JavaScript 重新加载 DIV
-
在
.load()
中使用window.location.href
在 JavaScript 中重新加载div
-
使用
" #id > *"
和.load()
在 JavaScript 中重新加载div
-
使用
window.setInterval()
在 JavaScript 中刷新div
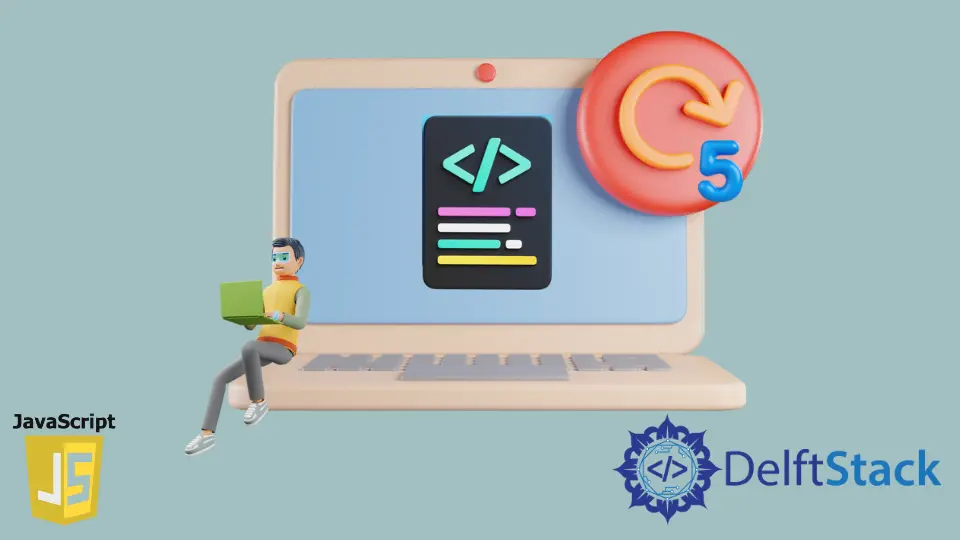
在 JavaScript 中找到一个单行编码约定来重新加载 div
元素是很困难的。重新加载或刷新的一般方法是调整 onload
功能或触发整个网页。
如果网页很大,这可能并不总是首选;更有可能的是,我们想要一种敏捷的方式来刷新某些部分。
为了解决这个问题,我们将完全依赖 jQuery .load()
函数,该函数获取网页的当前路径并添加 div
的 id。这是为了确保我们会在网页内容中专门添加 id
来表示 div
的位置并刷新它。
我们将遵循的另一种方法是在我们希望刷新 div
之后跟踪时间限制。在这种情况下,我们将需要 HTML 中的简单动态属性来与用户定义的时间限制协作。
这可能看起来不像 jQuery 的 .load()
那样专用,但通过 JavaScript 处理它相对容易。让我们跳到代码块上。
在 .load()
中使用 window.location.href
在 JavaScript 中重新加载 div
本例中最重要的部分是 id
属性,它将采用目标 div
实例。我们将使用 div
加载网页的那一部分,而不是整个网页。
最初,我们将有一个带有 span
标签的 div
来计算时间范围(检查它是否工作正常)。稍后我们将使用 div
(#here
) 的实例来使用 load()
函数。
在 .load()
函数中,我们的参数将是 window.location.href
,它指的是当前网站的路径。与 #here
相关的 id
将分配目的地并刷新它。
代码片段:
<script src="https://code.jquery.com/jquery-3.6.0.min.js" integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4=" crossorigin="anonymous"></script>
<div>
<div id="here">dynamic content <span id='off'>9</span></div>
<div>ABC</div>
</div>
<script>
$(document).ready(function(){
var counter = 9;
window.setInterval(function(){
counter = counter - 3;
if(counter>=0){
document.getElementById('off').innerHTML=counter;
}
if (counter===0){
counter=9;
}
$("#here").load(window.location.href + " #here" );
}, 3000);
});
</script>
输出:
在这里,我们的计数器每 3 秒更改一次。由于屏幕抓取,时间比可以至少为 3 秒,但它确实推断该进程正在运行。
使用 " #id > *"
和 .load()
在 JavaScript 中重新加载 div
在本节中,我们将再次使用 .load()
函数,这次我们将传递 " #here > *"
作为参数。考虑在表示 #id
之前添加一个空格。
这整个部分将像解释的其他示例一样执行。
代码片段:
<script src="https://code.jquery.com/jquery-3.6.0.min.js" integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4=" crossorigin="anonymous"></script>
<div>
<div id="here"> again dynamic content <span id='off'>3</span></div>
<div>ABC</div>
</div>
<script>
$(document).ready(function(){
var counter = 3;
window.setInterval(function(){
counter--;
if(counter>=0){
document.getElementById('off').innerHTML=counter;
}
if (counter===0){
counter=3;
}
$("#here").load(" #here > *");
}, 1000);
});
</script>
输出:
使用 window.setInterval()
在 JavaScript 中刷新 div
在这里,我们将获取一个 div
并设置它的 id
属性。在脚本部分,我们将激活计数时钟。
这个例子是一个很好的例子,我们有一系列内容在一定次数后显示在网页上。假设我们有多个 div
要呈现,并且在每个完整的时间倒计时之后,我们将更改数组索引。
因此,我们将拥有处理许多内容的动态方式。
在下面的示例中,我们没有获取任何此类数组数据。相反,我们只是启动了时钟周期。让我们检查代码以获得更好的预览。
<div>
The DIV below will refresh after <span id="cnt" style="color:red;">7</span> Seconds
</div>
</body>
<script>
var counter = 7;
window.setInterval(function () {
counter--;
if (counter >= 0) {
var span;
span = document.getElementById("cnt");
span.innerHTML = counter;
}
if (counter === 0) {
counter = 7;
}
}, 1000);
</script>
输出: