在 Java 中读取 JSON 文件
Siddharth Swami
2023年10月12日
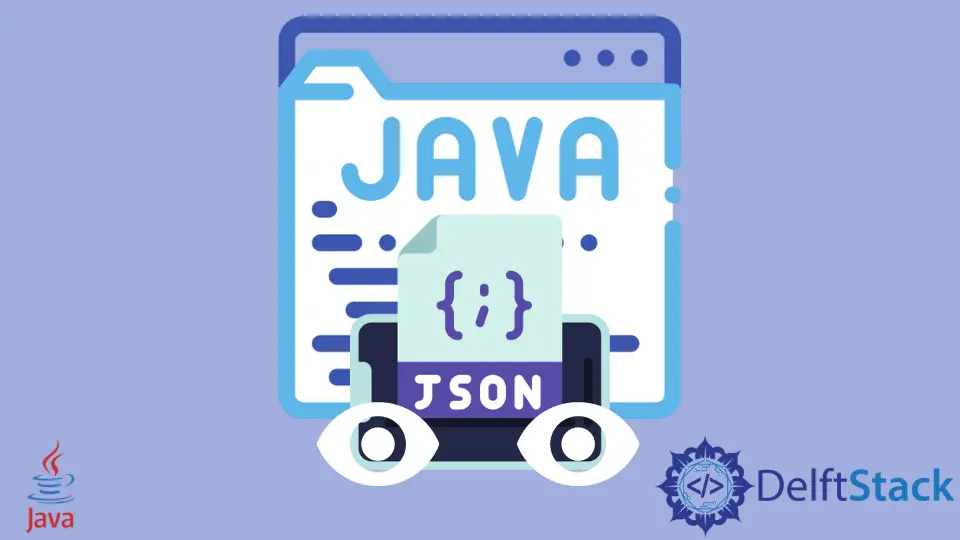
JavaScript Object Notation 是一种轻量级的基于文本的语言,用于存储和传输数据。对象和数组是由 JSON 文件表示的两种数据类型。
本教程演示了如何在 Java 中读取 JSON 文件。
要读取 JSON 文件,我们将使用 FileReader()
函数来启动一个 FileReader
对象并读取给定的 JSON 文件。
在我们的示例中,我们将读取以下文件。
{
"firstName": "Ram",
"lastName": "Sharma",
"age": 26
},
"phoneNumbers": [
{
"type": "home",
"phone-number": "212 888-2365"
}
]
}
为了解析这个文件的内容,我们将使用 json.simple
java 库。我们需要从 java.simple
库中导入两个类,org.json.simple.JSONArray
和 org.json.simple.JSONObject
类。JSONArray
帮助我们以数组的形式读取元素,而 JSONObject
帮助我们读取 JSON 文本中存在的对象。
在下面的代码中,我们读取了系统上已经存在的 JSON 文件,然后我们将打印文件中的名字和姓氏。
例如,
import org.json.simple.JSONArray;
import org.json.simple.JSONObject;
import org.json.simple.parser.*;
public class JSONsimple {
public static void main(String[] args) throws Exception {
// parsing file "JSONExample.json"
Object ob = new JSONParser().parse(new FileReader("JSONFile.json"));
// typecasting ob to JSONObject
JSONObject js = (JSONObject) ob;
String firstName = (String) js.get("firstName");
String lastName = (String) js.get("lastName");
System.out.println("First name is: " + firstName);
System.out.println("Last name is: " + lastName);
}
}
输出:
First name is: Ram
Last name is: Sharma
我们可以在上面的例子中看到我们从 JSON 文件中读取的名字和姓氏。
这里我们使用了 Java 中 org.json.simple.parser.*
类中的 JSONParser().parse()
,它解析文件中的 JSON 文本。此处的 js.get()
方法从文件中获取名字和姓氏。
我们可以使用不同的方法解析 JSON 内容,但使用 FileReader()
读取主文件。
我们也可以直接从字符串解析 JSON。还有其他方法可以解析 JSON 字符串。
下面的代码演示了 org.json
方法。在这里,我们将复制并粘贴静态字符串 json
中的完整文件。然后我们将创建一个对象,该对象将用于从文件中读取 JSON 对象和数组。
import org.json.JSONArray;
import org.json.JSONObject;
public class JSON2 {
static String json = "{\"contacDetails\": {\n" + // JSON text in the file is written here
" \"firstName\": \"Ram\",\n"
+ " \"lastName\": \"Sharma\"\n"
+ " },\n"
+ " \"phoneNumbers\": [\n"
+ " {\n"
+ " \"type\": \"home\",\n"
+ " \"phone-number\": \"212 888-2365\",\n"
+ " }\n"
+ " ]"
+ "}";
public static void main(String[] args) {
// Make a object
JSONObject ob = new JSONObject(json);
// getting first and last name
String firstName = ob.getJSONObject("contacDetails").getString("firstName");
String lastName = ob.getJSONObject("contacDetails").getString("lastName");
System.out.println("FirstName " + firstName);
System.out.println("LastName " + lastName);
// loop for printing the array as phoneNumber is stored as array.
JSONArray arr = obj.getJSONArray("phoneNumbers");
for (int i = 0; i < arr.length(); i++) {
String post_id = arr.getJSONObject(i).getString("phone-number");
System.out.println(post_id);
}
}
}
输出:
FirstName Ram
LastName Sharma
212 888-2365