如何使用 PowerShell 检查字符串的开头
-
使用
-like
逻辑操作符检查 PowerShell 中字符串的开头 -
使用
-clike
逻辑操作符检查 PowerShell 中字符串的开头 -
使用
StartsWith()
函数检查 PowerShell 中字符串的开头 - 使用子字符串比较检查 PowerShell 中字符串的开头
- 结论
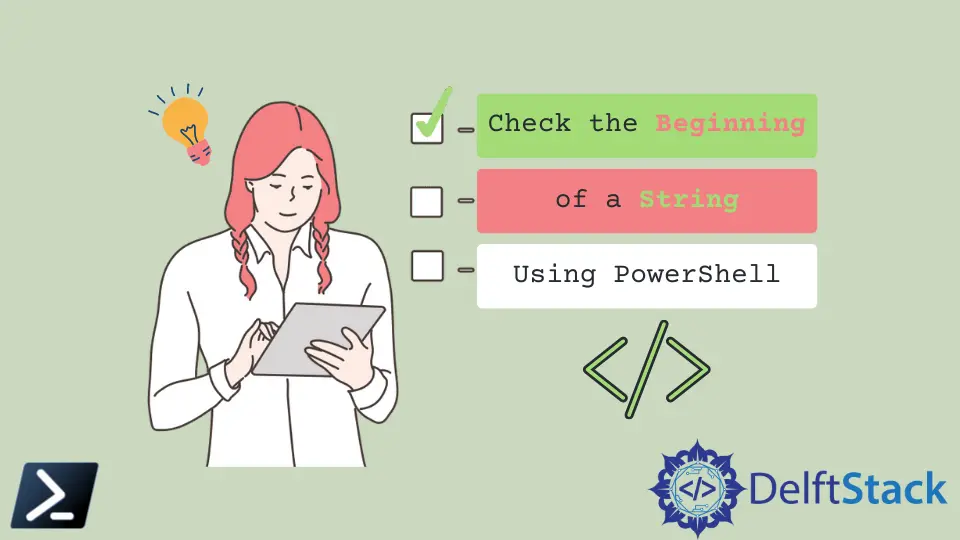
可能会出现我们遇到用例的情况,其中可能需要检查字符串变量是否以某个字符或字符串开头。检查字符串是否以特定字符或字符串开头是在编写脚本时的常见做法,并且在 Windows PowerShell 中编写时也相对简单。
本文将演示如何使用 Windows PowerShell 中的不同方法检查字符串变量的开头。
使用 -like
逻辑操作符检查 PowerShell 中字符串的开头
在 PowerShell 中,-Like
逻辑操作符是一个强大的模式匹配工具。当用于检查字符串的开头时,它允许基于通配符进行灵活比较。
默认情况下,-Like
操作符忽略区分大小写的声明。然而,如果我们使用逻辑操作符,则必须与星号通配符(*
)结合使用。
代码:
$strVal = 'Hello World'
if ($strVal -like 'hello*') {
Write-Host "Your string starts with hello."
}
else {
Write-Host "Your string does not start with hello."
}
输出:
Your string starts with hello.
在代码中,我们初始化一个字符串变量,$strVal = 'Hello World'
。然后我们使用 -like
操作符检查字符串($strVal
)是否以前缀 'hello'
开头。
-like
操作符允许使用通配符进行模式匹配,在这种情况下,星号(*
)代表零个或多个字符。条件语句评估字符串是否与模式 'hello*'
匹配。
在这种情况下,条件为真,输出为 Your string starts with hello
。
使用 -clike
逻辑操作符检查 PowerShell 中字符串的开头
PowerShell 中的 -clike
操作符用于执行区分大小写的字符串比较。它检查字符串是否与指定模式匹配,类似于 -like
操作符,但它是区分大小写的。
这意味着 -clike
操作符仅在字符串中的字符的大小写与模式中的字符的大小写完全匹配时才会返回 True
。我们可以使用 -cLike
操作符进行区分大小写的比较。
代码:
$strVal = 'Hello World!'
if ($strVal -clike 'h*') {
Write-Host "Your string starts with lowercase h."
}
else {
Write-Host "Your string starts with uppercase H."
}
输出:
Your string starts with uppercase H.
在这段代码中,我们初始化一个字符串变量 $strVal
,其值为 'Hello World!'
。我们使用 -clike
操作符,进行区分大小写的字符串比较,以检查字符串是否以小写字母 'h'
开头。
条件语句评估字符串是否以区分大小写的方式符合模式 'h*'
。由于条件为假,表示字符串以大写 'H'
开头,我们输出 Your string starts with uppercase H
。
请记住,-clike
操作符是区分大小写的,因此如果字符的大小写不完全匹配,它将不匹配字符串。如果我们想进行不区分大小写的比较,可以改用 -like
操作符。
使用 StartsWith()
函数检查 PowerShell 中字符串的开头
我们还可以使用.NET 框架的字符串扩展函数 StartsWith()
来检查字符串是否以一组字符开头。
PowerShell 中的 StartsWith()
函数是验证字符串是否以指定前缀开头的方法。StartsWith()
函数是 PowerShell 中字符串的内置方法,返回一个布尔值,指示给定字符串是否以指定子字符串开头。
代码:
$strVal = 'Hello World!'
if ($strVal.StartsWith('Hello')) {
Write-Host 'Your string starts with hello.'
}
else {
Write-Host 'Your string does not start with hello.'
}
输出:
Your string starts with hello.
在上述代码中,我们使用 StartsWith()
方法检查字符串变量 $strVal
是否以 'Hello'
开头。由于条件为真,我们输出 Your string starts with hello
。
StartsWith
函数还接受另一个参数,我们可以用来检查区分大小写的字符。这个参数是 CurrentCultureIgnoreCase
。
如果我们想进行区分大小写的比较,我们将使用以下方法。
代码:
$strVal = 'Hello world'
if ($strVal.StartsWith('hello', 'CurrentCultureIgnoreCase')) {
Write-Host 'True'
}
else {
Write-Host 'False'
}
输出:
True
在这段代码中,我们在不区分大小写的情况下检查字符串变量 $strVal
是否以 'hello'
开头,使用了带有 'CurrentCultureIgnoreCase'
参数的 StartsWith()
方法。由于条件为真,我们输出 True
。
使用子字符串比较检查 PowerShell 中字符串的开头
在这种方法中,使用 Substring()
函数将指定前缀与给定字符串的初始部分进行比较。这种比较有助于确定字符串是否以预定义的字符序列开头。
在 PowerShell 中,Substring 方法允许根据指定的起始索引和长度从字符串中提取部分内容。通过使用此方法,我们可以从原始字符串中获取子字符串,然后与所需的前缀进行比较,以检查字符串是否以该特定序列开头。
基本语法:
$substringToCompare = $strVal.Substring(0, $prefix.Length)
参数:
$strVal
- 这是保存我们要从中提取子字符串的原始字符串的变量。0
-0
是起始索引。$prefix.Length
- 这是要提取的子字符串的长度。在我们的示例中,它是我们想要检查的前缀的长度。$substringToCompare
- 这是一个变量,存储 Substring 操作的结果。它将包含从原始字符串提取的子字符串。
上面的语法本质上是从原始字符串中创建一个子字符串,从开头开始,其长度等于指定前缀的长度。
代码:
$strVal = 'Hello World!'
$prefix = 'Hello'
if ($strVal.Substring(0, $prefix.Length) -eq $prefix) {
Write-Output "String starts with $prefix"
}
else {
Write-Output "String does not start with $prefix"
}
输出:
String starts with Hello
在这段代码中,我们有一个字符串变量 $strVal = 'Hello World!'
和一个前缀 $prefix = 'Hello'
。我们使用 Substring()
方法从原始字符串中提取一个部分,从索引 0
开始,长度与前缀的长度相同。
然后脚本使用 -eq
操作符将提取的子字符串与指定的前缀进行比较。由于条件为真,表明字符串以 'Hello'
开头,因此我们输出 String starts with Hello
。
结论
总之,本文演示了在 Windows PowerShell 中检查字符串变量开头的各种方法。我们探索了使用 -like
逻辑操作符进行模式匹配,展示了它与通配符的灵活性。
此外,我们深入讨论了 -clike
逻辑操作符进行区分大小写的比较。此外,我们还介绍了 StartsWith()
函数,利用区分大小写和不区分大小写的检查来确定字符串是否以指定前缀开头。
最后,我们检查了 Substring()
函数作为替代方法,说明了其在与预定义序列比较子字符串时的应用,并根据评估输出结果。这些方法为不同的用例提供了灵活性,增强了 PowerShell 脚本中的字符串比较能力。
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn