在 PowerShell 中使用 CmdletBinding 属性
-
PowerShell 中的
CmdletBinding
属性 -
将
CmdletBinding
属性与Verbose
参数一起使用 -
将
CmdletBinding
属性与$PSCmdlet
对象和SupportsShouldProcess
一起使用 -
使用
CmdletBinding
属性和Parameter
属性来控制函数参数
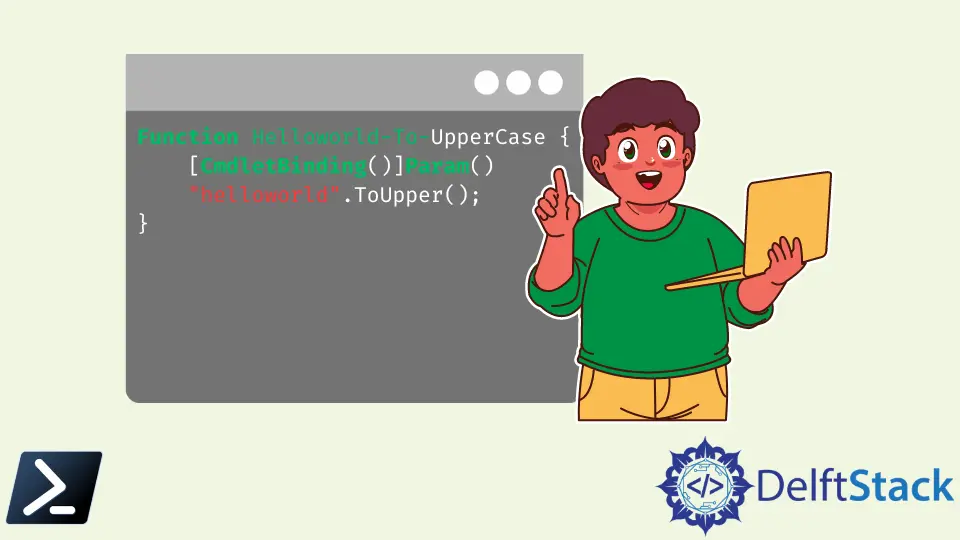
cmdlet
是在 PowerShell 环境中执行单一功能的轻量级脚本。cmdlet
可以用任何 .Net
语言编写。
通常,cmdlet
表示为执行命令的动词-名词对。该命令是对底层操作系统的命令,以由最终用户执行特定服务。
PowerShell 环境包括 200 多个基本的 cmdlet
,例如 New-Item
、Move-Item
、Set-Location
和 Get-Location
。cmdlet
共享一组简单的 PowerShell 函数中不可用的通用功能。
- 支持
-WhatIf
、ErrorAction
、Verbose
等常用参数。 - 提示确认
- 强制参数支持
PowerShell 中的 CmdletBinding
属性
通过继承上面讨论的基本 cmdlet
功能,可以将简单的 PowerShell 函数编写为高级函数。CmdletBinding
属性使你能够访问这些基本的 cmdlet
功能。
下面显示了带有所有可能参数的 CmdletBinding
属性语法。
{
[CmdletBinding(ConfirmImpact=<String>,
DefaultParameterSetName=<String>,
HelpURI=<URI>,
SupportsPaging=<Boolean>,
SupportsShouldProcess=<Boolean>,
PositionalBinding=<Boolean>)]
Param ($myparam)
Begin{}
Process{}
End{}
}
假设我们有一个名为 Helloworld-To-UpperCase
的简单 PowerShell 函数。
Function Helloworld-To-UpperCase {
"helloworld".ToUpper();
}
此函数没有附加参数。因此,这称为简单的 PowerShell 函数。
但是我们可以使用 CmdletBinding
属性将此功能转换为高级功能并访问基本的 cmdlet
功能和参数,如下所示。
Function Helloworld-To-UpperCase {
[CmdletBinding()]Param()
"helloworld".ToUpper();
}
Helloworld-To-UpperCase
函数已转换为继承所有基本 cmdlet
功能的高级函数。基本的 cmdlet
参数可用于此函数。
如果你在 PowerShell 窗口中使用 -
调用此函数,它应该列出来自 cmdlet
的所有常用参数。
helloworld-to-uppercase -
输出:
常见的 cmdlet
参数和功能可以在我们的高级功能中使用以扩展功能。
将 CmdletBinding
属性与 Verbose
参数一起使用
-verbose
是有价值的常用参数之一,可以在执行高级功能时显示消息。
Function Helloworld-To-UpperCase {
[CmdletBinding()]Param()
Write-Verbose "This is the common parameter usage -Version within our Helloworld-To-UpperCase function"
"helloworld".ToUpper();
}
当我们使用 -verbose
参数调用上述函数时,它会将所有 Write-Verbose
字符串打印到 PowerShell 窗口。
HelloWorld-To-UpperCase -Verbose
输出:
将 CmdletBinding
属性与 $PSCmdlet
对象和 SupportsShouldProcess
一起使用
由于我们使用了 CmdletBinding
属性,我们的高级函数可以轻松访问 $PSCmdlet
对象。该对象包含多个方法,例如 ShouldContinue
、ShouldProcess
、ToString
、WriteDebug
等。
将 CmdletBinding
属性与 ShouldContinue
方法一起使用
此方法允许用户处理确认请求。同时,必须将 SupportsShouldProcess
参数设置为 $True
。
ShouldContinue
方法有几个重载方法,我们将使用带有两个参数的方法。
Function Helloworld-To-UpperCase {
[CmdletBinding(SupportsShouldProcess=$True)]Param()
Write-Verbose "This is the common parameter usage -Version within our Helloworld-To-UpperCase function"
if ($PSCmdlet.ShouldContinue("Are you sure on making the helloworld all caps?", "Making uppercase with ToUpper")) {
"helloworld".ToUpper();
} Else {
"helloworld kept in lowercase."
}
}
我们可以使用 -Confirm
参数调用该函数,它会显示一个确认框,如下所示。
HelloWorld-To-UpperCase -Confirm
输出:
如果用户点击 Yes
,它应该实现 if
块中的方法并以大写字母打印 helloworld
字符串。
如果不是,它应该显示 helloworld 保持在小写
消息。
使用 CmdletBinding
属性和 Parameter
属性来控制函数参数
让我们让我们的高级函数将一个参数作为一个字符串。
Function Helloworld-To-UpperCase {
[CmdletBinding(SupportsShouldProcess=$True)]
Param([string]$word)
Write-Verbose "This is the common parameter usage -Version within our Helloworld-To-UpperCase function"
if ($PSCmdlet.ShouldContinue("Are you sure on making the helloworld all caps?", "Making uppercase with ToUpper")) {
$word.ToUpper();
} Else {
"helloworld kept in lowercase."
}
}
我们已将 Helloworld-To-UpperCase
函数更改为采用一个名为 $word
的字符串类型参数。当函数被调用时,我们需要提供一个字符串作为参数。
提供的文本将转换为大写。如果用户没有提供任何文本参数,该函数将给出一个空输出。
我们可以通过强制 $word
参数并给参数位置 0
来控制这一点。
Function Helloworld-To-UpperCase {
[CmdletBinding(SupportsShouldProcess=$True)]
Param(
[Parameter(
Mandatory=$True, Position=0
) ]
[string]$word
)
#Verbose
Write-Verbose "This is the common parameter usage -Version within our Helloworld-To-UpperCase function"
#If/Else block for request processing
if ($PSCmdlet.ShouldContinue("Are you sure on making the helloworld all caps?", "Making uppercase with ToUpper")) {
$word.ToUpper();
} Else {
"helloworld kept in lowercase."
}
}
我们添加了一些标志来控制 $word
参数的行为。由于它是强制性的,因此我们需要在函数执行时提供一个字符串值。
HelloWorld-To-UpperCase -Confirm "stringtouppercase"
如果我们不提供 text 参数,PowerShell 会一直要求这样做。
你可以使用多个标志来控制函数中的参数,如以下 Parameter
属性语法所示。
Param
(
[Parameter(
Mandatory=<Boolean>,
Position=<Integer>,
ParameterSetName=<String>,
ValueFromPipeline=<Boolean>,
ValueFromPipelineByPropertyName=<Boolean>,
ValueFromRemainingArguments=<Boolean>,
HelpMessage=<String>,
)]
[string[]]
$Parameter1
)
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.