在 Windows PowerShell 中逐行读取文件
Marion Paul Kenneth Mendoza
2023年1月30日
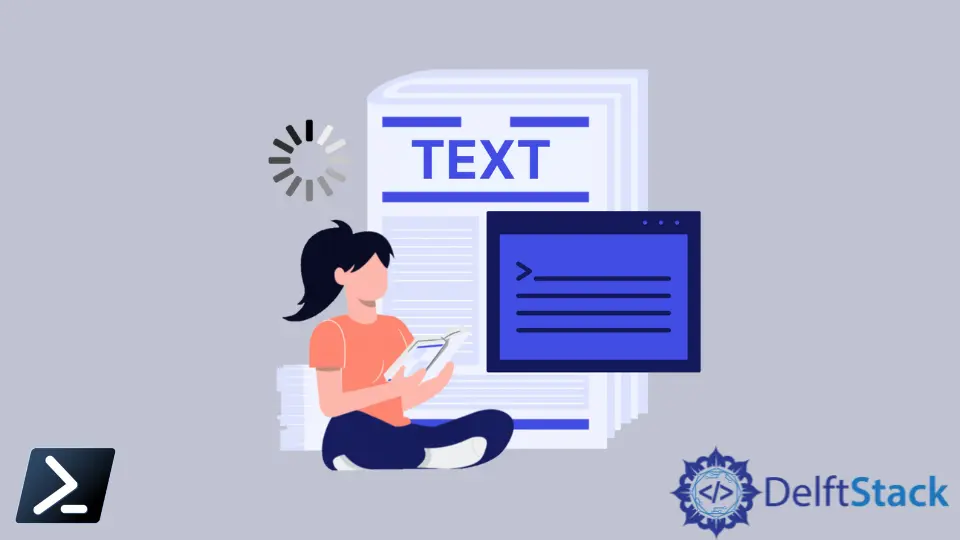
在 Windows PowerShell 中,我们可以使用 Get-Content
cmdlet 从文件中读取文件。但是,该 cmdlet 会立即将整个文件内容加载到内存中,这会导致大文件失败或冻结。
为了解决这个问题,我们可以做的是我们可以逐行读取文件,本文将向你展示如何。
在开始之前,最好创建一个包含多行的示例文本文件。例如,我创建了一个包含以下行的 file.txt
。
file.txt
:
one
two
three
four
five
six
seven
eight
nine
ten
根据微软的说法,正则表达式是一种用于匹配文本的模式。它可以由文字字符、运算符和其他结构组成。本文将尝试不同的脚本,其中涉及一个称为正则表达式的标准变量,用 $regex
表示。
改进 Get-Content
Cmdlet 语法
我们最初讨论过 Get-Content
cmdlet 在我们加载大文件时存在缺陷,因为它会将文件的内容一次全部加载到内存中。但是,我们可以通过使用 foreach
循环逐行加载来改进它。
示例代码:
foreach($line in Get-Content .\file.txt) {
if($line -match $regex){
Write-Output $line
}
}
使用 switch
加载特定行
我们可以使用 $regex
变量通过创建自定义 $regex
值并在 switch case 函数中匹配它来输入文件中的特定行。
示例代码:
$regex = '^t'
switch -regex -file file.txt {
$regex { "line is $_" }
}
输出:
line is two
line is three
line is ten
在这个例子中,我们定义了一个自定义值 $regex
,它只匹配并显示以字符 t
开头的行。
使用 PowerShell 中的 [System.IO.File]
类逐行读取文件
如果你有权访问 .NET 库,则可以使用 [System.IO.File]
类从文件中读取内容。它是 Get-Content
的绝佳替代品,因为你不需要正则表达式来逐行处理文件。
示例代码:
foreach($line in [System.IO.File]::ReadLines("C:\yourpathhere\file.txt"))
{
Write-Output $line
}
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn