Java 中一个文件中的多个类
Siddharth Swami
2023年10月12日
Java
Java Class
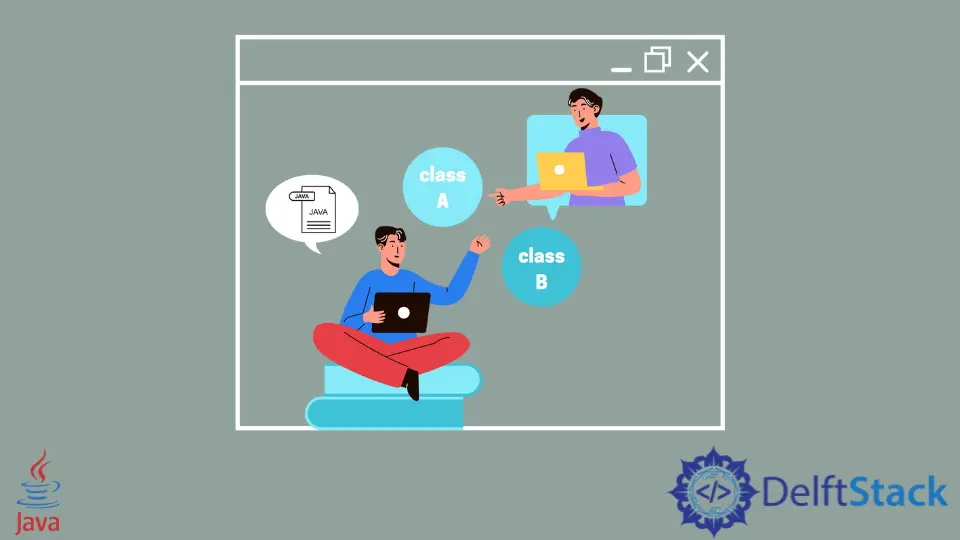
在本文中,我们将讨论 Java 中单个文件中的多个类。
有多种方法可以在一个文件中声明多个类。我们将讨论实现这一点的两种标准方法。
在 Java 中使用嵌套类在单个文件中包含多个类
在此方法中,类是在类中定义的。这种方法使我们能够对只在一个地方使用的类进行逻辑分组。
这些分为两类。首先是声明为 static
的静态嵌套类,另一个是非静态类型的内部类。
下面的代码演示了这种方法。
class Outer_Class {
// static member
static int x = 20;
// instance(non-static) member
int y = 20;
private static int outer_private = 40;
// static nested class
static class StaticNestedClass {
void display() {
System.out.println("outer_x = " + x);
System.out.println("outer_private = " + outer_private);
}
}
}
public class NestedDemo {
public static void main(String[] args) {
// accessing a static nested class
Outer_Class.StaticNestedClass nestedObject = new Outer_Class.StaticNestedClass();
nestedObject.display();
}
}
输出:
outer_x = 20
outer_private = 40
在 Java 中的单个文件中使用多个非嵌套类
我们还可以在 Java 的单个文件中拥有多个非嵌套类。
我们将通过以下示例更好地理解这一点。
public class Phone {
Phone() {
System.out.println("Constructor of phone class.");
}
void phone_method() {
System.out.println("Phone battery is low");
}
public static void main(String[] args) {
Phone p = new Phone();
Laptop l = new Laptop();
p.phone_method();
l.laptop_method();
}
}
class Laptop {
Laptop() {
System.out.println("Constructor of Laptop class.");
}
void laptop_method() {
System.out.println("Laptop battery is low");
}
}
输出:
Constructor of phone class.
Constructor of Laptop class.
Phone battery is low
Laptop battery is low
在上面的例子中,程序由两个类组成,第一个是 Phone
类,第二个是 Laptop
类。
这两个类都有构造函数和执行函数的方法。当我们运行我们的程序时,会为两个类创建两个 .class
文件。我们可以再次重用 .class
文件而无需重新编译代码。在输出中,我们可以看到我们的代码已成功执行。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe