在 Python 中合并 CSV 文件
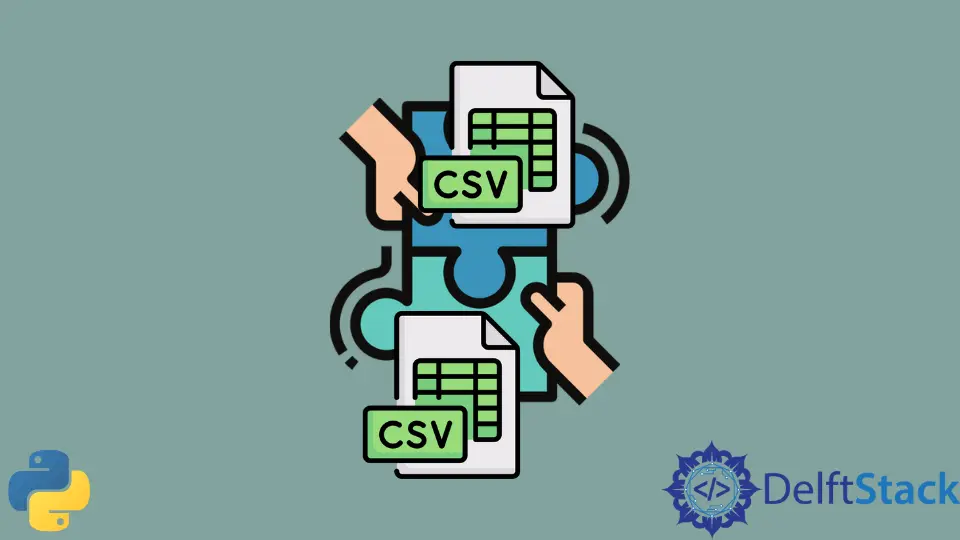
在 Pandas DataFrame
中处理 .csv 文件形式的大型数据集时,单个文件可能不包含用于数据分析的完整信息。在这种情况下,我们需要在单个 Pandas DataFrame
中合并多个文件。Python pandas 库提供了各种方法来解决这个问题,例如 concat
、merge
和 join
。
在本指南中,我们将学习两种不同的方法,借助不同的示例将多个 .csv 文件合并到一个 Pandas DataFrame
中。
使用按名称合并将多个 CSV 文件合并到单个 Pandas DataFrame
中
要合并多个 .csv 文件,首先,我们导入 pandas 库并设置文件路径。然后,使用 pd.read_csv()
方法读取所有 CSV 文件。pd.concat()
将映射的 CSV 文件作为参数,然后默认沿行轴合并它们。ignore_index=True
参数用于为新合并的 DataFrame
设置连续索引值。
请参阅以下示例,我们使用 pandas python 实现了上述方法:
示例代码:
import pandas as pd
# set files path
sales1 = "C:\\Users\\DELL\\OneDrive\\Desktop\\salesdata1.csv"
sales2 = "C:\\Users\DELL\\OneDrive\\Desktop\\salesdata2.csv"
print("*** Merging multiple csv files into a single pandas dataframe ***")
# merge files
dataFrame = pd.concat(map(pd.read_csv, [sales1, sales2]), ignore_index=True)
print(dataFrame)
输出:
*** Merging multiple csv files into a single pandas dataframe ***
Product_Name Quantity Sale_Price
0 Acer laptop 3 500$
1 Dell Laptop 6 700$
2 Hp laptop 8 800$
3 Lenavo laptop 2 600$
4 Acer laptop 3 500$
5 Dell Laptop 6 700$
6 Hp laptop 8 800$
7 Lenavo laptop 2 600$
通过合并所有字段在单个 Pandas DataFrame
中合并多个 CSV 文件
为了合并 Pandas DataFrame
中的所有 .csv 文件,我们在这种方法中使用了 glob 模块。首先,我们必须导入所有库。之后,我们为所有需要合并的文件设置路径。
在以下示例中,os.path.join()
将文件路径作为第一个参数,将要连接的路径组件或 .csv 文件作为第二个参数。在这里,salesdata*.csv
将匹配并返回指定主目录中以 salesdata
开头并以 .csv 扩展名结尾的每个文件。glob.glob(files_joined)
接受合并文件名的参数并返回所有合并文件的列表。
请参阅以下示例以使用 glob 模块合并所有 .csv 文件:
示例代码:
import pandas as pd
import glob
import os
# merging the files
files_joined = os.path.join(
"C:\\Users\\DELL\\OneDrive\\Desktop\\CSV_files", "salesdata*.csv"
)
# Return a list of all joined files
list_files = glob.glob(files_joined)
print("** Merging multiple csv files into a single pandas dataframe **")
# Merge files by joining all files
dataframe = pd.concat(map(pd.read_csv, list_files), ignore_index=True)
print(dataframe)
输出:
** Merging multiple csv files into a single pandas dataframe **
Product_Name Quantity Sale_Price
0 Acer laptop 3 500$
1 Dell Laptop 6 700$
2 Hp laptop 8 800$
3 Lenavo laptop 2 600$
4 Acer laptop 3 500$
5 Dell Laptop 6 700$
6 Hp laptop 8 800$
7 Lenavo laptop 2 600$
结论
我们在本教程中介绍了两种在 pandas python 中合并多个 CSV 文件的方法。我们已经看到了如何使用 pd.concat()
方法读取 .csv 文件并将它们合并到单个 Pandas DataFrame
中。此外,我们现在知道如何在 Pandas python 代码中使用 glob
模块。