如何在 Go 中将字符串转换为整数类型
Suraj Joshi
2023年1月30日
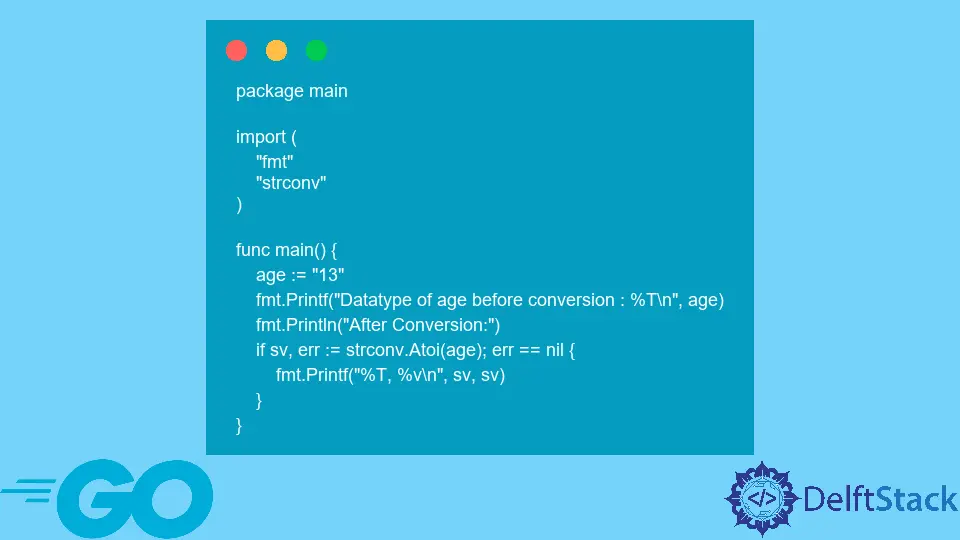
Go 是静态类型的语言,如果我们将变量定义为整型,则它只能为整型。我们不能在不转换变量的字符串数据类型的情况下为其分配字符串。Go 有一个名为 strconv
的内置包,它提供了三个函数 Atoi()
,Sscanf()
和 ParseInt()
将 Go 字符串转换为整型 int
。
来自 strconv
包的 Atoi()
函数
strconv
包实现了基本数据类型的字符串表示之间的转换。要将字符串转换为整数,我们使用来自 strconv
包的 Atoi()
函数。Atoi
代表 ASCII to integer
。
package main
import (
"fmt"
"strconv"
)
func main() {
age := "13"
fmt.Printf("Datatype of age before conversion : %T\n", age)
fmt.Println("After Conversion:")
if sv, err := strconv.Atoi(age); err == nil {
fmt.Printf("%T, %v\n", sv, sv)
}
}
输出:
Datatype of age before conversion : string
After Conversion:
int, 13
如果输入字符串不是整数格式,则该函数返回零。
package main
import (
"fmt"
"strconv"
)
func main() {
age := "abc"
fmt.Printf("Datatype of age before conversion : %T\n", age)
fmt.Println("After Conversion:")
sv, _ := strconv.Atoi(age)
fmt.Printf("%T, %v\n", sv, sv)
}
输出:
Datatype of age before conversion : string
After Conversion:
int, 0
来自 strconv
包的 ParseInt()
函数
strconv.ParseInt(s, base, bitSize)
方法以给定的 base (0,2 到 36)和位大小(0 到 64)解释输入字符串 s
并返回一个对应的整数。Atoi(s)
等同于 ParseInt(s, 10, 0)
。
package main
import (
"fmt"
"reflect"
"strconv"
)
func main() {
x := "123"
fmt.Println("Value of x before conversion: ", x)
fmt.Println("Datatype of x before conversion:", reflect.TypeOf(x))
intStr, _ := strconv.ParseInt(x, 10, 64)
fmt.Println("Value after conversion: ", intStr)
fmt.Println("Datatype after conversion:", reflect.TypeOf(intStr))
}
输出:
Value of x before conversion: 123
Datatype of x before conversion: string
Value after conversion: 123
Datatype after conversion: int64
fmt.Sscanf
方法
fmt.Sscanf()
非常适合解析包含数字的自定义字符串。Sscanf()
函数扫描参数字符串,将连续的以空格分隔的值存储到格式定义的连续参数中,并返回成功解析的项目数。
package main
import (
"fmt"
)
func main() {
s := "id:00234"
var i int
if _, err := fmt.Sscanf(s, "id:%5d", &i); err == nil {
fmt.Println(i)
}
}
输出:
234
方法比较
strconv.ParseInt()
是所有方法中最快的,strconv.Atoi()
比 strconv.ParseInt()
要慢一点,而 fmt.Sscanf()
是除最灵活方法之外最慢的方法。
作者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn