在 Java 中将字节写入文件
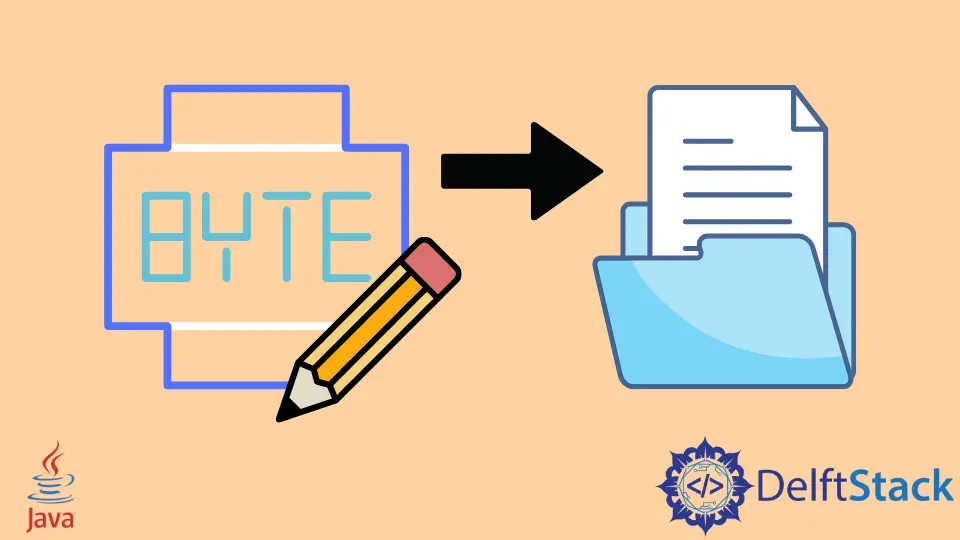
该程序演示了如何在 Java 中将字节数组写入文件。可以使用 FileOutputStream
和本文中提到的一些库来执行此任务。
在 Java 中使用 FileOutputStream
将字节写入文件
Java 中的类 FileOutputStream
是用于将数据或字节流写入文件的输出流。构造函数 FileOutputStream(File file)
创建一个文件输出流以写入由 File
对象 file
表示的文件,我们在下面的代码中创建了该文件。
String
类型的变量 s
被传递给 getBytes()
方法,该方法将字符串转换为字节序列并返回字节数组。write()
方法将字节数组作为参数,并将字节数组 b
中的 b.length 个字节写入此文件输出流。
在给定的路径上创建了一个扩展名为 .txt
的文件,如果我们打开它,我们可以看到与存储在变量 s
中的字符串相同的内容。
import java.io.File;
import java.io.FileOutputStream;
public class ByteToFile {
public static void main(String args[]) {
File file = new File("/Users/john/Desktop/demo.txt");
try {
FileOutputStream fout = new FileOutputStream(file);
String s = "Example of Java program to write Bytes using ByteStream.";
byte b[] = s.getBytes();
fout.write(b);
} catch (Exception e) {
e.printStackTrace();
}
}
}
使用 java.nio.file
将字节写入文件
Java NIO ( New I/O)
包由适用于文件、目录的静态方法组成,并且主要适用于 Path
对象。Path.get()
是一种将字符串序列或路径字符串转换为路径的静态方法。它只是调用 FileSystems.getDefault().getPath()
。
因此,我们可以使用 Files.write()
方法将字节数组 b
写入文件,方法是将路径传递给文件,并将字节数组转换为字符串。
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class ByteToFile {
public static void main(String args[]) {
Path p = Paths.get("/Users/john/Desktop/demo.txt");
try {
String s = "Write byte array to file using java.nio";
byte b[] = s.getBytes();
Files.write(p, b);
} catch (IOException ex) {
System.err.format("I/O Error when copying file");
}
}
}
在 Java 中使用 Apache Commons IO
库将字节写入文件
这个库的 maven 依赖如下。
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.6</version>
</dependency>
Apache Commons IO
库有 FilesUtils 类,它有 writeByteArrayToFile()
方法。此方法采用目标路径和我们正在写入的二进制数据。如果我们的目标目录或文件不存在,它们将被创建。如果文件存在,则在写入前将其截断。
import java.io.File;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import org.apache.commons.io.FileUtils;
public class ByteToFile {
public static void main(String args[]) {
{
File file = new File("doc.txt");
byte[] data =
"Here, we describe the general principles of photosynthesis"
.getBytes(StandardCharsets.UTF_8);
try {
FileUtils.writeByteArrayToFile(file, data);
System.out.println("Successfully written data to the file");
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
输出:
Successfully written data to the file
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn