在 Windows PowerShell 中终止脚本
-
在 Windows PowerShell 中使用
Exit
命令终止脚本 -
在 Windows PowerShell 中使用
Throw
命令终止脚本 -
Return
命令 -
break
命令 -
continue
命令
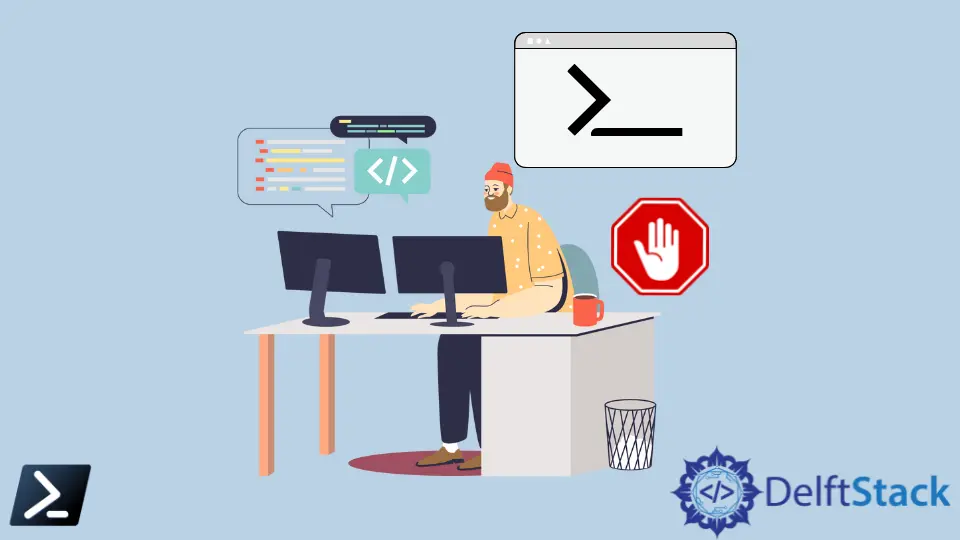
在 Windows PowerShell 中有多种终止脚本的方法。但是,其中一些在上下文方面可能听起来相似,但在功能方面,它们的实际目的却彼此不同。
本文将列举在 Windows PowerShell 中终止脚本的方法并一一定义。
在 Windows PowerShell 中使用 Exit
命令终止脚本
Exit
命令将退出从其名称派生的脚本。如果你没有打开的会话,此命令还将关闭你的 shell 或脚本窗口。Exit
命令还有助于通过使用退出代码提供反馈。
exit
仅运行 exit
命令可以具有退出代码 0
(默认),表示成功或正常终止,或 1
,表示失败或未捕获的抛出。
退出代码的优点是退出代码是完全可定制的。只要退出码是整数,退出码就有效。此外,要知道最后的退出代码,你可以输出变量 $LASTEXITCODE
。
退出.ps1
Write-Output 'Running sample script.'
exit 200
示例代码:
PS C:\>powershell.exe .\Exit.ps1
PS C:\>Running sample script.
PS C:\>Write-Output $LASTEXITCODE
PS C:\>200
另外,请记住,当你从正在运行的 PowerShell 脚本中使用 exit
命令调用另一个 PowerShell 文件时,正在运行的脚本也将终止。
在 Windows PowerShell 中使用 Throw
命令终止脚本
Throw
命令类似于带有退出代码的 Exit
命令,但信息量更大。你可以使用命令和自定义表达式来生成终止错误。通常,Throw
命令用于 Try-Catch
表达式内的 Catch
块内,以充分描述异常。
示例代码:
Try{
$divideAnswer = 1/0
}Catch{
Throw "The mathematical expression has a syntax error"
}
输出:
The mathematical expression has a syntax error
At line:4 char:5
+ Throw "The mathematical expression has a syntax error"
+ ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
+ CategoryInfo : OperationStopped: (The mathematica... a syntax error:String) [], RuntimeException
+ FullyQualifiedErrorId : The mathematical expression has a syntax error
Return
命令
与 Exit
命令不同,Return
命令将返回到其先前的调用点并且不会关闭你的脚本窗口。
通常,我们使用 Return
命令从脚本中某处执行的函数返回值。
示例代码:
Function sumValues($int1,$int2){
Return ($int1 + $int2)
}
# The function sumValues is called below, and the script will return to
# the same line with a value and store it in the output variable
$output = sumValues 1 2
Write-Output $output
输出:
3
break
命令
我们使用 break
命令来终止循环和案例。
示例代码:
$numList = 1,2,3,4,5,6,7,8
foreach($number in $numList){
if ($number -eq 8){
#Terminate loop if number variable is equal to 8
break
}
Write-Output $number
}
输出:
1
2
3
4
5
6
7
如果我们有嵌套循环,我们只会从调用 break
命令的循环中中断。
示例代码:
While ($true) {
While ($true) {
#The break command will only break out of this while loop
break
}
#The script will continue to run on this line after breaking out of the inner while loop
}
如果你想跳出一个特定的嵌套循环,break
命令使用标签作为它的参数。
While ($true) {
:thisLoop While ($true) {
While ($true) {
#The break command below will break out of the `thisLoop` while loop.
Break thisLoop
}
}
}
continue
命令
continue
命令还会在循环级别终止脚本。尽管如此,continue
命令并不会立即终止整个循环,而是仅终止当前迭代并保持循环继续进行,直到所有迭代都已处理完毕。
我们可以说这是一个在执行循环时跳过某些内容的命令。
示例代码:
$numList = 1,2,3,4,5,6,7,8
foreach($number in $numList){
if ($number -eq 2){
#The continue command below will skip number 2 and will directly process the next iteration, resetting the process to the top of the loop.
continue
}
Write-Output $number
}
输出:
1
3
4
5
6
7
8
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn