如何按一列的值对 Pandas DataFrame 进行排序
Asad Riaz
2023年1月30日
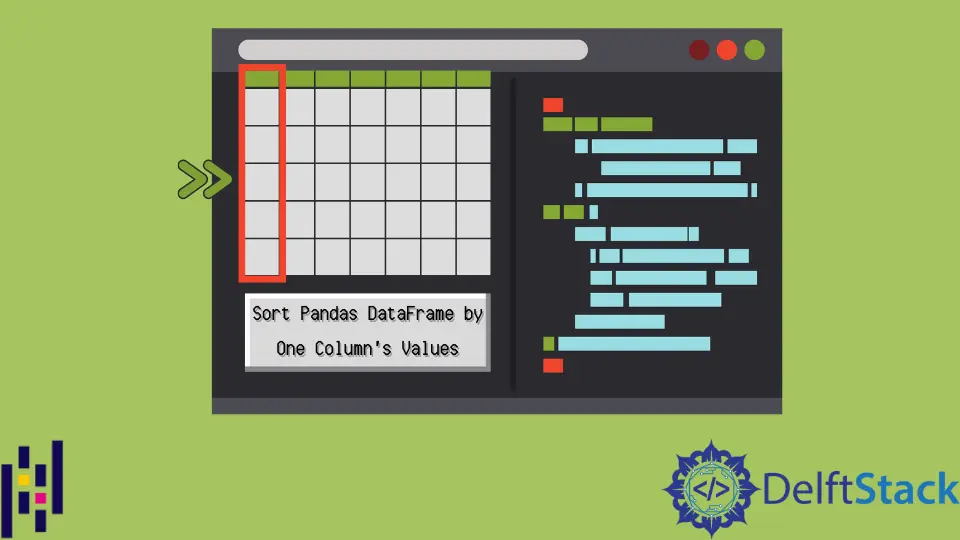
我们将介绍 pandas.DataFrame.sort_values
方法来对 DataFrame
值进行排序,以及类似 ascending
选项来指定排序顺序,以及 na_position
来确定 NaN 在排序结果中的位置。
参考下面的 DataFrame
,
import pandas as pd
df = pd.DataFrame(
{
"col1": ["g", "t", "n", "w", "n", "g"],
"col2": [5, 2, 5, 1, 3, 6],
"col3": [0, 7, 2, 8, 1, 2],
}
)
print(df)
如果运行此代码,你将得到以下尚未排序的输出。
col1 col2 col3
0 g 5 0
1 t 2 7
2 n 5 2
3 w 1 8
4 n 3 1
5 g 6 2
现在我们可以使用以下代码对 DataFrame
进行排序。
import pandas as pd
df = pd.DataFrame(
{
"col1": ["g", "t", "n", "w", "n", "g"],
"col2": [5, 2, 5, 1, 3, 6],
"col3": [0, 7, 2, 8, 1, 2],
}
)
print(df.sort_values(by=["col1"]))
我们按 col1
对 DataFrame
进行了排序。运行上面的代码后,你将获得以下输出。
col1 col2 col3
0 g 5 0
5 g 6 2
2 n 5 2
4 n 3 1
1 t 2 7
3 w 1 8
我们也可以使用多个列进行排序,让我们如下更改上述代码的最后一行,
print(df.sort_values(by=["col1", "col2"]))
运行代码后,我们将获得以下输出。
col1 col2 col3
0 g 5 0
5 g 6 2
4 n 3 1
2 n 5 2
1 t 2 7
3 w 1 8
现在,DataFrame
也通过 col2
进一步排序。
DataFrame
排序顺序-参数 Ascending
默认情况下,排序按升序排列,要按降序更改 DataFrame
,我们需要设置标志 ascending=False
。
print(df.sort_values(by=["col1", "col2"], ascending=False))
运行代码后,我们将获得以下输出。
col1 col2 col3
3 w 1 8
1 t 2 7
2 n 5 2
4 n 3 1
5 g 6 2
0 g 5 0
DataFrame
排序顺序 - 参数 na_position
na_position
在排序后指定 NaN
的位置.last
将 NaN
放在排序的最后,它的默认值是 first
,将 NaN
放在排序结果的开头。
参考下面的 DataFrame
,
import numpy as np
import pandas as pd
s = pd.Series([np.nan, 2, 4, 10, 7])
print(s.sort_values(na_position="last"))
运行代码后,我们将获得以下输出。
1 2.0
2 4.0
4 7.0
3 10.0
0 NaN