PHP 中如何检查字符串是否以指定字符串开头
Minahil Noor
2023年1月30日
PHP
PHP String
-
使用
substr()
函数检查字符串是否以 PHP 中的指定字符串开头 -
使用
strpos()
函数检查字符串是否以 PHP 中的指定字符串开头 -
使用
strncmp()
函数检查字符串是否以 PHP 中的指定字符串开头
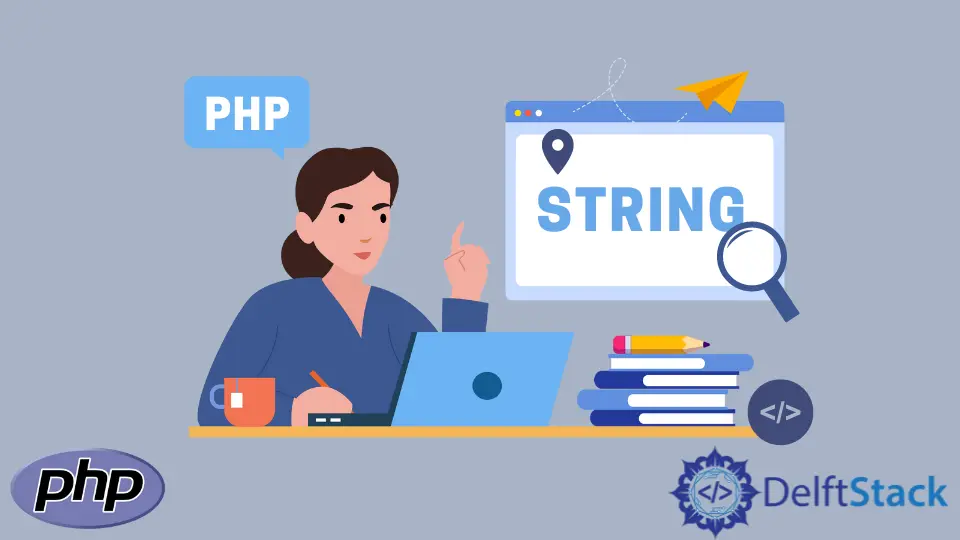
在本文中,我们将介绍检查 PHP 中字符串是否以指定的字符串开头的方法。
- 使用
substr()
函数 - 使用
strpos()
函数 - 使用
strncmp()
函数
使用 substr()
函数检查字符串是否以 PHP 中的指定字符串开头
内置函数 substr()
用于访问子字符串。传递字符串作为输入,并返回我们要访问的子字符串。我们可以使用这个函数来检查 string
是否以特定的字符串开头。使用此函数的正确语法如下
substr($string, $startPosition, $lengthOfSubstring);
该功能具有三个参数。其参数的详细信息如下。
参数 | 描述 | |
---|---|---|
$string |
强制的 | 我们希望访问其子字符串的原始字符串 |
$startPosition |
强制的 | 整数变量。它告诉我们子字符串开始的位置。如果为正,则我们的子字符串从字符串的左侧开始,即从开头开始。如果为负,则我们的子字符串从末尾开始。 |
$lengthOfSubstring |
可选的 | 整数变量。它从开始位置告诉字符串的总长度。如果省略,则返回从字符串的开始位置到结尾的子字符串。如果为负,则根据其值从末尾删除该字符串。如果为零,则返回一个空字符串。 |
<?php
$string = "Mr. Peter";
if(substr($string, 0, 3) === "Mr."){
echo "The string starts with the desired substring.";
}else
echo "The string does not start with the desired substring.";
?>
在上面的代码中,我们要检查我们的字符串是否以 Mr.
开头。
substr($string, 0, 3)
0
是子字符串的起始索引,换句话说,子字符串从给定字符串的第一个字符开始。
3 表示返回的子字符串的长度为 3。
如果字符串的开头与 Mr.
相同,则它将显示字符串以所需的子字符串开头。
输出:
The string starts with the desired substring.
使用 strpos()
函数检查字符串是否以 PHP 中的指定字符串开头
函数 strpos()
返回给定字符串中子字符串首次出现的位置。我们可以用它来检查字符串是否以指定的字符串开头。
如果返回值为 0
,则意味着给定的字符串以指定的子字符串开头。否则,字符串不能以检查的子字符串开头。
strpos()
是区分大小写的函数。使用此函数的正确语法如下。
strpos($string, $searchString, $startPosition);
它具有三个参数。其参数的详细信息如下。
参数 | 描述 | |
---|---|---|
$string |
强制的 | 这是我们希望找到其子字符串的字符串 |
$searchString |
强制的 | 它是将在字符串中搜索的子字符串 |
$startPosition |
可选的 | 它是字符串从搜索开始的位置 |
<?php
$string = "Mr. Peter";
if(strpos( $string, "Mr." ) === 0){
echo "The string starts with the desired substring.";
}else
echo "The string does not start with the desired substring.";
?>
在这里,我们通过查找第一次出现的先生来检查字符串是否以 Mr.
开头。
输出:
The string starts with the desired substring.
使用 strncmp()
函数检查字符串是否以 PHP 中的指定字符串开头
内置函数 strncmp()
比较两个给定的字符串。此函数也区分大小写。使用此函数的正确语法如下。
strncmp($string1, $string2, $length);
它具有三个参数。其参数的详细信息如下。
参数 | 描述 | |
---|---|---|
$string1 |
强制的 | 这是要比较的第一个字符串 |
$string2 |
强制的 | 这是要比较的第二个字符串 |
$length |
强制的 | 它是要比较的字符串的长度 |
如果两个字符串相等,则返回零。这是区分大小写的功能。
<?php
$string = "Mr. Peter";
if(strncmp($string, "Mr.", 3) === 0){
echo "The string starts with the desired substring.";
}else
echo "The string does not start with the desired substring.";
?>
在这里,比较两个字符串。要比较的字符串的长度为 3
。
输出:
The string starts with the desired substring.
strncmp 的不区分大小写的版本是 strncasecmp
。它比较给定两个字符串的前 n 个字符,而不管它们的大小写如何。
<?php
$string = "mr. Peter";
if(strncasecmp($string, "Mr.", 3) === 0){
echo "The string starts with the desired substring.";
}else
echo "The string does not start with the desired substring.";
?>
输出:
The string starts with the desired substring.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe