如何在 PowerShell 中使用 Read-Host 设置默认值
-
PowerShell 中
Read-Host
命令的概述 -
使用条件语句在 PowerShell 中通过
Read-Host
命令设置默认值 -
使用函数在 PowerShell 中通过
Read-Host
命令设置默认值 -
使用 Do-While 循环在 PowerShell 中通过
Read-Host
命令设置默认值 - 结论
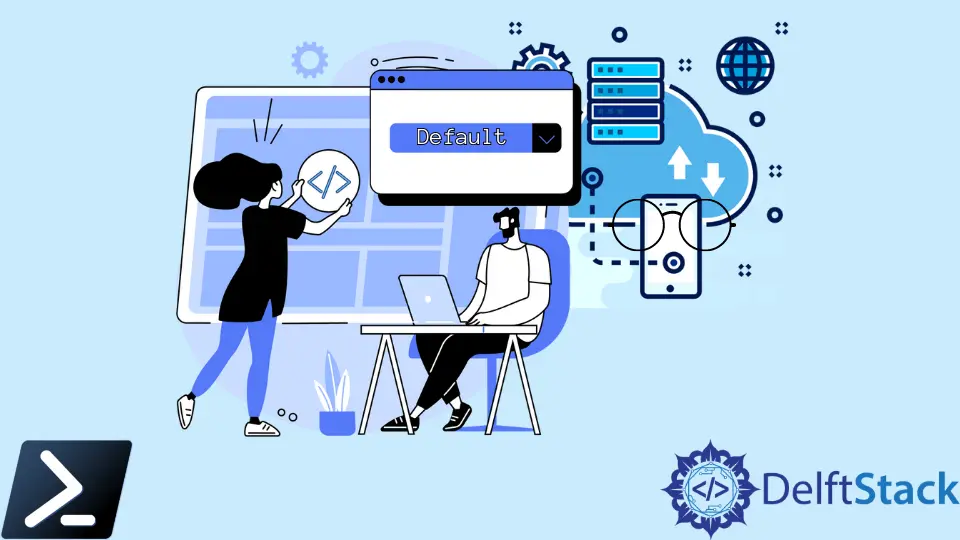
PowerShell 是一种强大的脚本语言,提供多种与用户交互的方式。一种常见的方法是使用 Read-Host
提示用户输入。
然而,在某些情况下,提供默认值是有益的。在本指南中,我们将探讨在 PowerShell 中将默认值与 Read-Host
结合使用的不同方法。
PowerShell 中 Read-Host
命令的概述
Read-Host
是 PowerShell 中的一个基本 Cmdlet,允许脚本通过提示用户输入与用户交互。这是一个在脚本执行过程中收集信息的多功能工具。在需要动态或特定于用户的信息的场景中,该 Cmdlet 特别有用。
语法:
Read-Host [-Prompt] <String> [-AsSecureString] [-Credential <PSCredential>] [-ErrorAction <ActionPreference>] [-Timeout <Int32>] [-OutVariable <String>] [-OutBuffer <Int32>] [-InputObject <PSObject>] [<CommonParameters>]
在这里,关键参数包括:
-Prompt
:指定将显示给用户的消息。-AsSecureString
:请求输入作为安全字符串(密码)。-Credential
:提示输入用户名和密码。返回一个PSCredential
对象。-ErrorAction
:定义发生错误时要采取的操作。-Timeout
:设置 Cmdlet 等待输入的时间限制。-OutVariable
:将结果存储在一个变量中。-OutBuffer
:确定在输出之前缓冲的对象数量。-InputObject
:允许从管道输入。
Read-Host
命令的基本用法
$userInput = Read-Host "Please enter your name"
在此示例中,用户将被提示“请输入您的姓名”。他们输入的值将存储在变量 $userInput
中。
安全字符串输入
$securePassword = Read-Host "Please enter your password" -AsSecureString
此命令提示用户输入密码,输入将作为安全字符串存储在变量 $securePassword
中。
超时功能
$userInput = Read-Host "Please enter your choice" -Timeout 10
此命令提示用户输入,并将在最长 10
秒内等待。如果在此时间内没有提供输入,则变量 $userInput
将为空。
您可以使用条件语句、函数、循环或结合 Read-Host
的尝试捕捉块来结合使用默认值。
使用条件语句在 PowerShell 中通过 Read-Host
命令设置默认值
创建一个名为 defaultName
的变量,并赋予它字符串值 John Doe
。如果用户选择不提供输入,则将使用该值作为替代。
$defaultName = "John Doe"
定义一个新变量 userName
,该变量将产生一个消息,询问用户的输入,并将利用 Read-Host
命令执行此操作。
$userName = Read-Host ("Please enter your name (default is {0})" -f $defaultName)
下面的代码实现了一个条件,检查用户是否提供了任何输入。它检查变量 $userName
是否为 null 或空字符串。
如果用户提供了一些输入(不是空字符串),则将 $defaultName
的值更新为用户的输入。
if (-not $userName) { $userName = $defaultName }
完整代码:
$defaultName = "John Doe"
$userName = Read-Host ("Please enter your name (default is {0})" -f $defaultName)
if (-not $userName) { $userName = $defaultName }
Write-Host "Hello, $($userName)!"
输出:
在下一个方法中,我们将接收用户输入的过程移到检查用户是否输入任何值的条件内部。这使我们可以更好地跟踪用户是否提供了任何数据。
如果用户没有任何操作,我们使用默认值 John Doe
。但是如果用户提供输入,我们就使用存储在 userName
变量中的值。
代码:
$defaultName = "John Doe"
if (($userName = Read-Host "Press enter to accept default value $defaultName") -eq '') { $defaultName } else { $userName }
输出:
最后的方法验证用户是否提供了输入,如果是的话,则将用户输入的值直接分配给名为 defaultName
的变量。
代码:
$defaultName = "John Doe"
if ($userName = Read-Host "Value [$defaultName]") { $defaultName = $userName }
输出:
这样,它不必使用 else
条件。
使用函数在 PowerShell 中通过 Read-Host
命令设置默认值
函数封装了一组可以在脚本中重复使用的指令。我们将设计一个名为 Read-HostWithDefault
的函数,该函数包含默认值。
function Read-HostWithDefault {
param (
[string]$prompt,
[string]$default
)
$userInput = Read-Host "$prompt (default is $default)"
$userInput = if ($userInput -ne "") { $userInput } else { $default }
return $userInput
}
说明:
function Read-HostWithDefault { ... }
:这定义了名为Read-HostWithDefault
的函数。param ( [string]$prompt, [string]$default )
:这指定函数期望的参数。在这种情况下,它是一个提示消息和一个默认值。$userInput = Read-Host "$prompt (default is $default)"
:这一行提示用户输入,同时显示提示和默认值。输入存储在变量$userInput
中。$userInput = if ($userInput -ne "") { $userInput } else { $default }
:这一行检查$userInput
是否不是空字符串($userInput -ne ""
)。如果此条件为真(意味着用户提供了输入),则$userInput
保留其值。如果为假(意味着用户没有提供输入),则$default
被分配给$userInput
。return $userInput
:最后,函数返回结果值。
现在,让我们看看如何在实际场景中使用这个函数:
$defaultName = "John Doe"
$name = Read-HostWithDefault -prompt "Enter your name" -default $defaultName
Write-Host "Hello, $name!"
说明:
$defaultName = "John Doe"
:在这里,我们为用户的姓名设置一个默认值。$name = Read-HostWithDefault -prompt "Enter your name" -default $defaultName
:这一行提示用户输入姓名,显示提供的提示消息和默认值。函数Read-HostWithDefault
被调用,并传入适当的参数。Write-Host "Hello, $name!"
:这生成一个问候语,该问候语可以是用户提供的或是默认值。
输出:
使用 Do-While 循环在 PowerShell 中通过 Read-Host
命令设置默认值
do-while
循环是 PowerShell 中的一种控制结构,只要指定条件为真,就会重复执行代码块。当您希望确保某个操作至少执行一次时,这特别有用。
do-while
循环的基本语法是:
do {
# Code to be executed
} while (condition)
do
语句中的代码块将继续执行,只要 while
关键字后面的条件为真。
让我们考虑一个示例,在该示例中,我们希望提示用户输入姓名,但如果他们没有输入任何内容,则提供默认值 John Doe
:
$defaultName = "John Doe"
do {
$userName = Read-Host "Please enter your name (default is $defaultName)"
$userName = if ($userName) { $userName } else { $defaultName }
} while (-not $userName)
Write-Host "Hello, $userName!"
说明:
$defaultName = "John Doe"
:这一行设置我们希望在用户不提供任何输入时使用的默认值。do { ... } while (-not $userName)
:这启动一个do-while
循环。do
语句中的代码块将至少执行一次。条件(-not $userName)
确保只要没有提供$userName
,循环就会继续。$userName = Read-Host "Please enter your name (default is $defaultName)"
:这提示用户输入姓名,同时在消息中显示默认值。$userName = if ($userName) { $userName } else { $defaultName }
:这一行使用if-else
语句确定$userName
的值。如果$userName
有值(即不为空或 null),它将保留该值。否则,它将分配默认姓名$defaultName
。-not $userName
:该条件检查$userName
是否为空或未提供。如果为空,循环将继续,重新提示用户。- 一旦用户提供了输入,条件
-not $userName
将变为假,循环终止。
输出:
结论
在本综合指南中,我们探讨了在 PowerShell 中通过 Read-Host
命令设置默认值的各种方法。每种方法根据脚本的具体要求提供了不同的优势。
- 条件语句:此方法允许直接检查用户输入,并在处理不同场景时提供灵活性。
- 函数:通过将逻辑封装在函数中,您可以创建可重复使用的代码,无缝集成默认值。
- Do-While 循环:此方法确保某个操作至少执行一次,适用于用户输入至关重要的场景。
通过理解和应用这些技术,您可以增强用户体验和 PowerShell 脚本的弹性。请记住选择最符合您特定脚本要求的方法。
I am Waqar having 5+ years of software engineering experience. I have been in the industry as a javascript web and mobile developer for 3 years working with multiple frameworks such as nodejs, react js, react native, Ionic, and angular js. After which I Switched to flutter mobile development. I have 2 years of experience building android and ios apps with flutter. For the backend, I have experience with rest APIs, Aws, and firebase. I have also written articles related to problem-solving and best practices in C, C++, Javascript, C#, and power shell.
LinkedIn