在 Windows PowerShell 中获取命令行参数
- 在 PowerShell 脚本中定义参数
- PowerShell 脚本中的命名参数
- 为 PowerShell 脚本中的参数分配默认值
- PowerShell 脚本中的切换参数
- PowerShell 脚本中的强制参数
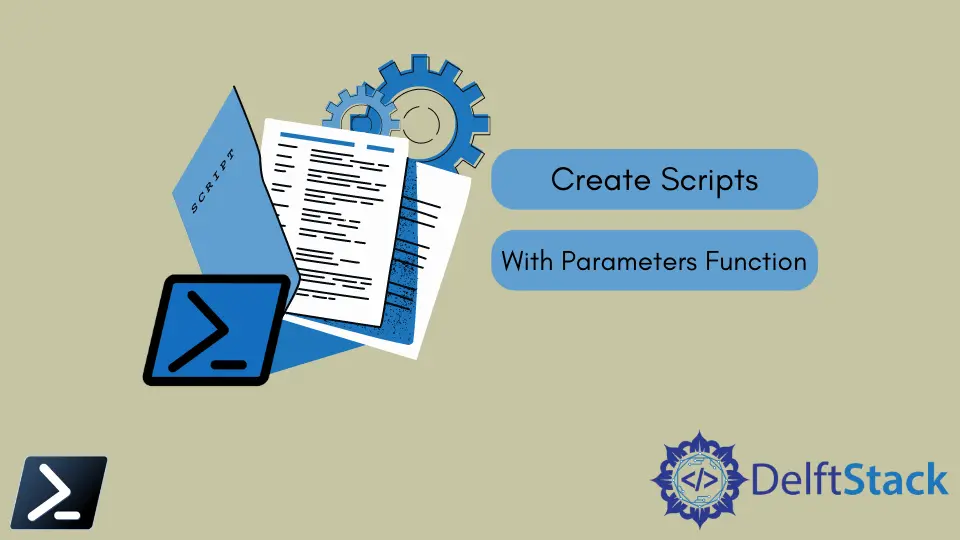
我们使用称为 param()
的 Windows PowerShell 参数函数处理参数。Windows PowerShell 参数函数是任何脚本的基本组件。一个参数是开发人员如何让脚本用户在运行时提供输入。
在本文中,你将学习如何使用参数函数创建脚本、使用它们以及构建参数的一些最佳实践。
在 PowerShell 脚本中定义参数
管理员可以使用参数函数 param()
为脚本和函数创建参数。一个函数包含一个或多个由变量定义的参数。
Hello_World.ps1
:
param(
$message
)
但是,为了确保参数只接受你需要的输入类型,最佳实践规定使用参数块 [Parameter()]
为参数分配数据类型,并在变量前用方括号 []
括住数据类型。
Hello_World.ps1
:
param(
[Parameter()]
[String]$message
)
在上面的示例 Hello_World.ps1
中,变量 message
仅在给定值具有数据类型 String
时才接受传递的值。
PowerShell 脚本中的命名参数
在脚本中使用参数函数的一种方法是通过参数名称——这种方法称为命名参数。通过命名参数调用脚本或函数时,我们使用变量名作为参数的全名。
在这个例子中,我们创建了一个 Hello_World.ps1
并在参数函数中定义了变量。请记住,我们可以在参数函数中放置一个或多个变量。
Hello_World.ps1
:
param(
[Parameter()]
[String]$message,
[String]$emotion
)
Write-Output $message
Write-Output "I am $emotion"
然后,我们可以在执行 .ps1
文件时使用命名参数作为参数。
.\Hello_World.ps1 -message 'Hello World!' -emotion 'happy'
输出:
Hello World!
I am happy
为 PowerShell 脚本中的参数分配默认值
我们可以通过在脚本中给参数一个值来为参数预分配一个值。然后,在不从执行行传递值的情况下运行脚本将采用脚本内定义的变量的默认值。
param(
[Parameter()]
[String]$message = "Hello World",
[String]$emotion = "happy"
)
Write-Output $message
Write-Output "I am $emotion"
.\Hello_World.ps1
输出:
Hello World!
I am happy
PowerShell 脚本中的切换参数
我们可以使用的另一个参数是 [switch]
数据类型定义的 switch 参数。switch 参数用于二进制或布尔值以指示 true
或 false
。
Hello_World.ps1
:
param(
[Parameter()]
[String]$message,
[String]$emotion,
[Switch]$display
)
if($display){
Write-Output $message
Write-Output "I am $emotion"
}else{
Write-Output "User denied confirmation."
}
我们可以使用下面的语法使用 script 参数调用我们的脚本。
.\Hello_World.ps1 -message 'Hello World!' -emotion 'happy' -display:$false
输出:
User denied confirmation.
PowerShell 脚本中的强制参数
执行脚本时必须使用一个或多个参数是很常见的。我们可以通过在参数块 [Parameter()]
中添加 Mandatory
属性来使参数成为强制性参数。
Hello_World.ps1:
param(
[Parameter(Mandatory)]
[String]$message,
[String]$emotion,
[Switch]$display
)
if($display){
Write-Output $message
Write-Output "I am $emotion"
}else{
Write-Output "User denied confirmation."
}
执行 Hello_World.ps1
时,Windows PowerShell 将不允许脚本运行并提示你输入值。
.\Hello_World.ps1
输出:
cmdlet hello_world.ps1 at command pipeline position 1
Supply values for the following parameters:
message:
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn