整示例代码。
import numpy as np
arry = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
print("percentiles using interpolation = ", "lower")
percentile_10 = np.percentile(arry, 10, interpolation="lower")
percentile_50 = np.percentile(arry, 50, interpolation="lower")
percentile_75 = np.percentile(arry, 75, interpolation="lower")
print(
"percentile_10 = ",
percentile_10,
", median = ",
percentile_50,
" and percentile_75 = ",
percentile_75,
)
输出:
percentiles using interpolation = lower
percentile_10 = 1 , median = 5 and percentile_75 = 7
你可以看到,最终的百分位数被粗略地调整到了最低值。
在 Python 中使用 NumPy 的高级插值方法计算百分位数
此方法将给出给定数组的百分数,取最高舍入值。
下面给出了高插值模式的完整示例代码。
import numpy as np
arry = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
print("percentiles using interpolation = ", "higher")
percentile_10 = np.percentile(arry, 10, interpolation="higher")
percentile_50 = np.percentile(arry, 50, interpolation="higher")
percentile_75 = np.percentile(arry, 75, interpolation="higher")
print(
"percentile_10 = ",
percentile_10,
", median = ",
percentile_50,
" and percentile_75 = ",
percentile_75,
)
输出:
percentiles using interpolation = higher
percentile_10 = 2 , median = 6 and percentile_75 = 8
在 Python 中使用 NumPy 的中点插值方法计算百分位数
此方法将给出百分位数的中点。
下面给出了中点插值模式的完整示例代码。
import numpy as np
arry = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
print("percentiles using interpolation = ", "midpoint")
percentile_10 = np.percentile(arry, 10, interpolation="midpoint")
percentile_50 = np.percentile(arry, 50, interpolation="midpoint")
percentile_75 = np.percentile(arry, 75, interpolation="midpoint")
print(
"percentile_10 = ",
percentile_10,
", median = ",
percentile_50,
" and percentile_75 = ",
percentile_75,
)
输出:
percentiles using interpolation = midpoint
percentile_10 = 1.5 , median = 5.5 and percentile_75 = 7.5
相关文章 - Python Math
- 在 Python 中计算阶乘
- 在 Python 中计算反余弦
- 在 Python 中计算模乘逆
- 使用基本编程概念在 Python 中打印乘法表
- 用 Python 制作成绩转换器
- Python 中的级数求和