将 Pandas DataFrame 导出到 Excel 文件
-
使用
to_excel()
函数将 PandasDataFrame
导出到 Excel 文件中 -
使用
ExcelWriter()
方法导出 PandasDataFrame
-
将多个 Pandas
dataframes
导出到多个 Excel 表格中
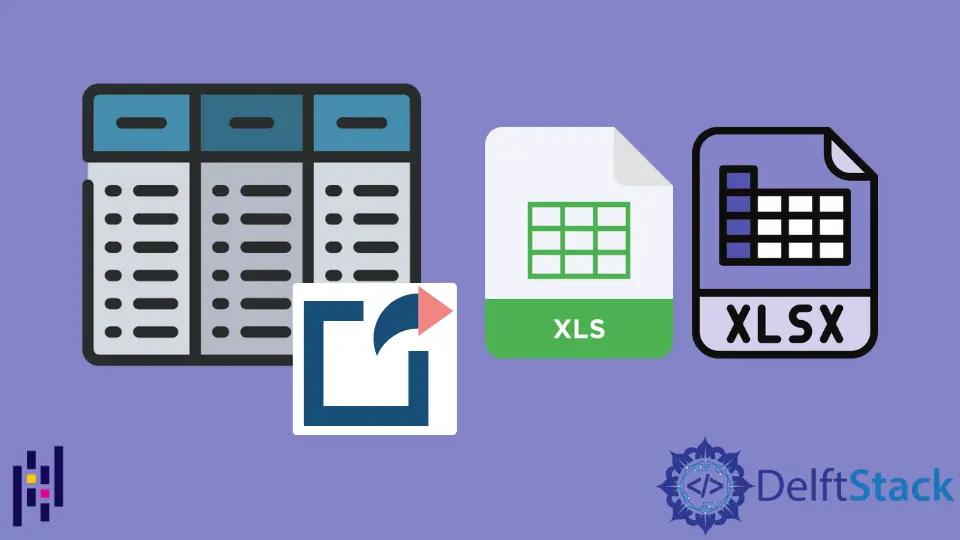
我们将在本教程中演示如何使用两种不同的方式将 Pandas DataFrame
导出到 excel 文件。第一种方法是通过使用文件名调用 to_excel()
函数,将 Pandas DataFrame
导出到 excel 文件。本文中讨论的另一种方法是 ExcelWriter()
方法。此方法将对象写入 Excel 工作表,然后使用 to_excel
函数将它们导出到 Excel 文件中。
在本指南中,我们还将讨论如何使用 ExcelWriter()
方法将多个 Pandas dataframes
添加到多个 Excel 工作表中。此外,我们在我们的系统上执行了多个示例来详细解释每种方法。
使用 to_excel()
函数将 Pandas DataFrame
导出到 Excel 文件中
当我们使用 dataframe.to_excel()
函数将 pandas DataFrame
导出到 excel 表时,它会直接将一个对象写入 excel 表中。要实现此方法,请创建一个 DataFrame
,然后指定 excel 文件的名称。现在,通过使用 dataframe.to_excel()
函数,将 Pandas DataFrame
导出到 excel 文件中。
在以下示例中,我们创建了一个名为 sales_record
的 DataFrame
,其中包含 Products_ID
、Product_Names
、Product_Prices
、Product_Sales
列。之后,我们为 excel 文件 ProductSales_sheet.xlsx
指定了名称。我们使用 sales_record.to_excel()
方法将所有数据保存到 excel 表中。
请参阅下面的示例代码:
import pandas as pd
# DataFrame Creation
sales_record = pd.DataFrame(
{
"Products_ID": {
0: 101,
1: 102,
2: 103,
3: 104,
4: 105,
5: 106,
6: 107,
7: 108,
8: 109,
},
"Product_Names": {
0: "Mosuse",
1: "Keyboard",
2: "Headphones",
3: "CPU",
4: "Flash Drives",
5: "Tablets",
6: "Android Box",
7: "LCD",
8: "OTG Cables",
},
"Product_Prices": {
0: 700,
1: 800,
2: 200,
3: 2000,
4: 100,
5: 1500,
6: 1800,
7: 1300,
8: 90,
},
"Product_Sales": {0: 5, 1: 13, 2: 50, 3: 4, 4: 100, 5: 50, 6: 6, 7: 1, 8: 50},
}
)
# Specify the name of the excel file
file_name = "ProductSales_sheet.xlsx"
# saving the excelsheet
sales_record.to_excel(file_name)
print("Sales record successfully exported into Excel File")
输出:
Sales record successfully exported into Excel File
执行上述源代码后,excel 文件 ProductSales_sheet.xlsx
将存储在当前运行项目的文件夹中。
使用 ExcelWriter()
方法导出 Pandas DataFrame
Excelwrite()
方法也可用于将 Pandas DataFrame
导出到 excel 文件中。首先,我们使用 Excewriter()
方法将对象写入 excel 表,然后使用 dataframe.to_excel()
函数,我们可以将 DataFrame
导出到 excel 文件中。
请参阅下面的示例代码。
import pandas as pd
students_data = pd.DataFrame(
{
"Student": ["Samreena", "Ali", "Sara", "Amna", "Eva"],
"marks": [800, 830, 740, 910, 1090],
"Grades": ["B+", "B+", "B", "A", "A+"],
}
)
# writing to Excel
student_result = pd.ExcelWriter("StudentResult.xlsx")
# write students data to excel
students_data.to_excel(student_result)
# save the students result excel
student_result.save()
print("Students data is successfully written into Excel File")
输出:
Students data is successfully written into Excel File
将多个 Pandas dataframes
导出到多个 Excel 表格中
在上述方法中,我们将单个 Pandas DataFrame
导出到 Excel 表格中。但是,使用这种方法,我们可以将多个 Pandas dataframes
导出到多个 Excel 表格中。
请参阅以下示例,其中我们将多个 DataFrame
分别导出到多个 Excel 工作表中:
import pandas as pd
import numpy as np
import xlsxwriter
# Creating records or dataset using dictionary
Science_subject = {
"Name": ["Ali", "Umar", "Mirha", "Asif", "Samreena"],
"Roll no": ["101", "102", "103", "104", "105"],
"science": ["88", "60", "66", "94", "40"],
}
Computer_subject = {
"Name": ["Ali", "Umar", "Mirha", "Asif", "Samreena"],
"Roll no": ["101", "102", "103", "104", "105"],
"computer_science": ["73", "63", "50", "95", "73"],
}
Art_subject = {
"Name": ["Ali", "Umar", "Mirha", "Asif", "Samreena"],
"Roll no": ["101", "102", "103", "104", "105"],
"fine_arts": ["95", "63", "50", "60", "93"],
}
# Dictionary to Dataframe conversion
dataframe1 = pd.DataFrame(Science_subject)
dataframe2 = pd.DataFrame(Computer_subject)
dataframe3 = pd.DataFrame(Art_subject)
with pd.ExcelWriter("studentsresult.xlsx", engine="xlsxwriter") as writer:
dataframe1.to_excel(writer, sheet_name="Science")
dataframe2.to_excel(writer, sheet_name="Computer")
dataframe3.to_excel(writer, sheet_name="Arts")
print("Please check out subject-wise studentsresult.xlsx file.")
输出:
Please check out subject-wise studentsresult.xlsx file.