Java 中抛出多个异常
Mohd Ebad Naqvi
2023年10月12日
Java
Java Exception
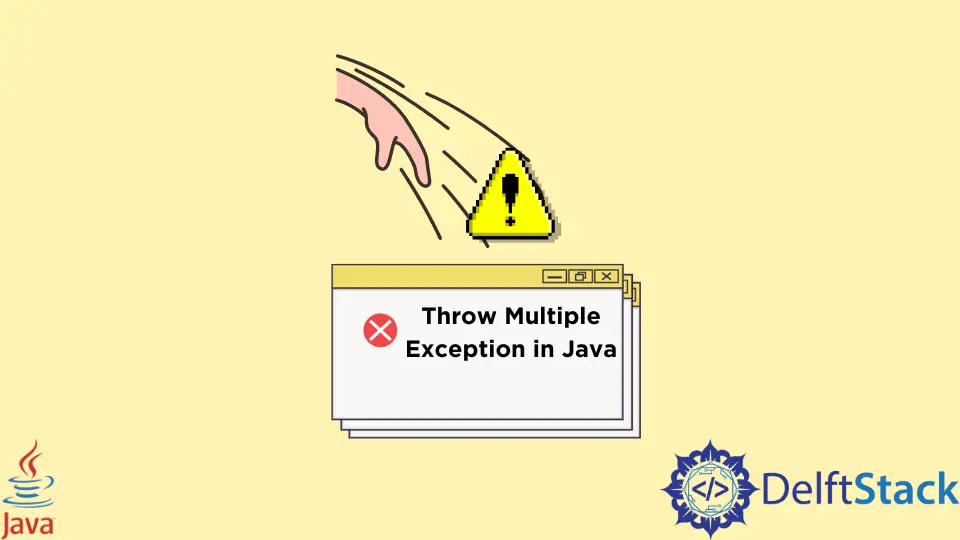
本教程将演示如何在 Java 中抛出多个异常。
异常是在程序执行期间破坏指令正常流程的不需要的和意外的事件。所有 Java 异常的根类都是 java.lang.Throwable
。此类由 Error 和异常子类继承。
为了处理异常,我们使用 try...catch
块。
在 try
块中,我们放置了可能引发一些异常的代码。try
块不是单独使用的。它必须始终跟在 catch
或 finally
之后。catch
块用于捕获异常。这个块可以跟在 finally 块之后。finally
块用于执行程序的重要代码,无论是否有异常。
在 Java 中,我们可能要处理多个异常。在 Java 中不可能抛出大量异常。我们可以指定多个异常,但只会抛出其中一个。
但是,我们有一些替代方法可以用来模拟抛出多个异常。
我们可以使用链式异常来处理多个异常。此类异常表明引发的异常是由另一个异常引起的。
例如,
public class Main {
public static void main(String[] args) {
try {
NumberFormatException ex = new NumberFormatException("NumberFormatException is thrown");
ex.initCause(new NullPointerException("NullPointerException is the main cause"));
throw ex;
}
catch (NumberFormatException ex) {
System.out.println(ex);
System.out.println(ex.getCause());
}
}
}
输出:
java.lang.NumberFormatException: NumberFormatException is raised
java.lang.NullPointerException: NullPointerException is the main cause
请注意 initCause()
和 getCause()
方法的使用。initCause()
函数将异常的原因设置为另一个异常,而 getCause()
将返回异常的原因,在我们的例子中是 NullPointerException
。
我们也可以使用抑制异常。在这里,我们将抑制的异常附加到主要异常。它是在 Java 7 中添加的。
为了附加被抑制的异常,我们使用 addSuppressed()
函数。我们将此函数与主要异常对象一起使用。
例如,
import java.io.*;
class Main {
public static void main(String[] args) throws Exception {
try {
Exception suppressed = new NumberFormatException();
Exception suppressed2 = new NullPointerException();
final Exception exe = new Exception();
exe.addSuppressed(suppressed);
exe.addSuppressed(suppressed2);
throw exe;
}
catch (Throwable e) {
Throwable[] suppExe = e.getSuppressed();
System.out.println("Suppressed:");
for (int i = 0; i < suppExe.length; i++) {
System.out.println(suppExe[i]);
}
}
}
}
输出:
Suppressed:
java.lang.NumberFormatException
java.lang.NullPointerException
在上面的示例中,我们将两个异常附加到主对象并打印了被抑制的异常。为了获得所有被抑制的异常,我们使用 getSuppressed()
函数。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe