MongoDB 从数组中删除元素
- MongoDB 从数组中删除元素
-
使用 MongoDB
$pull
从文档数组中删除元素 -
使用 MongoDB
$pullAll
从数组中删除元素 -
使用 MongoDB
$pop
从数组中删除元素 -
使用 MongoDB
$unset
删除包含给定元素的字段 -
使用 MongoDB 位置运算符将元素设置为
null
而不是删除
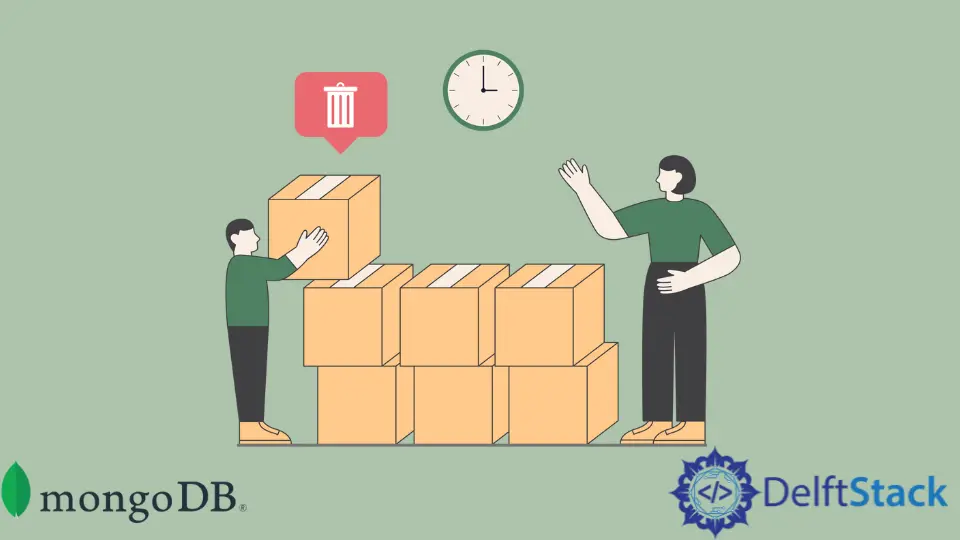
我们可以根据项目要求采用不同的方法。今天,我们将学习如何在工作时使用 $pull
、$pullAll
、$pop
、$unset
和位置运算符 ($
) 从数组或文档数组中删除元素在 MongoDB 中。
MongoDB 从数组中删除元素
我们必须有一个示例文档集合来练习所有提到的方法。我们将创建一个名为 collection
的集合并仅插入一个文档。
你也可以使用下面的 collection
,其中包含一个文档。
示例代码:
// MongoDB version 5.0.8
> db.collection.insertMany([
{
contact: {
phone: [
{"type" : "UAN", "number" : "123456789"},
{"type" : "office", "number" : "987546321"},
{"type" : "personal", "number" : "852147963"}
]
},
services: ["Google", "Instagram", "Twitter", "Facebook"],
scores: [ 0, 1, 8, 17, 18, 8, 20, 10 ]
},
{
contact: {
phone: [
{"type" : "UAN", "number" : "456321879"},
{"type" : "office", "number" : "874596321"},
{"type" : "personal", "number" : "964785123"}
]
},
services: ["Google", "Instagram", "Twitter"],
scores: [ 1, 8, 2, 3, 8, 7, 1 ]
}
]);
如果你没有名为 collection
的集合,请不要担心;上面的查询将为你创建并填充它。接下来,你可以运行 db.collection.find().pretty()
来查看插入的文档。
请记住,在执行接下来的代码示例后,你将看不到更新的文档。为此,你必须执行 db.collection.find().pretty()
命令。
使用 MongoDB $pull
从文档数组中删除元素
示例代码:
// MongoDB version 5.0.8
> db.collection.update(
{ _id : ObjectId("62aae796f27219958a489b89") },
{ $pull : { "contact.phone": { "number": "987546321" } } }
);
输出:
{
"_id" : ObjectId("62aae796f27219958a489b89"),
"contact" : {
"phone" : [
{ "type" : "UAN", "number" : "123456789" },
{ "type" : "personal", "number" : "852147963" }
]
},
"services" : [ "Google", "Instagram", "Twitter", "Facebook" ],
"scores" : [ 0, 1, 8, 17, 18, 8, 20, 10 ]
}
{
"_id" : ObjectId("62aae796f27219958a489b8a"),
"contact" : {
"phone" : [
{ "type" : "UAN", "number" : "456321879" },
{ "type" : "office", "number" : "874596321" },
{ "type" : "personal", "number" : "964785123" }
]
},
"services" : [ "Google", "Instagram", "Twitter" ],
"scores" : [ 1, 8, 2, 3, 8, 7, 1 ]
}
在这里,我们使用 $pull
运算符从现有数组中删除与过滤器查询或指定条件匹配的值/值的所有实例。
在上面的代码示例中,我们正在搜索 _id
等于 ObjectId("62aae796f27219958a489b89")
的文档。一旦文档符合指定条件,我们就从现有数组中删除实例,其中 contact.phone.number
等于 987546321
。
使用 $pull
运算符时请记住以下几点。
- 如果需要删除的给定
value
是一个数组,则$pull
运算符仅从完全满足给定value
的数组中删除元素(包括顺序)。 - 如果需要删除的给定
值
是文档,则$pull
运算符仅从包含确切字段和值的数组中删除元素。字段的顺序在此处可能有所不同。 - 如果给定的
条件
和数组的元素是嵌入文档,则$pull
运算符将应用条件
,就好像每个数组元素都是集合中的文档一样。有关这方面的更多详细信息,你可以查看 this。
现在,我们有另一种情况,我们想要对集合中的所有文档执行 remove
操作。为此,我们使用 $pull
使用以下语法,而 {multi: true}
对指定条件的所有文档进行操作。
示例代码:
// MongoDB version 5.0.8
> db.collection.update({},
{ $pull: { "contact.phone": { "type": "UAN" } } },
{ multi: true }
);
输出:
{
"_id" : ObjectId("62aae796f27219958a489b89"),
"contact" : {
"phone" : [
{ "type" : "personal", "number" : "852147963" }
]
},
"services" : [ "Google", "Instagram", "Twitter", "Facebook" ],
"scores" : [ 0, 1, 8, 17, 18, 8, 20, 10 ]
}
{
"_id" : ObjectId("62aae796f27219958a489b8a"),
"contact" : {
"phone" : [
{ "type" : "office", "number" : "874596321" },
{ "type" : "personal", "number" : "964785123" }
]
},
"services" : [ "Google", "Instagram", "Twitter" ],
"scores" : [ 1, 8, 2, 3, 8, 7, 1 ]
}
为了获得上述输出,我们从集合中的所有文档中删除数组元素,其中 contact.phone.type
等于 "UAN"
。
我们的下一个任务是从 scores
数组中删除值大于或等于 2
且小于或等于 6
(2 <= value <= 6
) 的元素。对所选集合的所有文档执行以下查询。
示例代码:
// MongoDB version 5.0.8
> db.collection.update(
{},
{ $pull : { scores : {$gte: 2, $lte: 6} } },
{ multi: true }
)
输出:
{
"_id" : ObjectId("62aae796f27219958a489b89"),
"contact" : {
"phone" : [
{ "type" : "personal", "number" : "852147963" }
]
},
"services" : [ "Google", "Instagram", "Twitter", "Facebook" ],
"scores" : [ 0, 1, 8, 17, 18, 8, 20, 10 ]
}
{
"_id" : ObjectId("62aae796f27219958a489b8a"),
"contact" : {
"phone" : [
{ "type" : "office", "number" : "874596321" },
{ "type" : "personal", "number" : "964785123" }
]
},
"services" : [ "Google", "Instagram", "Twitter" ],
"scores" : [ 1, 8, 8, 7, 1 ]
}
要练习使用字符串类型的值,我们可以通过以下方式进行。
示例代码:
// MongoDB version 5.0.8
> db.collection.update(
{},
{ $pull: { "services": { $in: [ "Google", "Instagram" ] }, "scores": 1 } },
{ multi: true }
)
输出:
{
"_id" : ObjectId("62aae796f27219958a489b89"),
"contact" : {
"phone" : [
{ "type" : "personal", "number" : "852147963" }
]
},
"services" : [ "Twitter", "Facebook" ],
"scores" : [ 0, 8, 17, 18, 8, 20, 10 ]
}
{
"_id" : ObjectId("62aae796f27219958a489b8a"),
"contact" : {
"phone" : [
{ "type" : "office", "number" : "874596321" },
{ "type" : "personal", "number" : "964785123" }
]
},
"services" : [ "Twitter" ],
"scores" : [ 8, 8, 7 ]
}
对于此代码示例,我们使用 $in
比较运算符来获取一个值数组,如果存在任何值,则将它们从 services
数组中删除。
此外,当我们使用 $pull
运算符执行所有这些删除操作时,我们从 scores
数组中删除 1
如果它在那里。
使用 MongoDB $pullAll
从数组中删除元素
示例代码:
// MongoDB version 5.0.8
> db.collection.update(
{ _id: ObjectId("62aae796f27219958a489b8a") },
{ $pullAll: { "scores": [8] } }
)
输出:
{
"_id" : ObjectId("62aae796f27219958a489b89"),
"contact" : {
"phone" : [
{ "type" : "personal", "number" : "852147963" }
]
},
"services" : [ "Twitter", "Facebook" ],
"scores" : [ 0, 8, 17, 18, 8, 20, 10 ]
}
{
"_id" : ObjectId("62aae796f27219958a489b8a"),
"contact" : {
"phone" : [
{ "type" : "office", "number" : "874596321" },
{ "type" : "personal", "number" : "964785123" }
]
},
"services" : [ "Twitter" ],
"scores" : [ 7 ]
}
对于此代码,我们使用 $pullAll
运算符从现有数组中删除给定值的所有出现(实例)。例如,如果数字 8
在 scores
数组中出现两次,则两个 8
值都将被删除。
就像 $pull
运算符通过指定过滤查询来删除数组元素一样,$pullAll
删除/删除与列出的值匹配的数组元素。
我们使用点符号来处理数组的嵌入文档。
使用 MongoDB $pop
从数组中删除元素
如果我们有兴趣从数组中删除第一个或最后一个元素,我们可以使用 $pop
运算符。为此,我们将 -1
或 1
传递给 $pop
运算符以从第一个或最后一个删除数组元素。
示例代码:
// MongoDB version 5.0.8
> db.collection.update(
{ _id: ObjectId("62aae796f27219958a489b89") },
{ $pop: { "scores": -1 } }
)
输出:
{
"_id" : ObjectId("62aae796f27219958a489b89"),
"contact" : {
"phone" : [
{ "type" : "personal", "number" : "852147963" }
]
},
"services" : [ "Twitter", "Facebook" ],
"scores" : [ 8, 17, 18, 8, 20, 10 ]
}
{
"_id" : ObjectId("62aae796f27219958a489b8a"),
"contact" : {
"phone" : [
{ "type" : "office", "number" : "874596321" },
{ "type" : "personal", "number" : "964785123" }
]
},
"services" : [ "Twitter" ],
"scores" : [ 7 ]
}
使用 MongoDB $unset
删除包含给定元素的字段
示例代码:
// MongoDB version 5.0.8
> db.collection.update(
{ _id : ObjectId("62aae796f27219958a489b89") },
{ $unset : {"scores" : 8 }}
)
输出:
{
"_id" : ObjectId("62aae796f27219958a489b89"),
"contact" : {
"phone" : [
{ "type" : "personal", "number" : "852147963" }
]
},
"services" : [ "Twitter", "Facebook" ]
}
{
"_id" : ObjectId("62aae796f27219958a489b8a"),
"contact" : {
"phone" : [
{ "type" : "office", "number" : "874596321" },
{ "type" : "personal", "number" : "964785123" }
]
},
"services" : [ "Twitter" ],
"scores" : [ 7 ]
}
在这里,我们使用 $unset
运算符来删除包含给定值的特定字段。例如,我们正在删除匹配过滤器查询并包含 8
作为数组元素的 scores
字段。
请记住,当我们将 $unset
运算符与位置运算符 ($
) 一起使用时,它不会删除匹配的元素,而是将其设置为 null
。请参阅以下部分进行练习。
使用 MongoDB 位置运算符将元素设置为 null
而不是删除
示例代码:
// MongoDB version 5.0.8
> db.collection.update(
{"services": "Facebook"},
{ $unset: {"services.$" : 1}}
)
输出:
{
"_id" : ObjectId("62aae796f27219958a489b89"),
"contact" : {
"phone" : [
{ "type" : "personal", "number" : "852147963" }
]
},
"services" : [ "Twitter", null ]
}
{
"_id" : ObjectId("62aae796f27219958a489b8a"),
"contact" : {
"phone" : [
{ "type" : "office", "number" : "874596321" },
{ "type" : "personal", "number" : "964785123" }
]
},
"services" : [ "Twitter" ],
"scores" : [ 7 ]
}
此代码片段使用 $unset
和位置运算符将数组元素设置为 null
而不是删除它。我们使用 $
来更新数组的第一个元素,其中 services
数组中的值为 Facebook
。
当我们不知道数组元素的位置时,可以使用这种方法。另一方面,如果我们想更新给定数组字段中的所有元素,我们也可以使用 $[]
(所有位置运算符)。