OpenCV 斑点或圆形检测
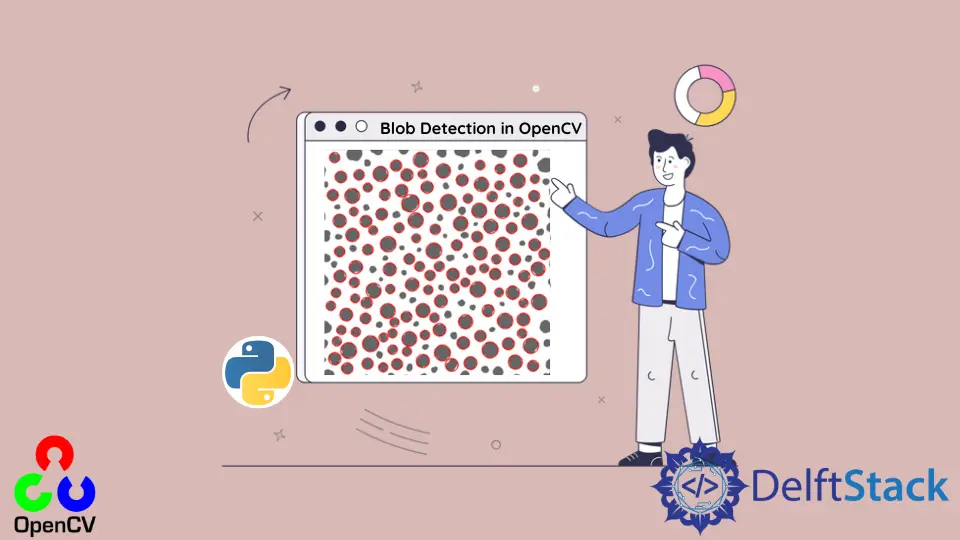
本教程将讨论在 Python 中使用 OpenCV 的 SimpleBlobDetector
类检测图像中的斑点或圆圈。
在 Python 中使用 OpenCV 的 SimpleBlobDetector
类检测图像中的斑点或圆圈
我们可以使用 OpenCV 的 SimpleBlobDetector
类检测图像中的斑点或圆圈。我们可以使用面积、圆度、凸度和惯性来检测斑点或圆。
如果我们想检测落在特定区域内的斑点或圆圈,我们必须将 filterByArea
参数设置为 true。然后我们可以使用 minArea
参数设置 blob 的最小面积,使用 maxArea
参数设置 blob 的最大面积。
它不会检测小于或大于指定区域的斑点。我们可以使用 SimpleBlobDetector
类的 Params()
方法来改变我们想要检测的形状。
我们可以使用 OpenCV 的 drawKeypoints()
函数来突出显示该函数检测到的斑点。
例如,让我们根据斑点区域检测给定图像中的一些斑点。请参阅下面的代码。
import cv2
import numpy as np
image = cv2.imread("blob2.jpg", 0)
params = cv2.SimpleBlobDetector_Params()
params.filterByArea = True
params.minArea = 100
detector = cv2.SimpleBlobDetector_create(params)
keypoints = detector.detect(image)
blank = np.zeros((1, 1))
blobs = cv2.drawKeypoints(
image, keypoints, blank, (0, 0, 255), cv2.DRAW_MATCHES_FLAGS_DRAW_RICH_KEYPOINTS
)
cv2.imshow("Blobs Using Area", blobs)
cv2.waitKey(0)
cv2.destroyAllWindows()
输出:
在 Python 中设置 filterByCircularity
以过滤图像中的斑点或圆圈
我们还可以使用它的圆形度来检测一个斑点,这将检查该形状的圆形程度。圆形度为 1 表示圆,0.78 表示长方体。
如果我们只想检测圆形斑点,我们必须将 filterByCircularity
参数设置为 True。然后我们可以使用 minCircularity
和 maxCircularity
来设置形状的最小和最大圆度值。
它不会检测圆形度大于或小于指定值的斑点。例如,让我们在上面的例子中设置循环。请参阅下面的代码。
import cv2
import numpy as np
image = cv2.imread("blob2.jpg", 0)
params = cv2.SimpleBlobDetector_Params()
params.filterByArea = True
params.minArea = 100
params.filterByCircularity = True
params.minCircularity = 0.9
detector = cv2.SimpleBlobDetector_create(params)
keypoints = detector.detect(image)
blank = np.zeros((1, 1))
blobs = cv2.drawKeypoints(
image, keypoints, blank, (0, 0, 255), cv2.DRAW_MATCHES_FLAGS_DRAW_RICH_KEYPOINTS
)
cv2.imshow("Using area,circularity", blobs)
cv2.waitKey(0)
cv2.destroyAllWindows()
输出:
如你所见,在上述输出中仅检测到圆形斑点。
在 Python 中设置 filterByConvexity
以过滤图像中的斑点或圆圈
我们还可以使用凸度过滤 blob。首先,我们必须将 filterByConvexity
参数设置为 true,然后我们可以使用 minConvexity
和 maxConvexity
来设置凸度的最小值和最大值。
一个椭圆也是一个圆,也会和一个正圆一起被检测到。我们可以使用 filterInertia
参数来使用形状的惯性过滤 blob。
对于正圆,惯性为 1,对于椭圆,惯性在 0 到 1 之间。我们可以使用 minInertiaRatio
和 maxInertiaRatio
来设置惯性的最小值和最大值。
我们还可以使用 OpenCV 的 putText()
函数显示图像上的斑点数量。
例如,让我们设置凸度和惯性的值,并显示在给定图像顶部检测到的斑点数量。请参阅下面的代码。
import cv2
import numpy as np
image = cv2.imread("blob2.jpg", 0)
params = cv2.SimpleBlobDetector_Params()
params.filterByArea = True
params.minArea = 100
params.filterByCircularity = True
params.minCircularity = 0.9
params.filterByConvexity = True
params.minConvexity = 0.2
params.filterByInertia = True
params.minInertiaRatio = 0.01
detector = cv2.SimpleBlobDetector_create(params)
keypoints = detector.detect(image)
blank = np.zeros((1, 1))
blobs = cv2.drawKeypoints(
image, keypoints, blank, (0, 0, 255), cv2.DRAW_MATCHES_FLAGS_DRAW_RICH_KEYPOINTS
)
number_of_blobs = len(keypoints)
text = "Circular Blobs: " + str(len(keypoints))
cv2.putText(blobs, text, (10, 100), cv2.FONT_HERSHEY_SIMPLEX, 0.8, (0, 0, 255), 2)
cv2.imshow("Original Image", image)
cv2.imshow("Circular Blobs Only", blobs)
cv2.waitKey(0)
cv2.destroyAllWindows()
输出:
我们还可以设置文本的位置、字体系列、字体比例、颜色和线条粗细。我们还在左侧显示了原始图像以进行比较。
我们还可以通过将 filterByColor
参数设置为 true 来根据它们的颜色过滤 blob。然后我们可以使用 minThreshold
和 maxThreshold
来设置我们想要检测的颜色的这些最小和最大阈值。
它不会检测颜色值小于或大于指定阈值的 blob。我们还可以通过更改 SimpleBlobDetector
类的参数来检测其他形状。
例如,我们在上面的示例中检测到圆形斑点,但我们也可以检测其他形状的斑点,例如矩形斑点。
单击此链接了解有关 SimpleBlobDetector
类的参数的更多详细信息。
相关文章 - Python OpenCV
- OpenCV sobel() 函数
- Python 中 OpenCV 的 GUI 特性
- 在 Python 中使用 OpenCV 创建二维码扫描器
- 在 Python 中使用 OpenCV 的 HSV 颜色空间
- OpenCV 中的模糊滤镜
- 使用 OpenCV 进行对象跟踪