如何在 PowerShell 中创建和抛出新的异常
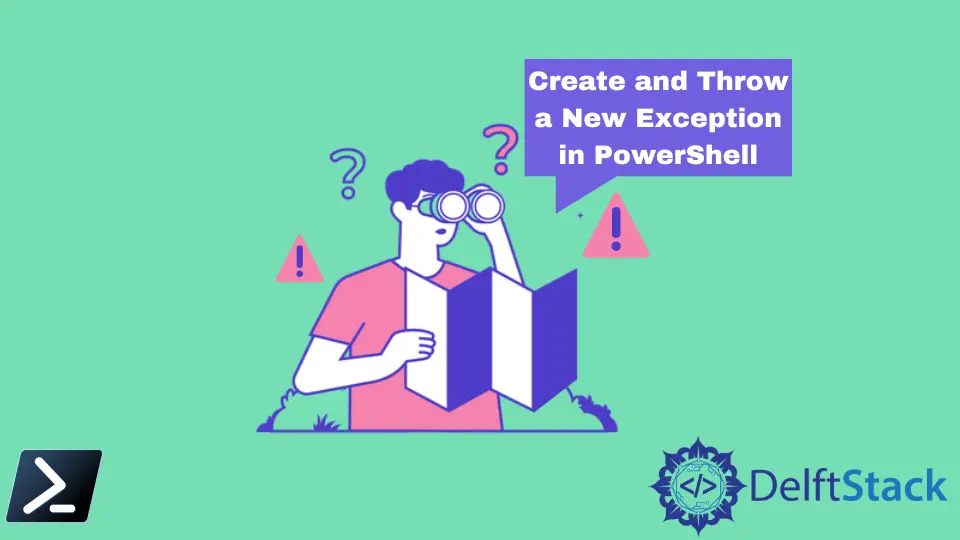
异常处理是编写强大且可靠的 PowerShell 脚本的关键方面。通过创建和抛出异常,您可以有效地传达在脚本执行过程中可能发生的错误和问题。
尽管 PowerShell 提供了几种内置异常类型,但在某些情况下,您需要创建并抛出自定义异常,以便根据脚本的特定需求量身定制错误处理。
在本文中,我们将探讨在 PowerShell 中创建和抛出自定义异常的过程,并提供详细的示例和输出。
创建自定义异常类
在深入探讨自定义异常之前,让我们简要回顾一下编程中异常的概念。
异常是干扰程序正常流程的意外事件或错误。当发生异常时,控制权转移到指定的异常处理代码块,从而实现优雅的错误管理。
PowerShell 提供多种内置异常类型,如 System.Management.Automation.CmdletInvocationException
、System.IO.IOException
和 System.Exception
,以处理常见错误。然而,当您遇到特定错误情况或希望为用户提供清晰且具有信息量的错误消息时,创建自定义异常是有益的。
要在 PowerShell 中创建自定义异常,您需要首先定义一个继承自 [System.Exception]
基类的新异常类。然后,您可以添加属性和方法来定制异常的行为。
让我们通过一个实际的例子来说明这一点:
class MyCustomException : [System.Exception] {
MyCustomException() {
}
MyCustomException([string] $message) {
$this.Message = $message
}
[string] GetMessage() {
return "Custom Exception: $($this.Message)"
}
}
在这个示例中,我们创建了一个名为 MyCustomException
的自定义异常类。MyCustomException
类继承自 [System.Exception]
基类。
然后,定义两个构造函数:一个是没有参数的默认构造函数,另一个是接受自定义错误消息的构造函数。
最后,我们包含一个 GetMessage
方法来返回格式化的错误消息。这个方法在处理异常时提供具体细节时可能非常有用。
使用 throw
语句抛出自定义异常
一旦您定义了自定义异常类,就可以在脚本中使用 throw
语句抛出它。为此,创建自定义异常的实例并传递相关的错误消息。
下面是一个演示如何抛出自定义异常的示例:
try {
# Simulate code that may cause an error
$result = 1 / 0
}
catch {
$errorMessage = "An error occurred: $($_.Exception.Message)"
throw [MyCustomException]::new($errorMessage)
}
在 try
块中,我们故意通过尝试除以零来创建一个错误。
在 catch
块中,我们捕获错误,创建自定义错误消息,然后抛出一个包含该错误消息的 MyCustomException
实例。
处理自定义异常
处理自定义异常类似于在 PowerShell 中处理内置异常。您可以使用 try
、catch
和 finally
块优雅地管理错误。
下面是一个演示捕获和处理自定义异常的示例:
try {
# Simulate code that may cause an error
$result = 1 / 0
}
catch [MyCustomException] {
Write-Host "Custom Exception Caught: $($_.Exception.Message)"
}
catch {
Write-Host "Other Exception Caught: $($_.Exception.Message)"
}
在这段代码中,我们在 try
块中尝试除以零。第一个 catch
块专门捕获 MyCustomException
并显示一条消息,表示捕获到了自定义异常,以及我们提供的自定义错误消息。
如果发生其他任何异常,第二个 catch
块作为通用异常处理程序会捕获它并显示不同的消息。
让我们执行脚本并查看输出:
Custom Exception Caught: An error occurred: Attempted to divide by zero.
在这个示例中,我们故意通过尝试在 try
块中除以零来触发错误。MyCustomException
类的 catch
块捕获了错误并显示了一条消息,说明 Custom Exception Caught
。
此外,它提供了自定义错误消息:An error occurred: Attempted to divide by zero
。如果发生任何其他异常,它们将由通用 catch
块捕获,但在这个例子中并不适用。
以下是创建和抛出自定义异常的完整 PowerShell 代码,以及如何处理的示例:
class MyCustomException : [System.Exception] {
MyCustomException() {
}
MyCustomException([string] $message) {
$this.Message = $message
}
[string] GetMessage() {
return "Custom Exception: $($this.Message)"
}
}
try {
# Simulate code that may cause an error (division by zero)
$result = 1 / 0
}
catch {
$errorMessage = "An error occurred: $($_.Exception.Message)"
throw [MyCustomException]::new($errorMessage)
}
try {
# Simulate code that may cause another error (e.g., accessing a non-existent variable)
$nonExistentVariable = $undefinedVariable
}
catch [MyCustomException] {
Write-Host "Custom Exception Caught: $($_.Exception.Message)"
}
catch {
Write-Host "Other Exception Caught: $($_.Exception.Message)"
}
此代码将输出异常处理的结果,区分自定义异常和其他异常。
结论
在 PowerShell 中创建和抛出自定义异常使您能够提供更具信息性的错误消息,并增强脚本的可靠性和可维护性。这些自定义异常帮助您根据特定脚本要求量身定制错误处理,确保更顺畅的用户体验和改进的故障排除能力。