Python 中如何将字节 bytes 转换为整数 int
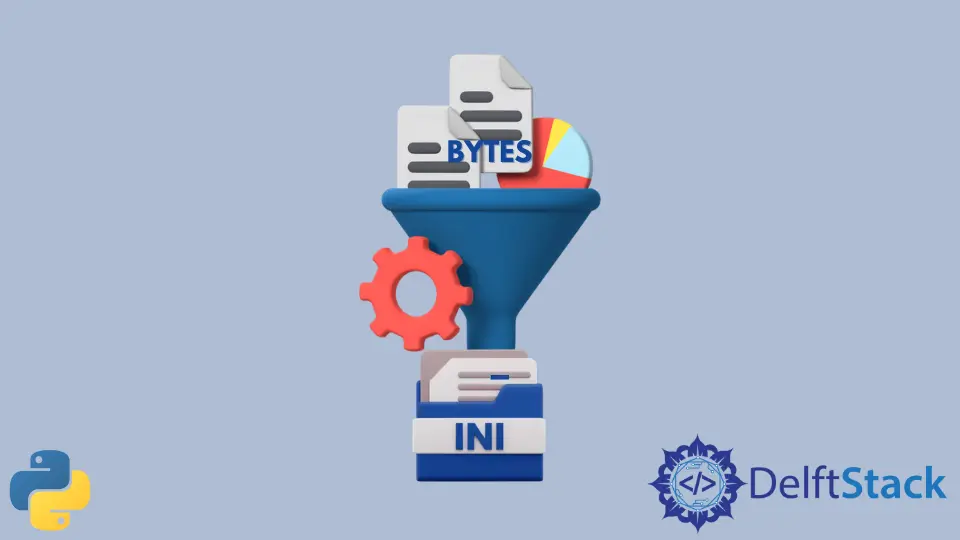
Bytes
数据类型的数值范围为 0~255(0x00~0xFF)。一个字节有 8 位数据,这就是为什么它的最大值是 0xFF。在某些情况下,你需要将字节或字节数组转换为整数以进行进一步的数据处理。我们就来看如何进行字节 Bytes
到整数 integer
的转换。
Python 2.7 中的字节 Bytes
Python 2.7 版本中没有内置的 bytes
数据类型。关键字 byte
与 str
是等同的。我们可以通过下面的 is
判断的高处这个结论。
>>> bytes is str
True
bytearray
用于定义一个 bytes
或一个 byte array
对象。
>>> byteExample1 = bytearray([1])
>>> byteExample1
bytearray(b'\x01')
>>> byteExample2 = bytearray([1,2,3])
>>> byteExample2
bytearray(b'\x01\x02\x03')
Python 2.7 中将字节转换为整数
Python 内部模块 struct
可以将二进制数据(字节)转换为整数。它可以双向转换 Python 2.7 中的字节(实际上是字符串)和整数。下面就是它的一个基本介绍,
struct.unpack(fmt, string)
# Convert the string according to the given format `fmt` to integers. The result is a tuple even if there is only one item inside.
struct
例子
import struct
testBytes = b"\x00\x01\x00\x02"
testResult = struct.unpack(">HH", testBytes)
print testResult
(1, 2)
格式字符串 >HH
包含两部分,
>
表示二进制数据是big-endian
,或换句话说,数据是从高端(最高有效位)排序的。例如,\x00\0x1
中,\x00
是高字节,\x01
是低字节。HH
表示字节字符串中有两个H
类型的对象。H
表示它是unsigned short
整数,占用了 2 个字节。
如果分配的数据格式不同,你可以从相同的字符串中得到不同的结果。
>>> testResult = struct.unpack('<HH', testBytes)
>>> testResult
(256, 512)
这里,<
表示字节顺序是 little-endian
。因此\x00\x01
变成 00+1*256 = 256
不是 0*256+1 = 1
了。
>>> testResult = struct.unpack('<BBBB', testBytes)
>>> testResult
(0, 1, 0, 2)
B
表示数据类型是 unsigned char
,它占用了 1 个字节。因此,\x00\x01\x00\x02
将被转换为 4 个 unsigned char
的值,而不再是 2 个 unsigned short
的值。
>>> testResult = struct.unpack('<BBB', b'\x00\x01\x00\x02')
Traceback (most recent call last):
File "<pyshell#35>", line 1, in <module>
testResult = struct.unpack('<BBB', b'\x00\x01\x00\x02')
error: unpack requires a string argument of length 3
你可以查看 struct
模块的官方文档来获取格式字符串的更多信息。
Python 3 中的字节 Bytes
bytes
是 Python 3 中的内置数据类型,因此,你可以使用 bytes
关键字直接来定义字节。
>>> testByte = bytes(18)
>>> type(testByte)
<class 'bytes'>
你也可以像下面这样直接定义一个或多个字节。
>>> testBytes = b'\x01\x21\31\41'
>>> type(testBytes)
<class 'bytes'>
Python 3 中将字节转换为整数
除了已经在 Python 2.7 中引入的 struct
模块之外,你还可以使用新的 Python 3 内置整数方法来执行字节到整数的转换,即 int.from_bytes()
方法。
int.from_bytes()
例子
>>> testBytes = b'\xF1\x10'
>>> int.from_bytes(testBytes, byteorder='big')
61712
该 byteorder
选项类似于 struct.unpack()
字节顺序格式的定义。
The byteorder
option is similar to struct.unpack()
format byte order definition.
int.from_bytes()
有第三个选项 signed
,它将整数类型赋值为 signed
或 unsigned
。
>>> testBytes = b'\xF1\x10'
>>> int.from_bytes(testBytes, byteorder='big', signed=True)
-3824
当字节是 unsigned chart
时使用 []
如果数据格式是 unsigned char
且只包含一个字节,则可以直接使用对象索引进行访问来获取该整数。
>>> testBytes = b'\xF1\x10'
>>> testBytes[0]
241
>>> testBytes[1]
16