如何获取 Pandas DataFrame 的行数
Asad Riaz
2023年1月30日
Pandas
Pandas DataFrame Row
-
.shape
方法获取数据DataFrame
的行数 -
.len(DataFrame.index)
获取 Pandas 行数的最快方法 -
dataframe.apply()
计算满足 Pandas 条件的行
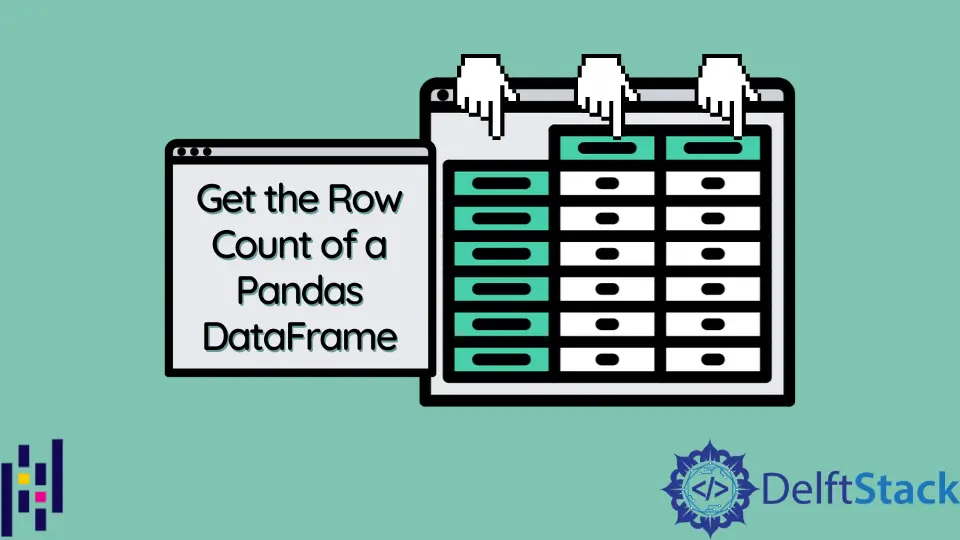
我们将介绍如何使用 shape
和 len(DataFrame.index)
之类的不同方法来获取 Pandas DataFrame
的行数。我们发现,len(DataFrame.index)
是最快的,存在明显的性能差异。
我们还将研究如何使用 dataframe.apply()
获取行中有多少个元素满足条件。
.shape
方法获取数据 DataFrame
的行数
假设 df 是我们的 DataFrame
,对于计算行数来说,
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(np.arange(15).reshape(3, 5))
print(df)
print("Row count is:", df.shape[0])
输出:
0 1 2 3 4
0 0 1 2 3 4
1 5 6 7 8 9
2 10 11 12 13 14
Row count is: 3
对于列数,我们可以使用 df.shape[1]
。
.len(DataFrame.index)
获取 Pandas 行数的最快方法
我们可以通过获取成员变量的长度索引来计算 DataFrame
中的行:
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(np.arange(15).reshape(3, 5))
print(df)
print("Row count is:", len(df.index))
输出:
0 1 2 3 4
0 0 1 2 3 4
1 5 6 7 8 9
2 10 11 12 13 14
Row count is: 3
我们还可以传递 df.axes[0]
而不是 df.index
:
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(np.arange(15).reshape(3, 5))
print(df)
print("Row count is:", len(df.axes[0]))
输出:
0 1 2 3 4
0 0 1 2 3 4
1 5 6 7 8 9
2 10 11 12 13 14
Row count is: 3
对于列数,我们可以使用 df.axes[1]
。
dataframe.apply()
计算满足 Pandas 条件的行
通过计算返回的 dataframe.apply()
结果序列中 True
的数目,我们可以得到满足条件的 DataFrame
中的行的元素。
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(np.arange(15).reshape(3, 5))
counterFunc = df.apply(lambda x: True if x[1] > 3 else False, axis=1)
numOfRows = len(counterFunc[counterFunc == True].index)
print(df)
print("Row count > 3 in column[1]is:", numOfRows)
输出:
0 1 2 3 4
0 0 1 2 3 4
1 5 6 7 8 9
2 10 11 12 13 14
Row count > 3 in column[1]is: 2
我们得到行中 column[1] > 3
的行数。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe