如何在 if 语句中组合多个条件
-
PowerShell 中的
if
语句 -
在 PowerShell 的
if
语句中使用逻辑运算符组合多个条件 -
在 PowerShell 的
if
语句中使用多个运算符组合多个条件 -
在 PowerShell 中嵌套
if
语句以组合多个条件 - 结论
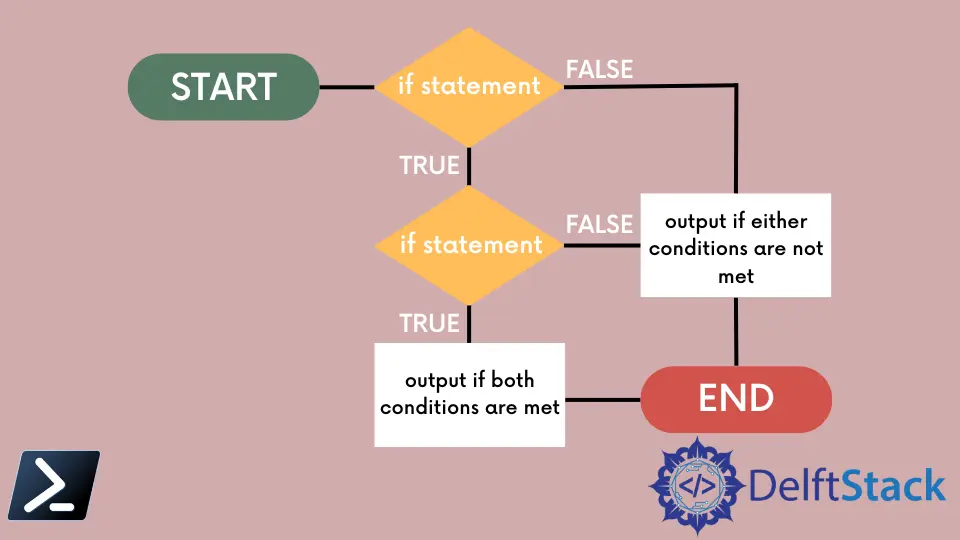
在 PowerShell 中,if
语句是一个强大的决策工具。然而,许多任务要求您结合多个条件以获得更精确的控制。
在本文中,我们将查看如何使用 PowerShell 的 if
语句中的逻辑运算符组合多个条件的各种方法。
PowerShell 中的 if
语句
以下是一个简单的 if
语句示例。如果括号内的条件评估为真,它将执行命令 Write-Host "2 is equal to 2."
。
代码:
if (2 -eq 2) {
Write-Host "2 is equal to 2."
}
如果括号 ()
中指定的条件评估为 $true
,则会运行大括号 {}
中的命令。
输出:
2 is equal to 2.
如果条件为 $false
,则将跳过该代码块。上述示例使用一个条件,但您也可以在 if
语句中评估多个条件。
在 PowerShell 的 if
语句中使用逻辑运算符组合多个条件
逻辑运算符连接 PowerShell 中的语句和表达式。您可以使用单个表达式来测试多个条件。
PowerShell 中支持的逻辑运算符有 -and
、-or
、-not
、-xor
和 !
。在本文中,我们将仅讨论如何使用 -and
、-or
、-not
和 -xor
运算符在 PowerShell 的 if
语句中组合多个条件。
在 PowerShell 的 if
语句中使用 -and
运算符组合多个条件
以下示例使用 -and
运算符连接 if
语句中的两个语句。如果第一个条件 5 小于 10
为真且第二个条件 7 大于 5
为真,则将执行 Write-Host
命令。
代码:
if ((5 -lt 10) -and (7 -gt 5)) {
Write-Host "The above conditions are true."
}
输出:
The above conditions are true.
由于在这种情况下两个条件都为真,因此代码块将被执行,并在输出中打印消息 "The above conditions are true."
。
在 PowerShell 的 if
语句中使用 -or
运算符组合多个条件
在此代码中,我们使用 -lt
(小于)和 -gt
(大于)运算符来检查 5 是否小于 10 或 7 是否大于 5。然后,我们使用 -or
运算符检查 (5 -lt 10)
或 (7 -gt 5)
中的任一个是否为真。
代码:
if ((5 -lt 10) -or (7 -gt 5)) {
Write-Host "At least one of the above conditions is true."
}
else {
Write-Host "Neither of the above conditions is true."
}
输出:
At least one of the above conditions is true.
由于原始代码中的两个条件都为真,因此它打印出 "At least one of the conditions is true."
。
在 PowerShell 的 if
语句中使用 -not
运算符组合多个条件
在以下代码中,我们有一个表示数字值的变量 $number
。我们使用 -lt
(小于)和 -gt
(大于)运算符来检查该数字是否小于 5 或大于 10。
-or
运算符用于组合左侧和右侧的两个条件。如果至少有一个条件为真,则返回真。由于两个条件都为假,因此 -or
表达式评估为假。
-not
运算符应用于 -or
表达式的结果。由于 -or
表达式为假,应用 -not
后结果变为真。
代码:
$number = 7
if (-not ($number -lt 5 -or $number -gt 10)) {
Write-Host "The number is between 5 and 10 (inclusive)."
}
else {
Write-Host "The number is outside the range of 5 to 10."
}
输出:
The number is between 5 and 10 (inclusive).
因为 -not
运算符使合并条件为真,所以 if
块中的代码被执行。输出指示 $number = 7
的值在指定的 5 到 10 的范围内(含)。
在 PowerShell 的 if
语句中使用 -xor
运算符组合多个条件
以下代码检查两个条件,并使用 -xor
运算符来确定它们的组合真值。在 if
语句中,有两个条件:第一个条件是 5 是否小于 10,第二个条件是 7 是否大于 5。
-xor
运算符是一个排他性的 OR
运算符,这意味着它仅在其操作数中恰好有一个为真时返回真,如果两个操作数都为真或都为假,则返回假。
在这种情况下,两个条件都为真,因此 -xor
表达式评估为假,else
块中的代码将被执行。
代码:
if ((5 -lt 10) -xor (7 -gt 5)) {
Write-Host "Exactly one of the above conditions is true."
}
else {
Write-Host "Neither or both of the above conditions are true."
}
输出:
Neither or both of the above conditions are true.
输出打印 "Neither or both of the above conditions are true."
,因为它指示上述条件都不是唯一为真;两个条件要么一起为真,要么一起为假。
在 PowerShell 的 if
语句中使用多个运算符组合多个条件
同样,您可以在 PowerShell 的 if
语句中使用逻辑运算符组合多个条件。在此示例中,我们根据组合条件是否为 true
或 false
执行不同的命令。
下面的代码使用逻辑运算符 -and
和 -or
组合多个条件。如果这些条件中的任何一个为真,则将执行第一个命令 ( Write-Host "It is true."
),否则将执行第二个命令 (Write-Host "It is false."
)。
代码:
if (((10 -lt 20) -and (10 -eq 10)) -or ((15 -gt 5) -and (12 -lt 6))) {
Write-Host "It is true."
}
else {
Write-Host "It is false."
}
输出:
It is true.
在这里,if
语句检查组合条件并根据这些条件的评估执行适当的命令块。由于条件为真,它打印了消息 "It is true."
。
记得将每组条件括在括号 ()
中,并使用适合您特定要求的逻辑运算符。
在 PowerShell 中嵌套 if
语句以组合多个条件
嵌套 if
语句在您有复杂条件需要多层评估时非常有价值。例如,当您需要在特定上下文中检查一个条件或根据不同条件执行不同操作时,嵌套 if
语句允许您采用更结构化的方法。
在此代码中,我们声明一个变量 $number = 15
。然后,我们创建外部 if
语句,检查 $number 是否大于或等于 10
。
如果外部条件为真,嵌套 if
语句检查 $number 是否小于或等于 20
。
代码:
$number = 15
if ($number -ge 10) {
if ($number -le 20) {
Write-Host "The number is between 10 and 20."
}
}
输出:
The number is between 10 and 20.
在此代码中,外部 if
语句检查 $number
是否 大于或等于 10
。如果满足此条件,它会进入嵌套的 if
语句,检查 $number
是否 小于或等于 20
。
仅当两个条件都为真时,它才会执行 Write-Host
命令。在输出中,两个条件都得到满足,并打印出消息 "The number is between 10 and 20."
。
结论
总之,在 PowerShell 的 if
语句中组合多个条件对于编写高效脚本至关重要。通过逻辑运算符 -and
、-or
、-not
和 -xor
,您可以创建复杂的条件逻辑,以应对各种脚本挑战。
无论是简单条件还是嵌套 if
语句,PowerShell 都提供了强大的工具,帮助您做出明智的决策并在脚本中执行正确的操作。