使用基本编程概念在 Python 中打印乘法表
Jesse John
2023年1月30日
Python
Python Math
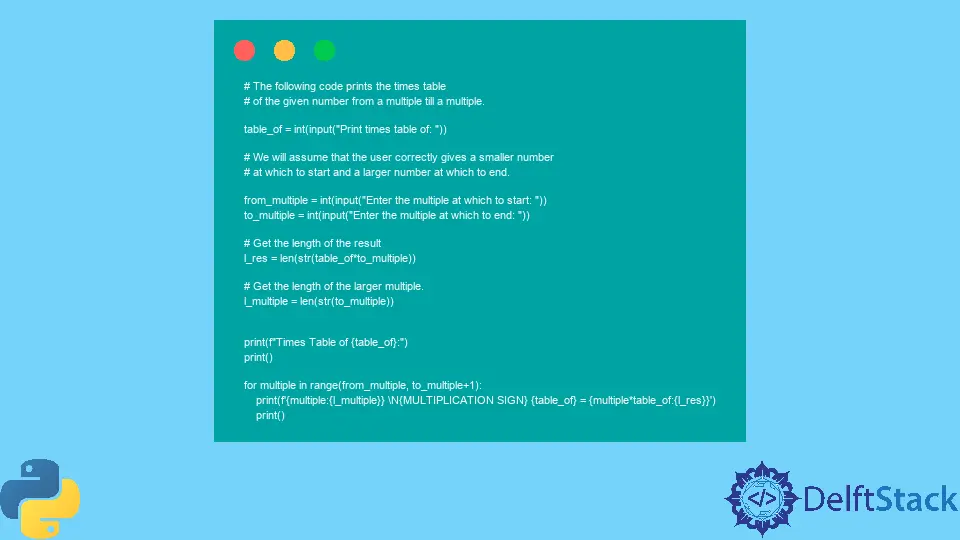
我们可以通过学习在 Python 中打印时间表来练习几个基本的编程概念。这些包括:
- 使用变量
- 获取用户输入
- 使用内置函数
- 类型转换变量
- 迭代(循环)
- 字符串格式化
- 使用 Unicode 符号
我们将使用 Python 的 f
字符串格式化功能,可用于 Python 3.6 及更高版本。
基本编程概念
我们可以声明一个变量并为其赋值,如下所示。
table_of = 5
我们将使用 input()
函数来获取用户输入,如下所示。
table_of = input("Print times table of: ")
程序将显示字符串 Print times table of:
并等待用户输入。用户可以输入任何内容。Python 将输入解释为字符串。
要将其转换为整数,我们将在 input()
函数周围使用 int()
函数。
table_of = int(input("Print times table of: "))
print("Times")
在显示屏上打印单词 Times
。一个空的 print()
函数打印一个空行。
range()
函数创建从 start_int
到但不包括 end_int
的序列。默认情况下,它增加 1。
range(start_int, end_int, step_int)
我们将在代码中使用 for
循环。只要变量在指定范围内,它就会重复循环中的代码多次。
for variable in range(start, end):
code to repeat
Python 的 f
字符串格式化功能允许我们使用占位符 {}
在字符串中包含变量。要使用变量 table_of
的值,我们将使用:
print(f"Times table of {table_of}")
我们可以使用整数指定占位符的长度。在代码中,我们使用另一个变量来指定这一点:结果 table_of * 9
的长度。
我们使用 str()
将整数转换为字符串以获得长度。
乘法符号使用其 Unicode 名称指定。
\N{MULTIPLICATION SIGN}
在 Python 中打印给定数字的时间表
我们现在将把上述所有概念放在下面的代码中。它将以两种方式打印用户给定数字的乘法表。
示例代码:
# The following code prints the times table
# of the given number till 'number x 9'.
# It prints the times table in two different ways.
table_of = int(input("Print times table of: "))
# Get the length of the result
l_res = len(str(table_of * 9))
print(f"Times Table of {table_of}:")
print()
for multiple in range(1, 10):
print(
f"{multiple} \N{MULTIPLICATION SIGN} {table_of} = {table_of*multiple:{l_res}}"
)
print()
print("-------------")
print()
for multiple in range(1, 10):
print(
f"{table_of} \N{MULTIPLICATION SIGN} {multiple} = {table_of*multiple:{l_res}}"
)
print()
样本输出:
Print times table of: 1717
Times Table of 1717:
1 × 1717 = 1717
2 × 1717 = 3434
3 × 1717 = 5151
4 × 1717 = 6868
5 × 1717 = 8585
6 × 1717 = 10302
7 × 1717 = 12019
8 × 1717 = 13736
9 × 1717 = 15453
-------------
1717 × 1 = 1717
1717 × 2 = 3434
1717 × 3 = 5151
1717 × 4 = 6868
1717 × 5 = 8585
1717 × 6 = 10302
1717 × 7 = 12019
1717 × 8 = 13736
1717 × 9 = 15453
作为一种变体,我们可以从给定数字的所需倍数打印乘法表。
示例代码:
# The following code prints the times table
# of the given number from a multiple till a multiple.
table_of = int(input("Print times table of: "))
# We will assume that the user correctly gives a smaller number
# at which to start and a larger number at which to end.
from_multiple = int(input("Enter the multiple at which to start: "))
to_multiple = int(input("Enter the multiple at which to end: "))
# Get the length of the result
l_res = len(str(table_of * to_multiple))
# Get the length of the larger multiple.
l_multiple = len(str(to_multiple))
print(f"Times Table of {table_of}:")
print()
for multiple in range(from_multiple, to_multiple + 1):
print(
f"{multiple:{l_multiple}} \N{MULTIPLICATION SIGN} {table_of} = {multiple*table_of:{l_res}}"
)
print()
样本输出:
Print times table of: 16
Enter the multiple at which to start: 5
Enter the multiple at which to end: 15
Times Table of 16:
5 × 16 = 80
6 × 16 = 96
7 × 16 = 112
8 × 16 = 128
9 × 16 = 144
10 × 16 = 160
11 × 16 = 176
12 × 16 = 192
13 × 16 = 208
14 × 16 = 224
15 × 16 = 240
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jesse John