如何在 Go 中将整型 int 值转换为字符串
Suraj Joshi
2023年12月11日
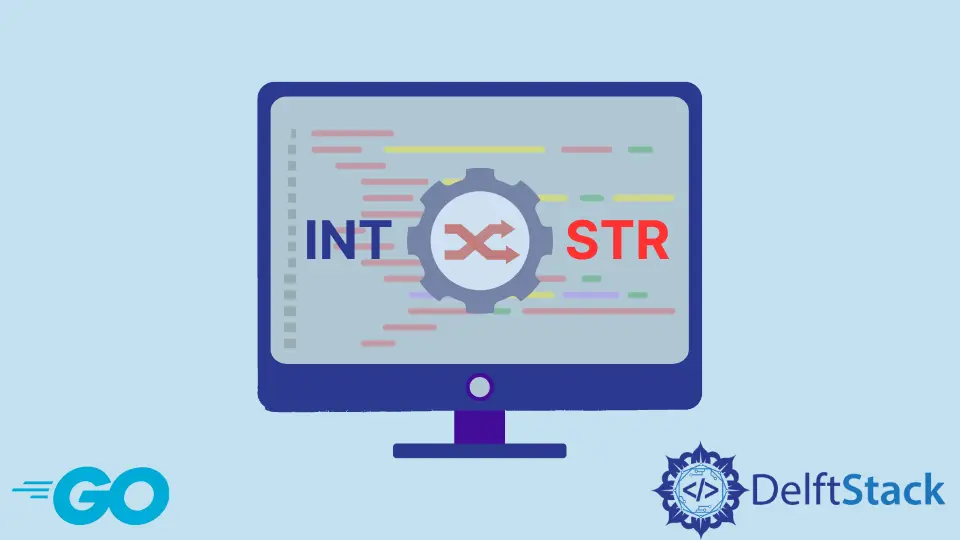
数据类型确定可以分配给该类型的值以及可以对其执行的操作。数据类型的转换是编程中广泛使用的操作,并且在数据类型转换中,从整型 int 到字符串值的转换非常流行。
在将数字打印到屏幕上或将其当作字符串使用时使用。Go 直接从标准库 strconv
提供的包中直接提供字符串和整数转换。
如果我们使用从 int
到字符串的简单转换,则整数值将被解释为 Unicode 代码点。结果字符串将包含代码点表示的字符,并以 UTF-8
编码。
package main
import "fmt"
func main() {
s := string(97)
fmt.Printf("Value of 97 after conversion : %v\n", s)
}
输出:
Value of 97 after conversion : a
但这是不可取的,因此我们使用一些标准函数将整数转换为字符串数据类型,下面将对此进行讨论:
strconv
包中的 Itoa
函数
strconv
包实现了基本数据类型的字符串表示之间的转换。要将整数转换为字符串,我们使用来自 strconv
包的 Itoa
函数。
package main
import (
"fmt"
"strconv"
)
func main() {
x := 138
fmt.Printf("Datatype of 138 before conversion : %T\n", x)
a := strconv.Itoa(x)
fmt.Printf("Datatype of 138 after conversion : %T\n", a)
fmt.Println("Number: " + a)
}
输出:
Datatype of 138 before conversion : int
Datatype of 138 after conversion : string
Number: 138
来自 strconv
包的 FormatInt
函数
我们使用 strconv.FormatInt
在给定的基础上格式化 int64。FormatInt
给出上述基数中整数的字符串表示形式,对于 2
<= base
<=36
,结果对数字值 >= 10
使用小写字母 a 至 z。
package main
import (
"fmt"
"strconv"
)
func main() {
var integer_1 int64 = 31
fmt.Printf("Value of integer_1 before conversion : %v\n", integer_1)
fmt.Printf("Datatype of integer_1 before conversion : %T\n", integer_1)
var string_1 string = strconv.FormatInt(integer_1, 10)
fmt.Printf("Value of integer_1 after conversion in base 10: %v\n", string_1)
fmt.Printf("Datatype of integer_1 after conversion in base 10 : %T\n", string_1)
var string_2 string = strconv.FormatInt(integer_1, 16)
fmt.Printf("Value of integer_1 after conversion in base 16 : %v\n", string_2)
fmt.Printf("Datatype of integer_1 after conversion in base 16 : %T\n", string_2)
}
输出:
Value of integer_1 before conversion : 31
Datatype of integer_1 before conversion : int64
Value of integer_1 after conversion in base 10: 31
Datatype of integer_1 after conversion in base 10 : string
Value of integer_1 after conversion in base 16 : 1f
Datatype of integer_1 after conversion in base 16 : string
Go fmt.Sprint
方法
当我们将一个整数传递给 fmt.Sprint
方法时,我们得到一个整数的字符串值。
package main
import (
"fmt"
)
func main() {
x := 138
fmt.Printf("Datatype of 138 before conversion : %T\n", x)
a := fmt.Sprint(x)
fmt.Printf("Datatype of 138 after conversion : %T\n", a)
fmt.Println("Number: " + a)
}
输出:
Datatype of 138 before conversion : int
Datatype of 138 after conversion : string
Number: 138
作者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn