获取 Java 中的对象类型
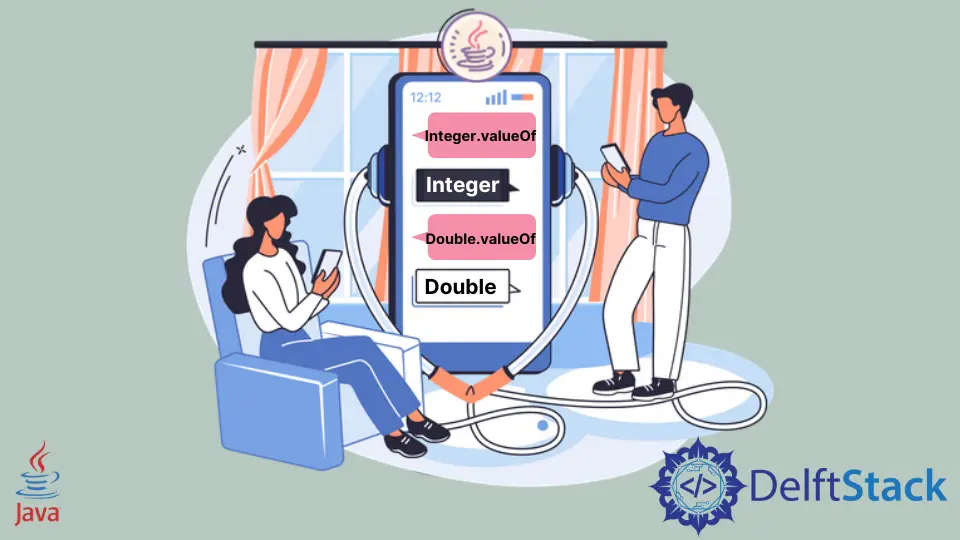
在本文中,我们将学习如何在 Java 中获取对象的类型。当对象来自源时,检查对象类型会很有帮助。这是我们无法验证对象类型的地方,例如来自我们无权访问的 API 或私有类。
在 Java 中使用 getClass()
获取对象类型
在第一种方法中,我们检查包装类的 Object 类型,如 Integer
和 String
。我们有两个对象,var1
和 var2
,用于检查类型。我们将使用 Object
类的 getClass()
方法,Java 中所有对象的父类。
我们使用 if
条件检查类。由于包装类还包含一个返回类型的字段类,我们可以检查谁的类型与 var1
和 var2
匹配。下面,我们检查三种类型的对象。
public class ObjectType {
public static void main(String[] args) {
Object var1 = Integer.valueOf("15");
Object var2 = String.valueOf(var1);
if (var1.getClass() == Integer.class) {
System.out.println("var1 is an Integer");
} else if (var1.getClass() == String.class) {
System.out.println("var1 is a String");
} else if (var1.getClass() == Double.class) {
System.out.println("var1 is a Double");
}
if (var2.getClass() == Integer.class) {
System.out.println("var2 is an Integer");
} else if (var2.getClass() == String.class) {
System.out.println("var2 is a String");
} else if (var2.getClass() == Double.class) {
System.out.println("var2 is a Double");
}
}
}
输出:
var1 is an Integer
var2 is a String
在 Java 中使用 instanceOf
获取对象类型
在 Java 中获取对象类型的另一种方法是使用 instanceOf
函数;如果对象的实例与给定的类匹配,则返回。在此示例中,我们使用以下三种类型检查对象 var1
和 var2
:Integer
、String
和 Double
;如果任何一个条件满足,我们就可以执行不同的代码。
因为 var1
是 Integer
类型,条件 var1 instanceOf Integer
将变为真,而 var2
是 Double
,因此,var2 instanceOf Double
将变为真。
public class ObjectType {
public static void main(String[] args) {
Object var1 = Integer.valueOf("15");
Object var2 = Double.valueOf("10");
if (var1 instanceof Integer) {
System.out.println("var1 is an Integer");
} else if (var1 instanceof String) {
System.out.println("var1 is a String");
} else if (var1 instanceof Double) {
System.out.println("var1 is a Double");
}
if (var2 instanceof Integer) {
System.out.println("var2 is an Integer");
} else if (var2 instanceof String) {
System.out.println("var2 is a String");
} else if (var2 instanceof Double) {
System.out.println("var2 is a Double");
}
}
}
输出:
var1 is an Integer
var2 is a Double
在 Java 中获取手动创建的类对象的类型
我们检查了包装类的类型,但是在这个例子中,我们得到了三个手动创建的类的三个对象的类型。我们创建了三个类:ObjectType2
、ObjectType3
和 ObjectType4
。
ObjectType3
继承了 ObjectType4
,ObjectType2
继承了 ObjectType3
。现在我们想知道所有这些类的对象类型。我们有三个对象,obj
、obj2
和 obj3
;我们使用了我们在上面的例子中讨论的 getClass()
和 instanceOf
两种方法。
但是,obj2
的类型之间存在差异。obj2
变量返回类型 ObjectType4
,而其类是 ObjectType3
。发生这种情况是因为我们继承了 ObjectType3
中的 ObjectType4
类,而 instanceOf
检查所有类和子类。obj
返回 ObjectType3
,因为 getClass()
函数只检查直接类。
public class ObjectType {
public static void main(String[] args) {
Object obj = new ObjectType2();
Object obj2 = new ObjectType3();
Object obj3 = new ObjectType4();
if (obj.getClass() == ObjectType4.class) {
System.out.println("obj is of type ObjectType4");
} else if (obj.getClass() == ObjectType3.class) {
System.out.println("obj is of type ObjectType3");
} else if (obj.getClass() == ObjectType2.class) {
System.out.println("obj is of type ObjectType2");
}
if (obj2 instanceof ObjectType4) {
System.out.println("obj2 is an instance of ObjectType4");
} else if (obj2 instanceof ObjectType3) {
System.out.println("obj2 is an instance of ObjectType3");
} else if (obj2 instanceof ObjectType2) {
System.out.println("obj2 is an instance of ObjectType2");
}
if (obj3 instanceof ObjectType4) {
System.out.println("obj3 is an instance of ObjectType4");
} else if (obj3 instanceof ObjectType3) {
System.out.println("obj3 is an instance of ObjectType3");
} else if (obj3 instanceof ObjectType2) {
System.out.println("obj3 is an instance of ObjectType2");
}
}
}
class ObjectType2 extends ObjectType3 {
int getAValue3() {
System.out.println(getAValue2());
a = 300;
return a;
}
}
class ObjectType3 extends ObjectType4 {
int getAValue2() {
System.out.println(getAValue1());
a = 200;
return a;
}
}
class ObjectType4 {
int a = 50;
int getAValue1() {
a = 100;
return a;
}
}
输出:
obj is of type ObjectType2
obj2 is an instance of ObjectType4
obj3 is an instance of ObjectType4
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn