在 PowerShell 中的字节数组
- 在 PowerShell 中将数据转换为字节数组
- 在 PowerShell 5+ 中将数据转换为字节数组
- 在 PowerShell 7+ 中将数据转换为字节数组
- 在 PowerShell 中将字节数组转换为字符串
- 在 PowerShell 中将字符串转换为字节数组
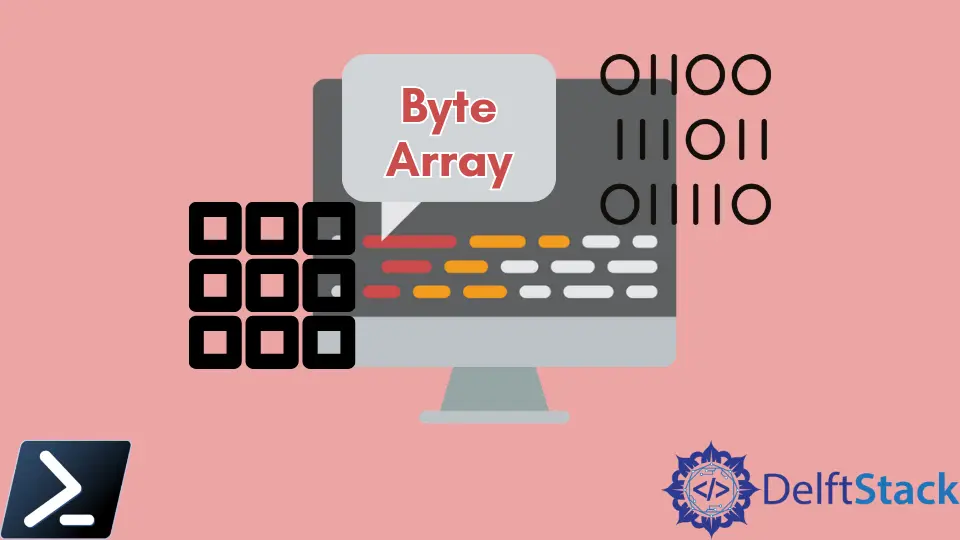
本文旨在展示如何使用 PowerShell 脚本 API 将以各种形式存在的数据转换为字节数组。
在 PowerShell 中将数据转换为字节数组
在自动化任务和处理数据时,数据可能需要以特定方式处理以提取有用信息或以有意义的方式修改数据。在 PowerShell 中处理这些数据有时会变得不便,尤其是在不同的数据类型中。
为了使处理更简单或减少处理计算的数量,在某些情况下,将数据转换为字节数组然后在其上执行操作而不是原始数据可能是理想的。
请考虑以下代码:
$data = Get-Content "a.exe"
Get-Item "a.exe" | Format-List -Property * -Force
Write-Host "File Datatype: "$data.GetType().Name
这将产生以下输出:
< Output redacted >
.
.
.
.
BaseName : a
Target :
LinkType :
Length : 400644
.
.
.
Extension : .exe
Name : a.exe
Exists : True
.
.
.
.
.
Attributes : Archive
File Datatype: Object[]
从上述输出中,我们打开的文件是一个 Windows 可执行文件(exe
文件)。在使用 Get-Item
和 Format-List
命令后,关于该文件,尤其是大小,可以获得有意义的见解,这在迭代过程中可能非常有用。
这种情况的一个不利方面是,通过使用 Get-Content
命令检索的文件数据返回的是 Object[]
。
让我们看看如何将其转换为 Byte[]
以进行场景依赖的操作。
在 PowerShell 5+ 中将数据转换为字节数组
请考虑以下代码:
$file = "a.exe"
[byte[]]$data = Get-Content $file -Encoding Byte
Write-Host "File Datatype: "$data.GetType().Name
for ($i = 0; $i -lt 10; $i++) { Write-Host $data[$i] }
这将产生以下输出:
File Datatype: Byte[]
Byte No 0 : 77
Byte No 1 : 90
Byte No 2 : 144
Byte No 3 : 0
Byte No 4 : 3
Byte No 5 : 0
Byte No 6 : 0
Byte No 7 : 0
Byte No 8 : 4
Byte No 9 : 0
Byte No 10 : 0
通过类型转换和 -Encoding
参数,可以直接将文件读取为字节数组。我们可以通过使用 GetType()
方法并访问名为 Name
的属性来验证结果确实是字节数组。
为了进一步验证数据确实转换正确,我们可以写一个小的 for
循环并打印文件中的一些字节。
在 PowerShell 7+ 中将数据转换为字节数组
请考虑以下代码:
[byte[]]$data = Get-Content "a.exe" -AsByteStream
Write-Host $data.GetType().Name
这将产生以下输出:
textByte[]
大多数语法在不同版本之间是相同的。唯一的区别是 -Encoding Byte
被替换为 -AsByteStream
。
在 PowerShell 中将字节数组转换为字符串
将任何给定的字节数组转换为字符串是简单的。请考虑以下代码:
$array = @(0x54, 0x68, 0x65, 0x20, 0x6f, 0x62, 0x6a, 0x65, 0x63, 0x74, 0x20, 0x6f, 0x66, 0x20, 0x6c, 0x69, 0x66, 0x65, 0x20, 0x69, 0x73, 0x20, 0x6e, 0x6f, 0x74, 0x20, 0x74, 0x6f, 0x20, 0x62, 0x65, 0x20, 0x6f, 0x6e, 0x20, 0x74, 0x68, 0x65, 0x20, 0x73, 0x69, 0x64, 0x65, 0x20, 0x6f, 0x66, 0x20, 0x74, 0x68, 0x65, 0x20, 0x6d, 0x61, 0x6a, 0x6f, 0x72, 0x69, 0x74, 0x79, 0x2c, 0x20, 0x62, 0x75, 0x74, 0x20, 0x74, 0x6f, 0x20, 0x65, 0x73, 0x63, 0x61, 0x70, 0x65, 0x20, 0x66, 0x69, 0x6e, 0x64, 0x69, 0x6e, 0x67, 0x20, 0x6f, 0x6e, 0x65, 0x73, 0x65, 0x6c, 0x66, 0x20, 0x69, 0x6e, 0x20, 0x74, 0x68, 0x65, 0x20, 0x72, 0x61, 0x6e, 0x6b, 0x73, 0x20, 0x6f, 0x66, 0x20, 0x74, 0x68, 0x65, 0x20, 0x69, 0x6e, 0x73, 0x61, 0x6e, 0x65, 0x2e)
$string = [System.Text.Encoding]::UTF8.GetString($array)
$string
这将产生以下输出:
The object of life is not to be on the side of the majority but to escape finding oneself in the ranks of the insane.
使用 UTF8.GetString
方法,我们可以将任何 UTF8 编码的字节数组转换回其字符串表示。务必注意文本的编码,因为在其他编码(如 ASCII)上使用此方法可能会产生异常结果。
在 PowerShell 中将字符串转换为字节数组
类似于字节数组可以转换为其字符串表示,字符串也可以转换为其字节表示。
请考虑以下代码:
$string = "Never esteem anything as of advantage to you that will make you break your word or lose your self-respect."
$bytes = [System.Text.Encoding]::Unicode.GetBytes($string)
Write-Host "First 10 Bytes of String are: "
for ($i = 0; $i -lt 10; $i++) { Write-Host $bytes[$i] }
这将产生输出:
First 10 Bytes of String are:
78
0
101
0
118
0
101
0
114
0
在这种特定情况下,可以使用 Unicode.GetBytes
来获取 Unicode 字符串的字节。确保字符串是 Unicode;否则,转换可能导致重要数据的丢失。
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn