在 Java 中实现数据访问对象
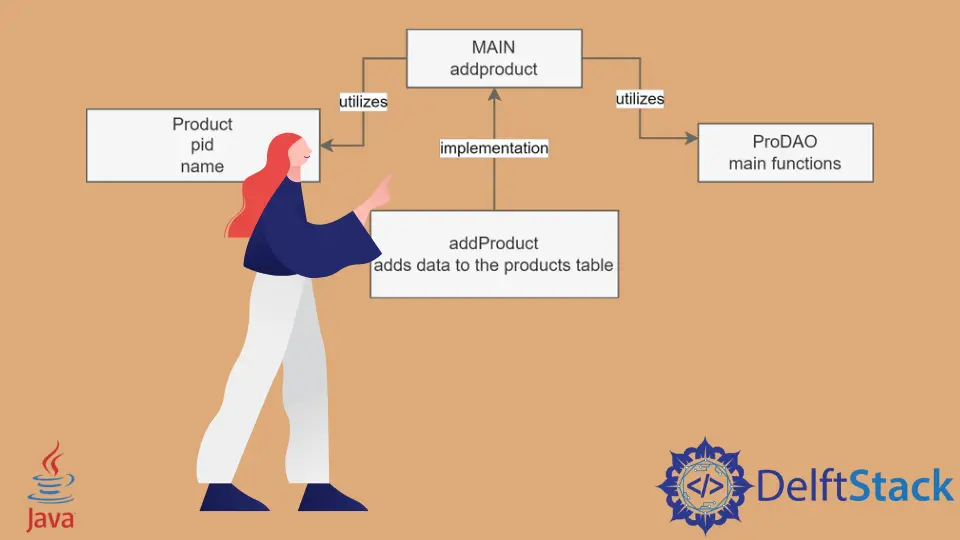
我们将创建一个新的数据库 mydb
和 products
表来展示使用 Java 中的 DAO 实时实现数据插入。
我们的 DAO 模型能够动态地使用 CRUD 应用程序。它使用 mysql-connector-java-8.0.22
的 JDBS 驱动
进行数据库连接。
它成功地抽象了对象的底层数据访问实现,以提供对数据的透明访问。
了解 Java 中的数据访问对象
我们将使用这种模式来实现我们与 DAO 的接口。这个模型是一个可以管理抽象数据源的自定义但功能齐全的 Java 数据访问对象的粗略表示。
假设你要更改数据库操作。你需要做的就是修改主类。
在这种情况下,我们的主类被定义为 JDBC 演示。
DAO 模式:
我们不会在一开始就让你感到困惑。因此,我们将尽可能简单地构建一个逻辑,而不是复制和粘贴,而是创建你的界面。
也就是说,这是我们的 product
类,它包含通过在主类中抛出的构造函数传递的产品值,该构造函数是本示例中为 addProduct
的函数对象的实例。
// Product class
class Product {
int id;
String name;
// add more values
}
然后,我们有一个接口,它使用数据访问对象模式来管理我们的数据库。让我们暂时保持它的重要性。
查看一个可以将产品添加到数据库表的简单函数。
public static void main(String[] args) {
// Now let us insert new product
// constructor PDAO class
// objects
ProDAO dao2 = new ProDAO(); // constructor
Product pro = new Product(); // constructor
// values to insert
// dynamically modify values from here later
pro.id = 3;
pro.name = "Oppo LOL";
// we have created a separate function for the db connection
dao2.Dbconnect();
// it is set to addProduct as of now, you can run CRUD directly from here
dao2.addProduct(pro);
}
这是我们将在实现代码部分执行的 DAO 的典型演示。
请注意,我们将在 MySQL 中创建一个数据库,然后我们将使用 MySQL 连接器 jar
文件与 SQL 服务器连接。
不用担心!因为我们还将向你展示如何使用 CLI 创建数据库。
如果你使用的是 Windows 操作系统:
但是,你可以使用诸如 MySQL Workbench
、SQL Yog
、phpMyAdmin
之类的 GUI 来创建你喜欢的数据库。
在 Java 中连接到 MySQL 服务器
保持透视并避免脏代码。我们将创建一个单独的数据库函数。
// Database Connection will use jdbc driver from the mysql connector jar
public void Dbconnect() {
try {
Class.forName("com.mysql.cj.jdbc.Driver"); // Mysql Connector's JDBC driver is loaded
// connection to mysql
con = DriverManager.getConnection(
"jdbc:mysql://localhost/mydb", "root", ""); // URL, database name after
// localhost, user name,
// password
} catch (Exception ex) {
System.out.println(ex);
}
}
你可以将此连接用于你的 MySQL 项目。不要忘记,如果你使用的是旧版本的 Java,请使用 Class.forName("com.mysql.jdbc.Driver");
用于加载 JDBC。
MySQL 数据库中数据访问对象的 Java 实现
首先,它有助于正确构建路径以避免运行时出现异常和警告。
通过右键单击你的 Java 项目、构建路径和配置 jar
文件,可以避免频繁出现的错误。只需确保你的构建路径如下图所示。
如果你的概念很清楚,你就会理解我们模型的以下 DAO 实现。尽管如此,我们已经为你评论了代码的每个元素。
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
// This Data Access Object DAO is a dynamic way to handle database records.
// main class
class DAOexample {
public static void main(String[] args) {
// Now let us insert new product
// constructor PDAO class
// objects
ProDAO dao2 = new ProDAO(); // constructor
Product pro = new Product(); // constructor
// values to insert
// dynamically modify values from here later
pro.id = 3;
pro.name = "Oppo LOL";
// we have created a separate function for the db connection
dao2.Dbconnect();
// it is set to addProduct as of now, you can run CRUD directly from here
dao2.addProduct(pro);
}
}
class ProDAO {
Connection con = null;
// Database Connection will use jdbc driver from the mysql connector jar
public void Dbconnect() {
try {
Class.forName("com.mysql.cj.jdbc.Driver");
// connection to mysql
con = DriverManager.getConnection(
"jdbc:mysql://localhost/mydb", "root", ""); // URL, database name after
// localhost, user name,
// password
} catch (Exception ex) {
System.out.println(ex);
}
}
// We will use the insert operation in this function, its conductor is already
// declared in the main class (DAO)
public void addProduct(Product p) { // this function will insert values
// insert query
// using prepared statements
String query2 = "insert into products values (?,?)";
try {
PreparedStatement pst;
pst = con.prepareStatement(query2);
pst.setInt(1, p.id);
pst.setString(2, p.name); //
pst.executeUpdate(); // executeUpdate is used for the insertion of the data
System.out.println("Inserted!");
} catch (Exception ex) {
}
}
}
// Product class
class Product {
int id;
String name;
// add more values
}
输出:
如果你仍有任何疑问,我们会提供此实现的完整 zip 文件夹,其中包含 jar
文件以及配置你的第一个 DAO 所需的一切。
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn